JavaScript Array Sort – How to Use the Sort Function

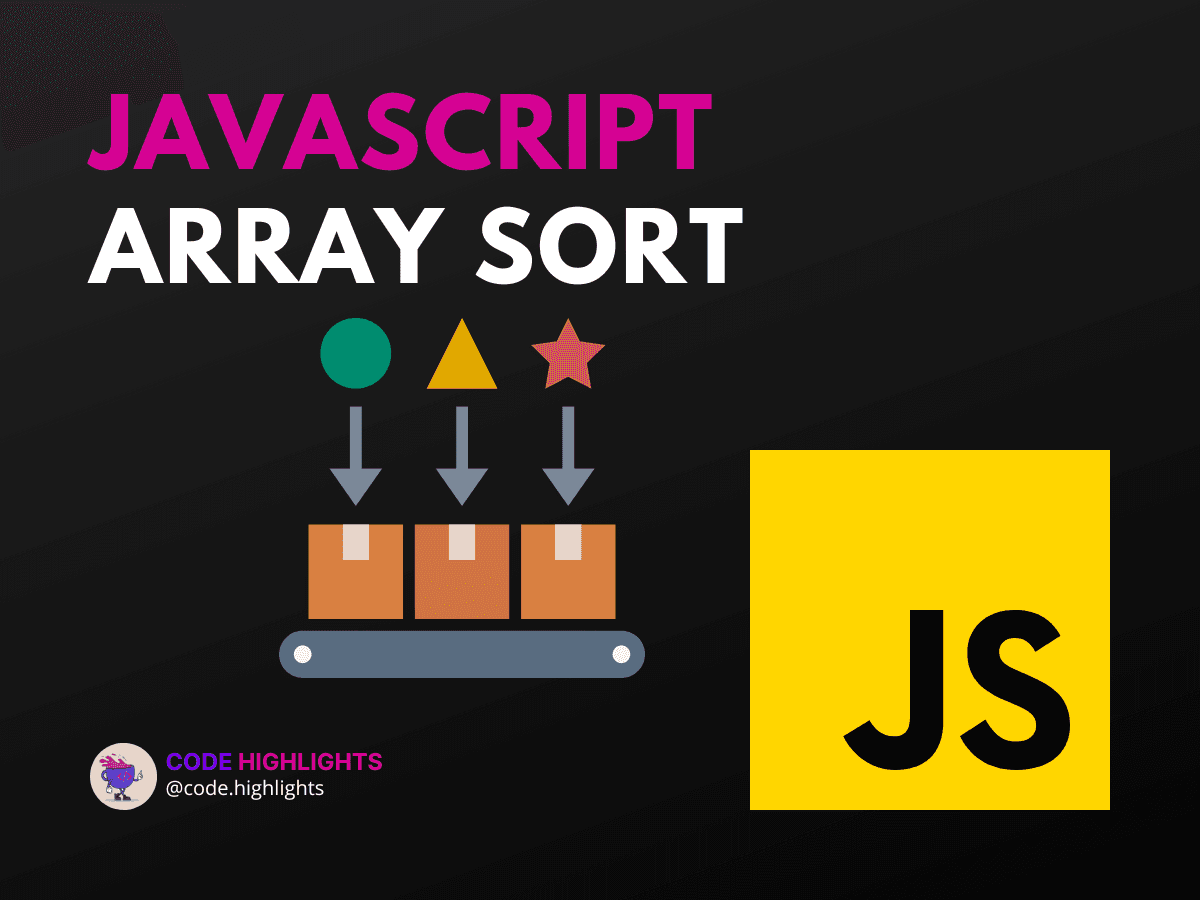
- Quick Example of JavaScript Array Sort
- Understanding the Sort Method
- Custom Sorting with Comparison Functions
- Advanced Sorting Techniques
- Best Practices and Performance
- Conclusion and Further Learning
Sorting arrays is an essential operation in programming, and JavaScript provides a versatile sort()
method for this purpose. Whether you're a beginner or an experienced developer, mastering array sorting in JavaScript can greatly enhance your coding toolkit. In this tutorial, we'll dive deep into the javascript array sort
method, exploring its syntax and behavior with practical examples to solidify your understanding.
Quick Example of JavaScript Array Sort
Before we delve into the nitty-gritty, let's take a quick look at how sorting works in JavaScript:
1let fruits = ['banana', 'apple', 'orange'];
2fruits.sort();
3console.log(fruits); // Output: ['apple', 'banana', 'orange']
As you can see, the sort()
method arranges the elements of the array in ascending, lexicographical order by default. But there's much more to it, so let's get started!
Understanding the Sort Method
The sort()
method can be a bit tricky, as it sorts the array elements as strings by default. This can lead to unexpected results when sorting numbers:
To handle numeric sorting correctly, you need to provide a comparison function:
Custom Sorting with Comparison Functions
When sorting objects or complex data, you'll need to define how the elements are compared. Here's an example of sorting an array of objects by a property:
1let items = [
2 { name: 'Table', price: 45 },
3 { name: 'Chair', price: 20 },
4 { name: 'Lamp', price: 10 }
5];
6
7items.sort(function(a, b) {
8 return a.price - b.price;
9});
10
11console.log(items); // Output: sorted by price in ascending order
Advanced Sorting Techniques
For more advanced sorting, such as sorting in descending order or by multiple criteria, you can adjust your comparison function accordingly:
1// Descending order
2numbers.sort(function(a, b) {
3 return b - a;
4});
5
6// Multiple criteria
7items.sort(function(a, b) {
8 let diff = a.price - b.price;
9 if (diff === 0) {
10 return a.name.localeCompare(b.name);
11 }
12 return diff;
13});
Best Practices and Performance
When using javascript array sort
, consider the performance implications for large datasets. Sorting is generally an expensive operation, so optimizing your comparison function and considering algorithms like quicksort or mergesort for custom implementations is wise.
Conclusion and Further Learning
This guide has provided you with a comprehensive understanding of the javascript array sort
method. To continue learning and enhancing your JavaScript skills, consider checking out these resources:
For additional information on sorting algorithms and their complexities, refer to external resources from Mozilla Developer Network (MDN), W3Schools, and JavaScript.info.
By applying the knowledge from this tutorial and leveraging these resources, you'll be well-equipped to tackle array sorting challenges in your future projects. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
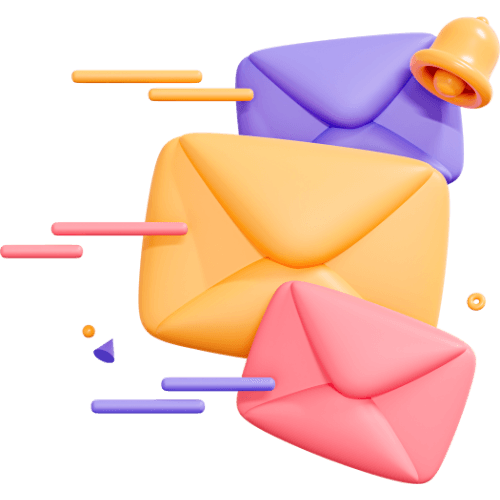
Related articles
9 Articles
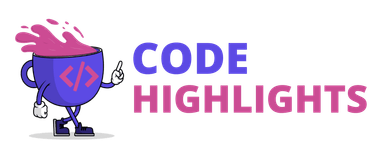
Copyright © Code Highlights 2025.