Master JavaScript Array Slice() Method in 3 Easy Steps

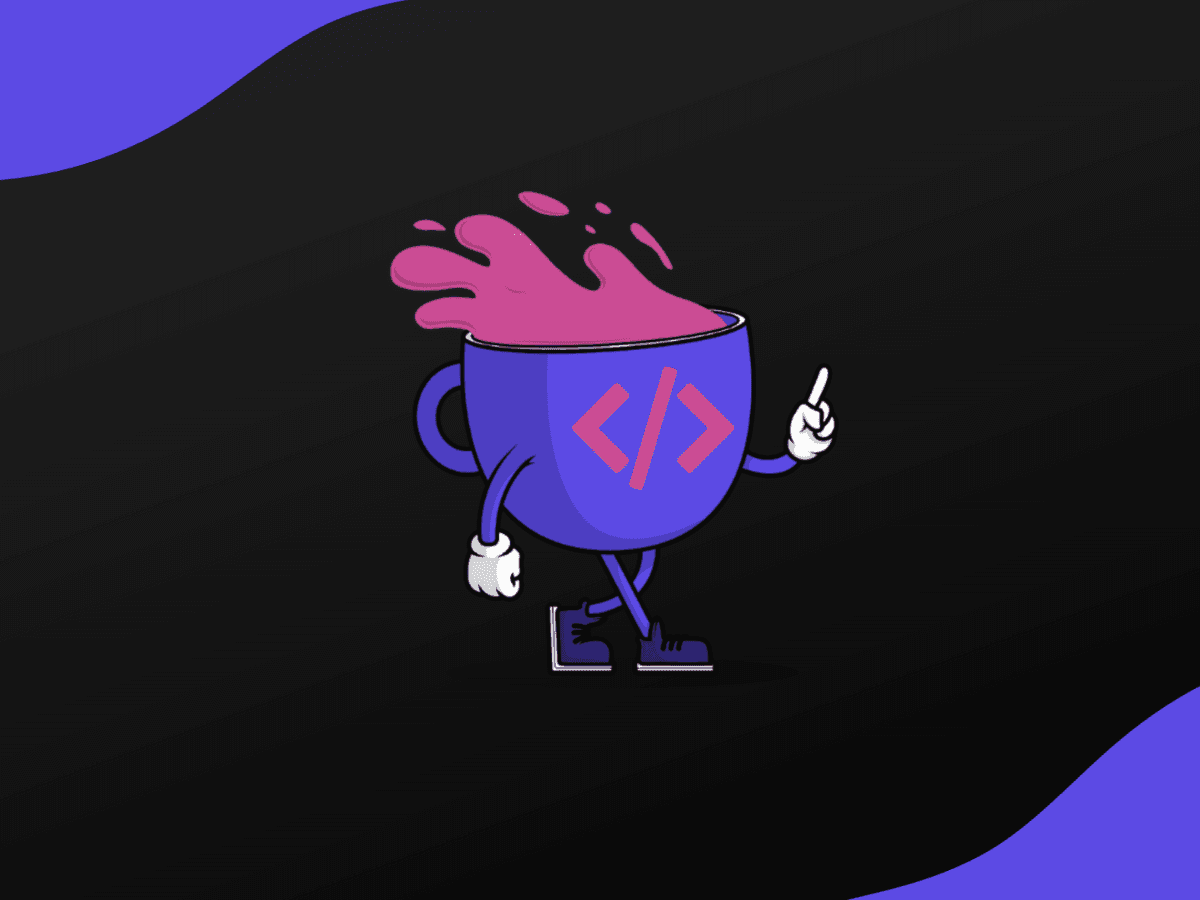
- Understanding Array.prototype.slice()
- Using Negative Indexes with slice()
- Omitting the end Parameter
- Browser Compatibility
In JavaScript, arrays are a crucial data type that developers use quite often. They store multiple values within a single variable, which can be manipulated in many ways. One of the most useful methods is the Array.prototype.slice()
method.
1let fruits = ["apple", "banana", "mango", "grape", "pineapple"];
2let citrusFruits = fruits.slice(1, 3);
3console.log(citrusFruits); // Outputs: ["banana", "mango"]
The slice()
method creates a new array from a portion of an existing array. Let's explore how this works.
Understanding Array.prototype.slice()
The Array.prototype.slice()
method returns a shallow copy of a portion of an array into a new array object. It takes two parameters: start
and end
(optional). The method doesn't modify the original array but instead returns a new one.
1let numbers = [1, 2, 3, 4, 5];
2let subset = numbers.slice(2, 4);
3console.log(subset); // Outputs: [3, 4]
Using Negative Indexes with slice()
The slice()
method also accepts negative indexes. A negative index indicates an offset from the end of the array. For instance, -1
refers to the last element, -2
to the second last, and so on.
1let colors = ["red", "green", "blue", "yellow", "purple"];
2let primaryColors = colors.slice(-3, -1);
3console.log(primaryColors); // Outputs: ["blue", "yellow"]
Omitting the end
Parameter
If the end
parameter is omitted, slice()
will extract till the end of the array.
1let animals = ["cat", "dog", "elephant", "giraffe", "lion"];
2let zoo = animals.slice(2);
3console.log(zoo); // Outputs: ["elephant", "giraffe", "lion"]
Browser Compatibility
The Array.prototype.slice()
method is supported in all modern browsers, and IE6 and up.
If you found this tutorial useful, you might also like our Javascript Fundamentals Course, which features a ton of useful methods like these.
For further reading on JavaScript arrays, I recommend articles from MDN Web Docs and JavaScript Info.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
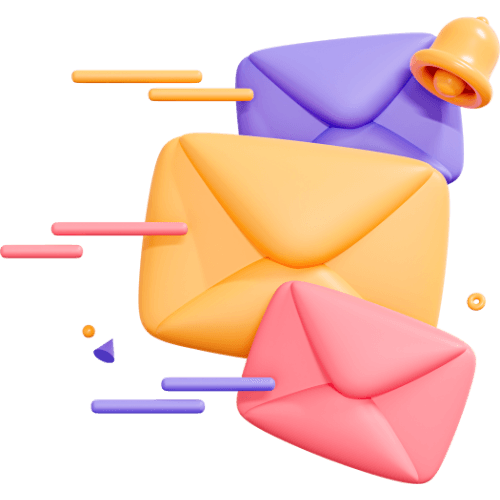
Related articles
9 Articles
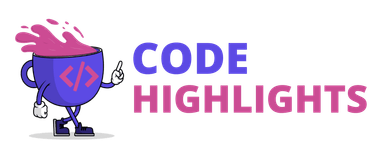
Copyright © Code Highlights 2024.