JavaScript Array shift() Method

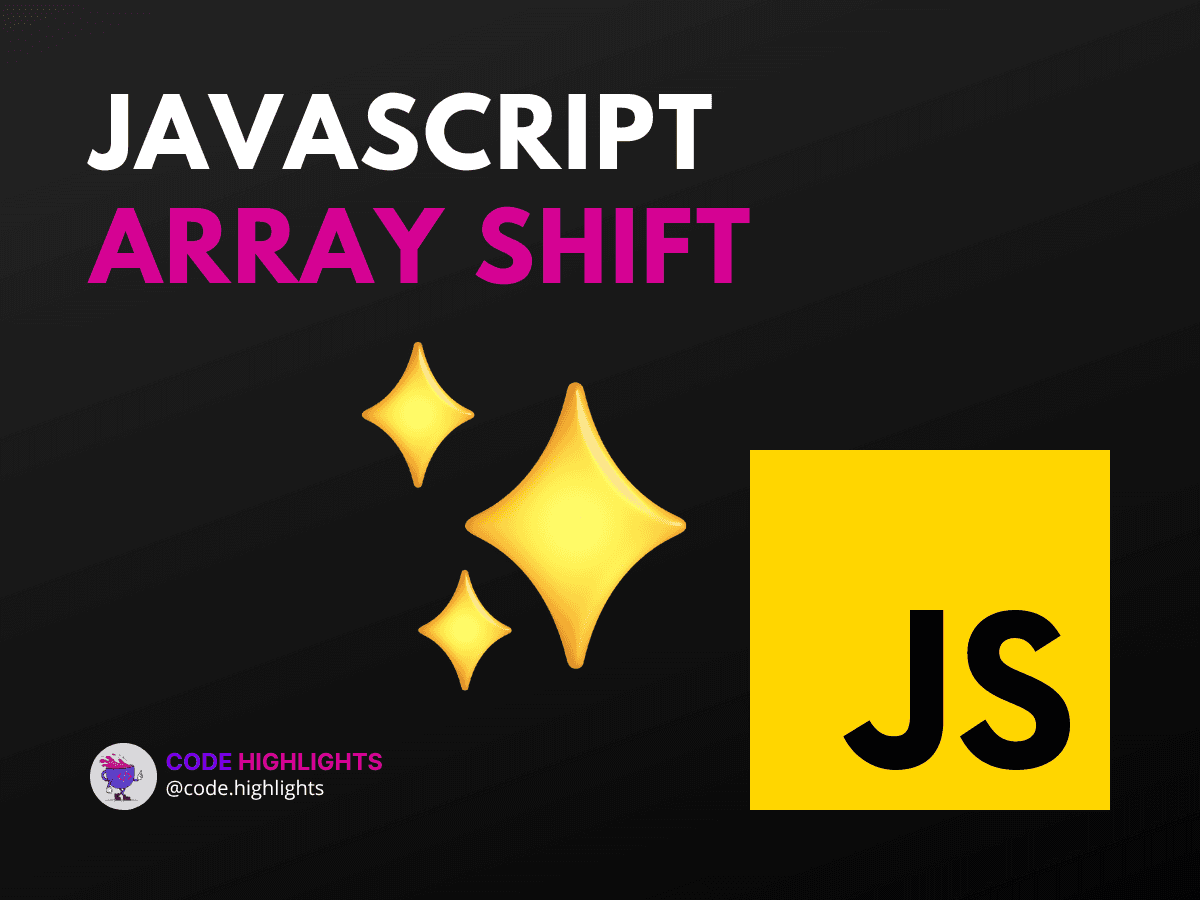
- Understanding shift() in Detail
- Practical Examples
- When Not to Use shift()
- Further Learning
- Conclusion
JavaScript is a powerful language that breathes life into web pages, turning them from static documents into dynamic, interactive experiences. One of the fundamental building blocks in JavaScript is the array, and understanding how to manipulate these arrays is crucial for any developer. Today, we're diving into one of the essential array methods: shift()
. This method is simple but incredibly useful when you need to remove the first element from an array. Let's explore how the javascript array shift
method works and see it in action with some code examples.
1let fruits = ["Apple", "Banana", "Cherry"];
2let firstFruit = fruits.shift();
3console.log(firstFruit); // Output: Apple
4console.log(fruits); // Output: ["Banana", "Cherry"]
In the snippet above, we see the shift()
method at work. It removed "Apple" from the fruits
array and returned it, leaving the rest of the array intact but shorter by one element.
Understanding shift()
in Detail
The javascript array shift
method is a straightforward tool with a specific purpose: to remove the first element from the beginning of an array. When you call shift()
on an array, it does two things:
- It removes the first element from the array.
- It returns the removed element.
This can be particularly handy when you're dealing with a queue structure where you want to process items in the order they were added (First In, First Out).
Syntax:
Return Value:
The value of the first element of the array that was removed. If the array is empty, it returns undefined
.
Practical Examples
Let's put the javascript array shift
method to use with some practical examples.
Imagine you're managing a to-do list, and you want to start by tackling the first task:
1let toDoList = ["Pay bills", "Meet with team", "Update website"];
2let firstTask = toDoList.shift();
3console.log(`Starting my day with: ${firstTask}`);
4// Output: Starting my day with: Pay bills
5console.log(`Remaining tasks: ${toDoList}`);
6// Output: Remaining tasks: Meet with team,Update website
In this example, we've successfully removed the first task from our toDoList
array and are ready to take it on.
When Not to Use shift()
While javascript array shift
is handy, it's not always the best choice. For instance, if you need to maintain the original array, shift()
is not what you want since it alters the array. In such cases, you might want to use methods like slice()
that do not modify the array.
Further Learning
For those looking to deepen their understanding of JavaScript and its array methods, consider checking out these resources:
Conclusion
The javascript array shift
method is a simple yet powerful tool that you'll find yourself using frequently when working with arrays. It's part of the array manipulation toolkit that every JavaScript developer should know. Remember, practice is key to mastering JavaScript, so try using shift()
in your next project.
For more information on JavaScript arrays and the shift()
method, check out these external resources:
- Mozilla Developer Network - Array.prototype.shift()
- W3Schools JavaScript Array shift() Method
- JavaScript Tutorial - Array Shift
Now that you have a solid understanding of the shift()
method, you're one step closer to becoming a JavaScript array wizard! Keep experimenting, keep learning, and most importantly, keep coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
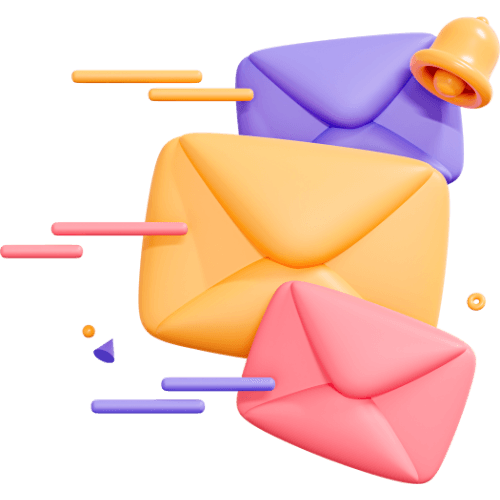
Related articles
9 Articles
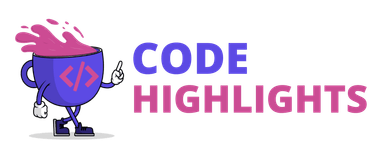
Copyright © Code Highlights 2025.