How to Use JavaScript Average Function for Effortless Calculations

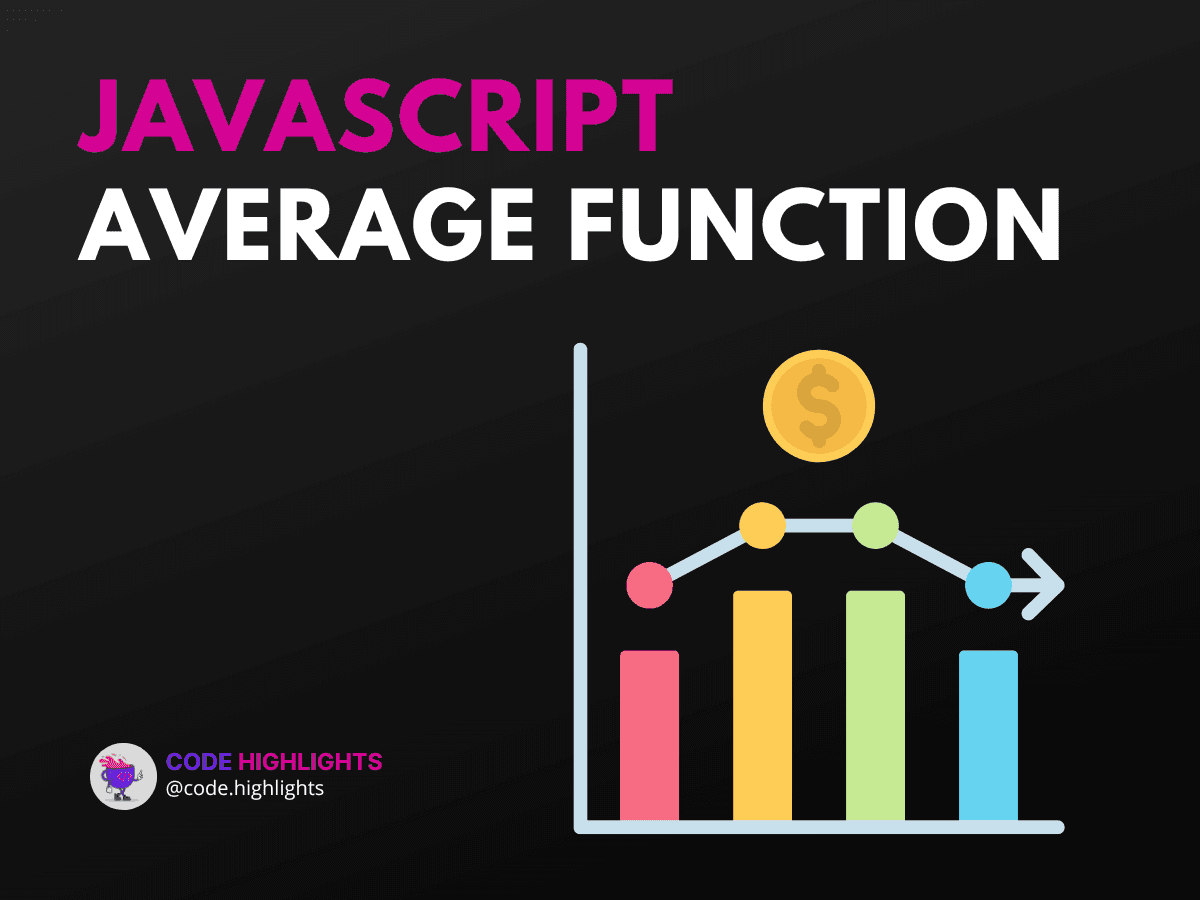
- Quick Example
- Understanding the Syntax
- Parameters
- Return Value
- Variations
- Example 1: Basic Average Calculation
- Title: Basic Average of Numbers
- Example 2: Average with Non-Numeric Values
- Title: Handling Non-Numeric Values
- Example 3: Average of Three Numbers
- Title: Finding the Average of Three Numbers
- Example 4: Average in a Web Application
- Title: Integrating Average Function in a Form
- Browser Compatibility
- Key Points Summary
- Answers to Common Questions
Calculating the average of numbers is a common task in programming. The JavaScript average function can help simplify this process. Whether you are working on a school project, building a website, or developing a web application, knowing how to find the average can save you time and effort. In this tutorial, we will explore how to create a simple average function in JavaScript and see various examples to illustrate its use.
Quick Example
Here's a quick look at how you can define an average function in JavaScript:
1function average(numbers) {
2 const sum = numbers.reduce((acc, num) => acc + num, 0);
3 return sum / numbers.length;
4}
5
6console.log(average([10, 20, 30])); // Output: 20
In this example, the function takes an array of numbers, calculates the sum, and then divides it by the number of elements to find the average.
