5 Proven Strategies to Master JavaScript Bang (!) for Coders

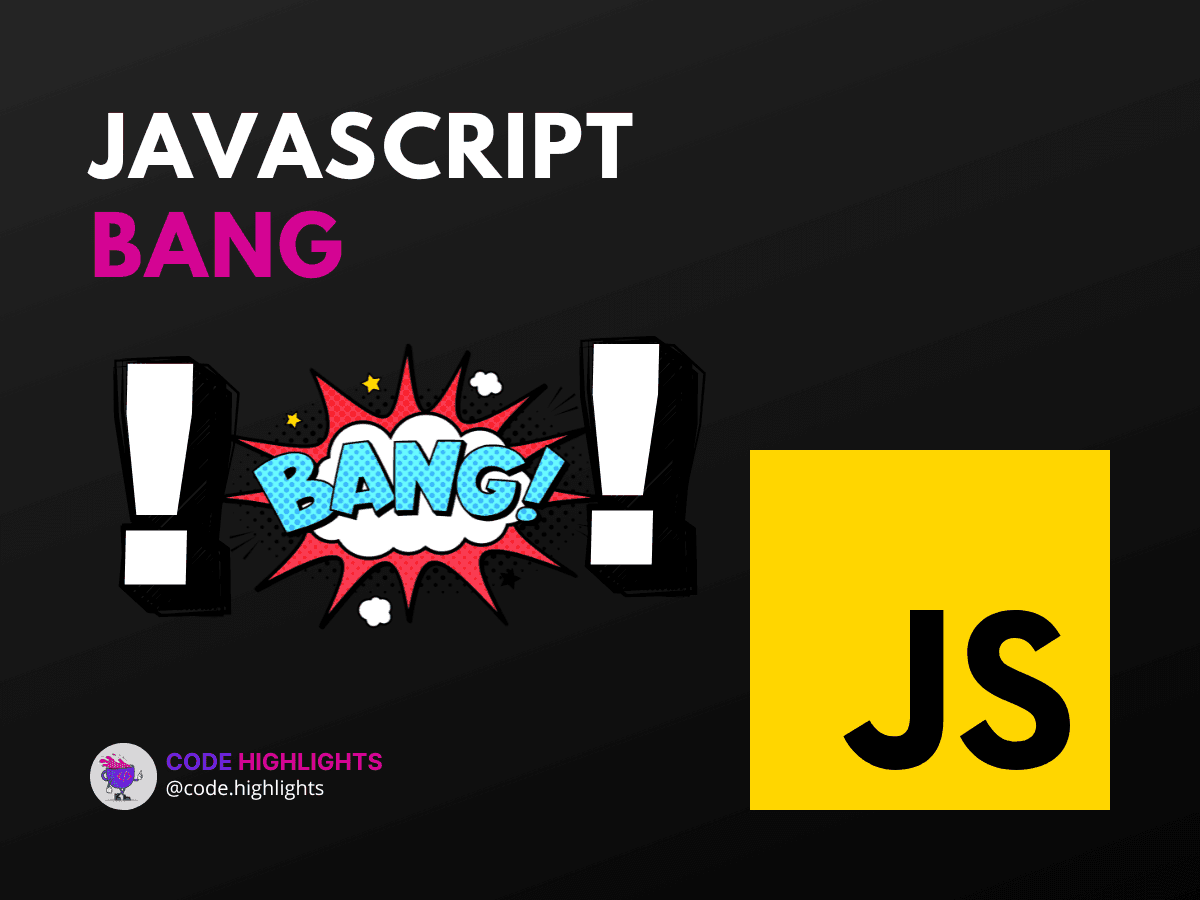
- Strategy 1: Using Bang for Boolean Conversion
- Strategy 2: Double Bang for Explicit Boolean Casting
- Strategy 3: Bang with Nullish and Undefined Values
- Strategy 4: Short-Circuit Evaluation with Bang
- Strategy 5: Bang in Ternary Operations
- Wrapping Up
JavaScript is a language full of nuances and tricks that can make our code cleaner and more efficient. One such trick is the use of the JavaScript bang (!
), also known as the logical NOT operator. It's a powerful tool in a coder's arsenal when used correctly. In this tutorial, we'll explore five proven strategies to master the JavaScript bang, ensuring you write more concise and readable code.
Before we dive into the strategies, let's look at a simple example of the JavaScript bang in action:
This code snippet demonstrates how the bang operator can invert a boolean value. But there's more to it than just flipping true
to false
and vice versa. Ready to become a JavaScript bang expert? Let's get started!
Strategy 1: Using Bang for Boolean Conversion
The bang operator is a quick way to convert a value to its boolean opposite. This is particularly useful in conditional statements:
1const userResponse = ''; // An empty string
2if (!userResponse) {
3 console.log('No response received.');
4}
In the above example, the empty string is falsy, and the bang operator converts it to true
, triggering the if
block.
Strategy 2: Double Bang for Explicit Boolean Casting
Sometimes, you need to explicitly check the truthiness of a value. That's where the double bang !!
comes in handy:
Here, the first bang converts the number to false
, and the second one flips it back, effectively casting the original value to a boolean.
Strategy 3: Bang with Nullish and Undefined Values
When dealing with variables that might be null
or undefined
, the bang operator can help you avoid errors:
This ensures that user
has a value before proceeding with your code.
Strategy 4: Short-Circuit Evaluation with Bang
Combine the bang with logical operators for short-circuit evaluation:
If isLoggedIn
is false
, the message prompts the user to log in.
Strategy 5: Bang in Ternary Operations
Ternary operations become more readable with the bang operator:
This succinctly sets access
based on the isAdmin
flag.
Wrapping Up
Mastering the JavaScript bang operator can greatly enhance your coding skills. For those eager to delve deeper into JavaScript, consider exploring our JavaScript course. And if you're new to coding, our Introduction to Web Development course is a great starting point.
Remember, practice makes perfect. So, experiment with these strategies in your own projects. Happy coding!
Further Reading
- MDN Web Docs on Logical NOT Operator
- JavaScript.info on JavaScript Type Conversions
- W3Schools JavaScript Tutorial
- ECMA International's ECMAScript Language Specification
Expand your front-end skills by learning more about HTML and CSS. They are the building blocks that work alongside JavaScript to create dynamic web experiences.
With these strategies and resources, you're well on your way to mastering the JavaScript bang operator and writing more effective code. Keep practicing, and don't forget to check out the recommended courses and readings to further your development journey!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
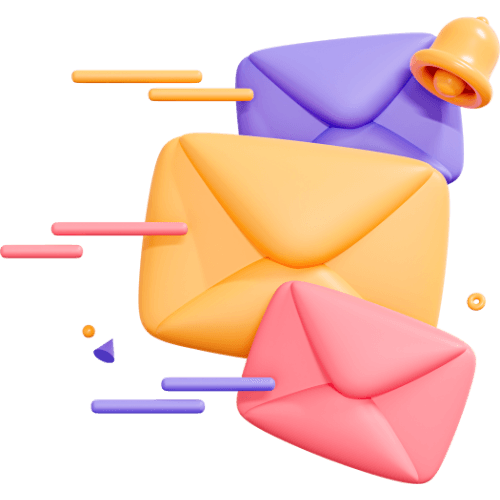
Related articles
9 Articles
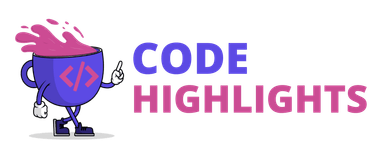
Copyright © Code Highlights 2025.