JavaScript Splice: How to Modify Arrays Like a Pro

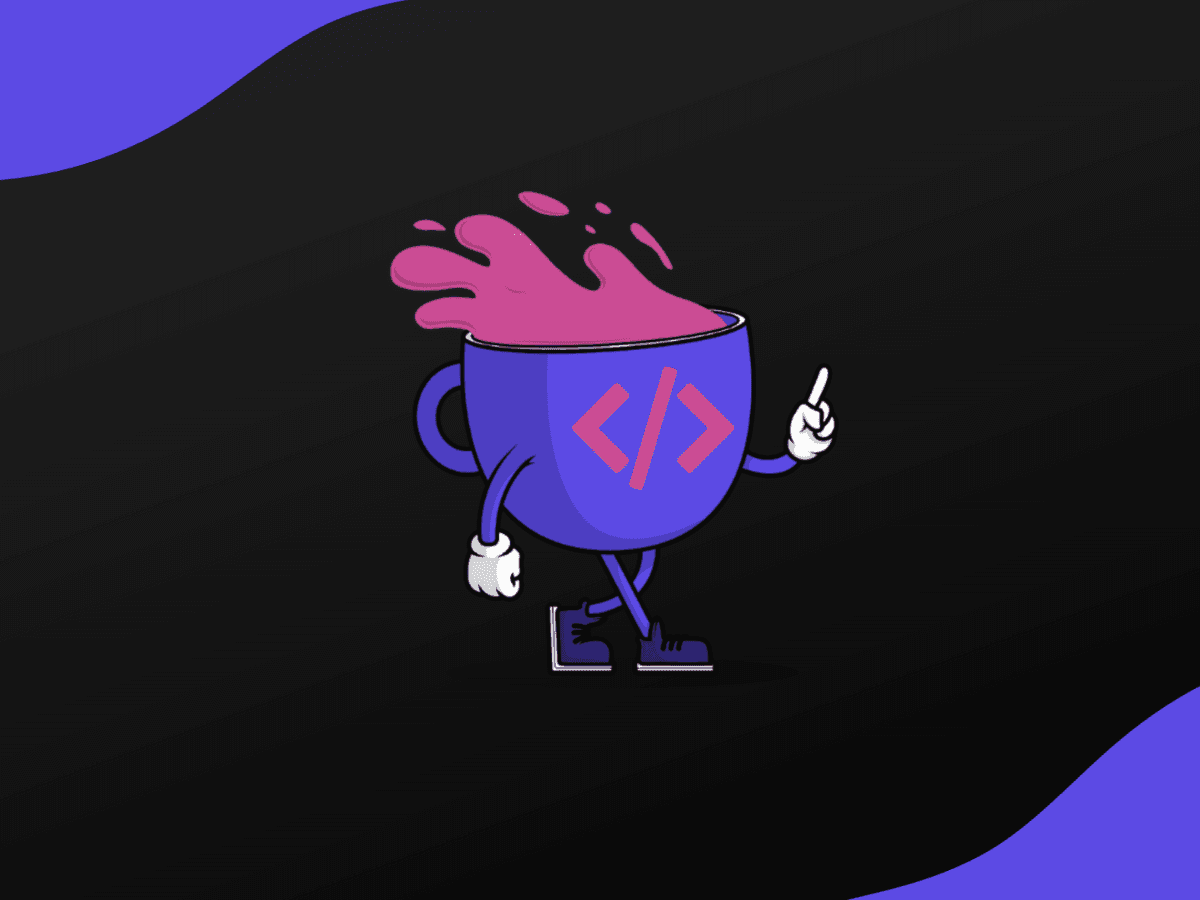
- Understanding splice()
- Deleting Elements with splice()
- Inserting Elements with splice()
- Replacing Elements with splice()
- Browser Compatibility
In JavaScript, arrays are a versatile data structure with various methods for manipulation. One such method is splice()
. This tutorial will explore how to use the splice()
method to delete, insert, and replace elements in an array.
Understanding splice()
The splice()
method changes the content of an array by removing or replacing existing elements and/or adding new elements in place. Here's its basic syntax:
The index
parameter specifies at what position to add/remove items. The deleteCount
parameter indicates the number of items to be removed. The item1, ..., itemX
parameters are the new items to be added.
Deleting Elements with splice()
To remove elements from an array, you can use splice()
by providing the index position and the number of elements to delete.
1var fruits = ["Banana", "Orange", "Apple", "Mango"];
2fruits.splice(2, 2); // Returns ["Apple", "Mango"]
In this example, starting from the third element (index 2), two elements - "Apple" and "Mango" - are removed.
Inserting Elements with splice()
splice()
can also insert elements without removing any. Just set the deleteCount
parameter to 0
.
1var fruits = ["Banana", "Orange", "Apple", "Mango"];
2fruits.splice(2, 0, "Lemon", "Kiwi"); // Returns ["Banana", "Orange", "Lemon", "Kiwi", "Apple", "Mango"]
Here, "Lemon" and "Kiwi" are inserted at the third position, and no elements are removed.
Replacing Elements with splice()
Lastly, splice()
can replace elements by removing them and adding new ones at the same position.
1var fruits = ["Banana", "Orange", "Apple", "Mango"];
2fruits.splice(2, 1, "Lemon"); // Returns ["Banana", "Orange", "Lemon", "Mango"]
In this case, "Apple" is replaced with "Lemon".
Browser Compatibility
The splice()
method works in all modern browsers, as well as Internet Explorer 6 and up. For more JavaScript learning resources, check out our JavaScript course.
To answer the question "Was macht Splice?", splice()
is a versatile JavaScript array method that can delete, insert, and replace elements in an array.
For more in-depth information on JavaScript arrays and the splice()
method, you can visit Mozilla Developer Network and W3Schools.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
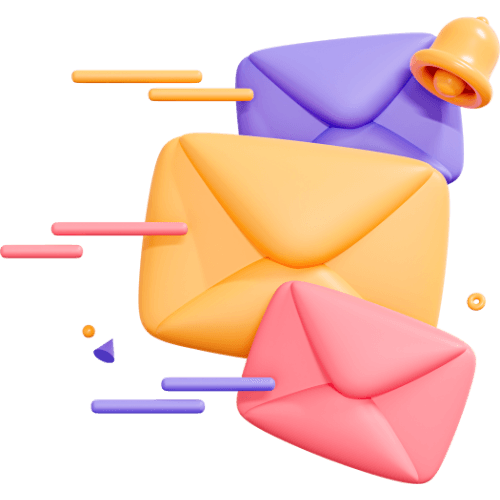
Related articles
9 Articles
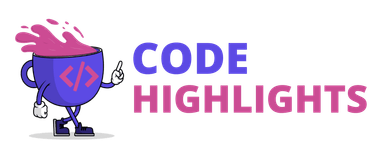
Copyright © Code Highlights 2025.