Understanding the Syntax
The syntax for creating an average function can vary, but here’s a basic outline:
Parameters
- array: This parameter accepts an array of numbers.
Return Value
- The function returns the average value calculated from the input array.
Variations
You can customize the average function to handle different scenarios. For instance, you might want to ignore non-numeric values or calculate the average of only a specific subset of numbers.
Example 1: Basic Average Calculation
Title: Basic Average of Numbers
1function average(numbers) {
2 const sum = numbers.reduce((acc, num) => acc + num, 0);
3 return sum / numbers.length;
4}
5
6console.log(average([5, 15, 25])); // Output: 15
This example shows a straightforward way to calculate the average of three numbers: 5, 15, and 25.
Example 2: Average with Non-Numeric Values
Title: Handling Non-Numeric Values
1function average(numbers) {
2 const filteredNumbers = numbers.filter(num => typeof num === 'number');
3 const sum = filteredNumbers.reduce((acc, num) => acc + num, 0);
4 return sum / filteredNumbers.length;
5}
6
7console.log(average([10, 'a', 20, null, 30])); // Output: 20
In this case, the function filters out non-numeric values before calculating the average.
Example 3: Average of Three Numbers
Title: Finding the Average of Three Numbers
1function average(a, b, c) {
2 return (a + b + c) / 3;
3}
4
5console.log(average(10, 20, 30)); // Output: 20
This example demonstrates how to find the average of three specific numbers. It’s a simpler approach when you know the exact values you want to average.
Example 4: Average in a Web Application
Title: Integrating Average Function in a Form
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>Average Calculator</title>
6</head>
7<body>
8 <input type="number" id="num1" placeholder="Enter first number">
9 <input type="number" id="num2" placeholder="Enter second number">
10 <input type="number" id="num3" placeholder="Enter third number">
11 <button onclick="calculateAverage()">Calculate Average</button>
12 <p id="result"></p>
13
14 <script>
15 function calculateAverage() {
16 const num1 = parseFloat(document.getElementById('num1').value);
17 const num2 = parseFloat(document.getElementById('num2').value);
18 const num3 = parseFloat(document.getElementById('num3').value);
19 const avg = average(num1, num2, num3);
20 document.getElementById('result').innerText = `Average: ${avg}`;
21 }
22
23 function average(a, b, c) {
24 return (a + b + c) / 3;
25 }
26 </script>
27</body>
28</html>
In this example, we create a simple HTML form that allows users to input three numbers and calculate their average when they click a button.
Browser Compatibility
The JavaScript average function works well across all major web browsers like Chrome, Firefox, Safari, and Edge. You can use it confidently in your projects without worrying about compatibility issues.
Key Points Summary
In this tutorial, we learned about the JavaScript average function. We covered how to create a basic function, handle non-numeric values, and even integrate it into a web application. Understanding how to calculate averages can be beneficial for various projects, from simple calculations to more complex applications. If you want to dive deeper into JavaScript, consider checking out our JavaScript course.
For those looking to enhance their web development skills, our course on HTML Fundamentals and CSS Introduction can provide valuable insights. Additionally, if you're interested in a broader understanding of web development, take a look at our Introduction to Web Development.
Answers to Common Questions
-
Does JavaScript have an average function? No, JavaScript does not have a built-in average function. However, you can easily create one using arrays.
-
How to find the average of inputs in JavaScript? You can use the
reduce
method on an array to calculate the sum and then divide by the number of elements. -
How to find the average of three numbers in JavaScript? You can create a simple function that takes three parameters and returns their average.
-
What is the difference between average() function and MIN() function? The
average()
function calculates the mean of a set of numbers, while theMIN()
function finds the smallest number in a set.
By mastering the JavaScript average function, you can make your programming tasks easier and more efficient!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
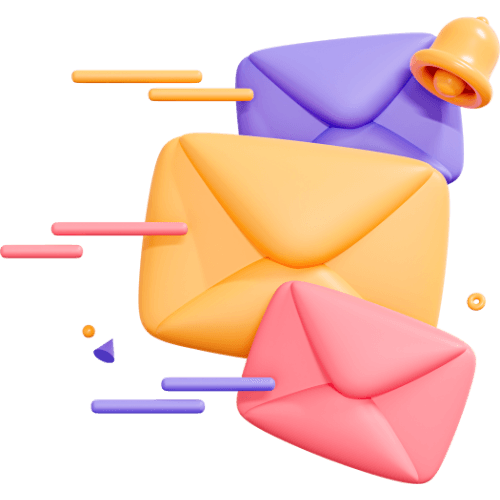
Related articles
9 Articles
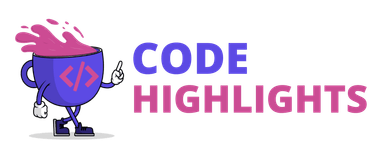
Copyright © Code Highlights 2025.