How to use the Javascript Array Pop Method

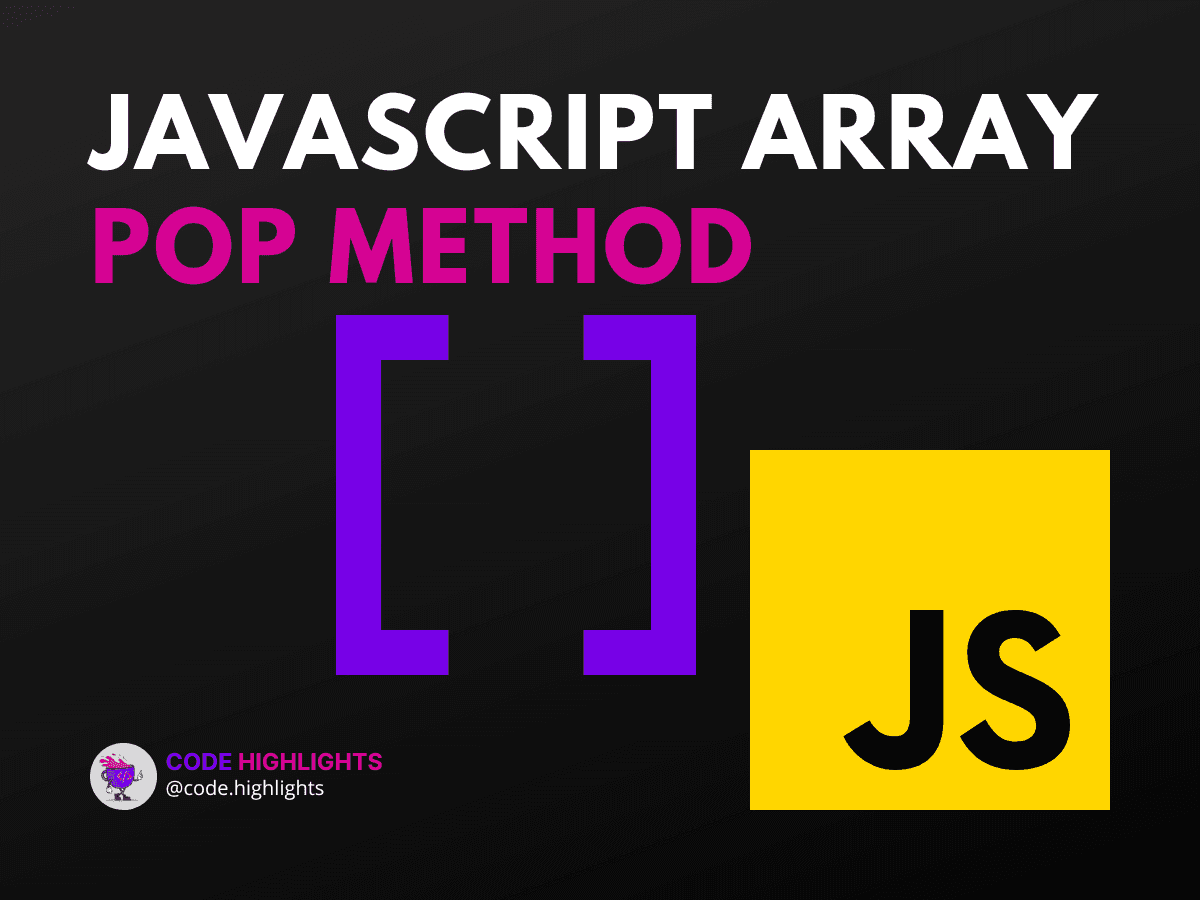
- How to Pop Value from Array in JavaScript
- What Does .pop() Do to an Array?
- How Do You Pop a Specific Value in an Array?
- How to Remove the Last Item from Array in JavaScript?
Are you looking to manipulate arrays in JavaScript efficiently? Understanding the JavaScript array pop method is essential for any budding developer. This method allows you to remove the last element from an array, which can be quite handy in various programming scenarios. Let's dive into how this method works and explore its practical applications.
In the example above, we can see the basic syntax of the .pop()
method in action—simple yet powerful. Now, let's delve deeper into its functionality and answer some common questions you might have.
How to Pop Value from Array in JavaScript
The .pop()
method is straightforward to use. It removes the last element of an array and returns that element. This method changes the length of the array.
1let numbers = [1, 2, 3, 4, 5];
2console.log(numbers.pop()); // Output: 5
3console.log(numbers); // Output: [1, 2, 3, 4]
What Does .pop()
Do to an Array?
When you invoke .pop()
, it not only returns the last element but also alters the original array by removing that element. If the array is empty, it returns undefined
.
How Do You Pop a Specific Value in an Array?
The .pop()
method only removes the last item of an array. To remove a specific value, you would have to find its index first and then use the .splice()
method. Here's how:
1let colors = ['Red', 'Green', 'Blue', 'Yellow'];
2let indexToRemove = colors.indexOf('Green');
3if (indexToRemove !== -1) {
4 colors.splice(indexToRemove, 1);
5}
6console.log(colors); // Output: ['Red', 'Blue', 'Yellow']
How to Remove the Last Item from Array in JavaScript?
Removing the last item is as simple as calling the .pop()
method on your array. It's a no-arguments method that does the job seamlessly.
1let tools = ['Hammer', 'Screwdriver', 'Wrench'];
2tools.pop();
3console.log(tools); // Output: ['Hammer', 'Screwdriver']
For those of you who are eager to expand your JavaScript knowledge, consider checking out this JavaScript course. And if you're just starting with web development, understanding HTML and CSS is equally important. Take a look at this HTML fundamentals course and this introductory CSS course. These resources will solidify your foundation and prepare you for more advanced topics like the JavaScript array pop method.
To further enhance your understanding, here are some reputable external sources:
- Mozilla Developer Network's Array.pop()
- W3Schools JavaScript Array pop() Method
- GeeksforGeeks explanation of the pop() method
Understanding the JavaScript array pop method is crucial for manipulating array elements. Remember, practice makes perfect. Try using .pop()
in different scenarios to see how it can help streamline your code. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
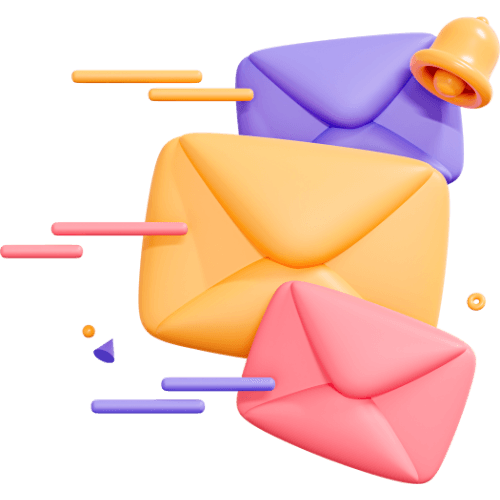
Related articles
114 Articles
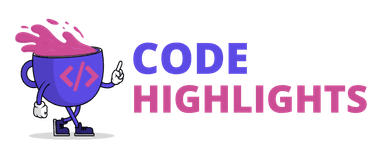
Copyright © Code Highlights 2024.