5 Essential JavaScript Between Methods to Master in 2024

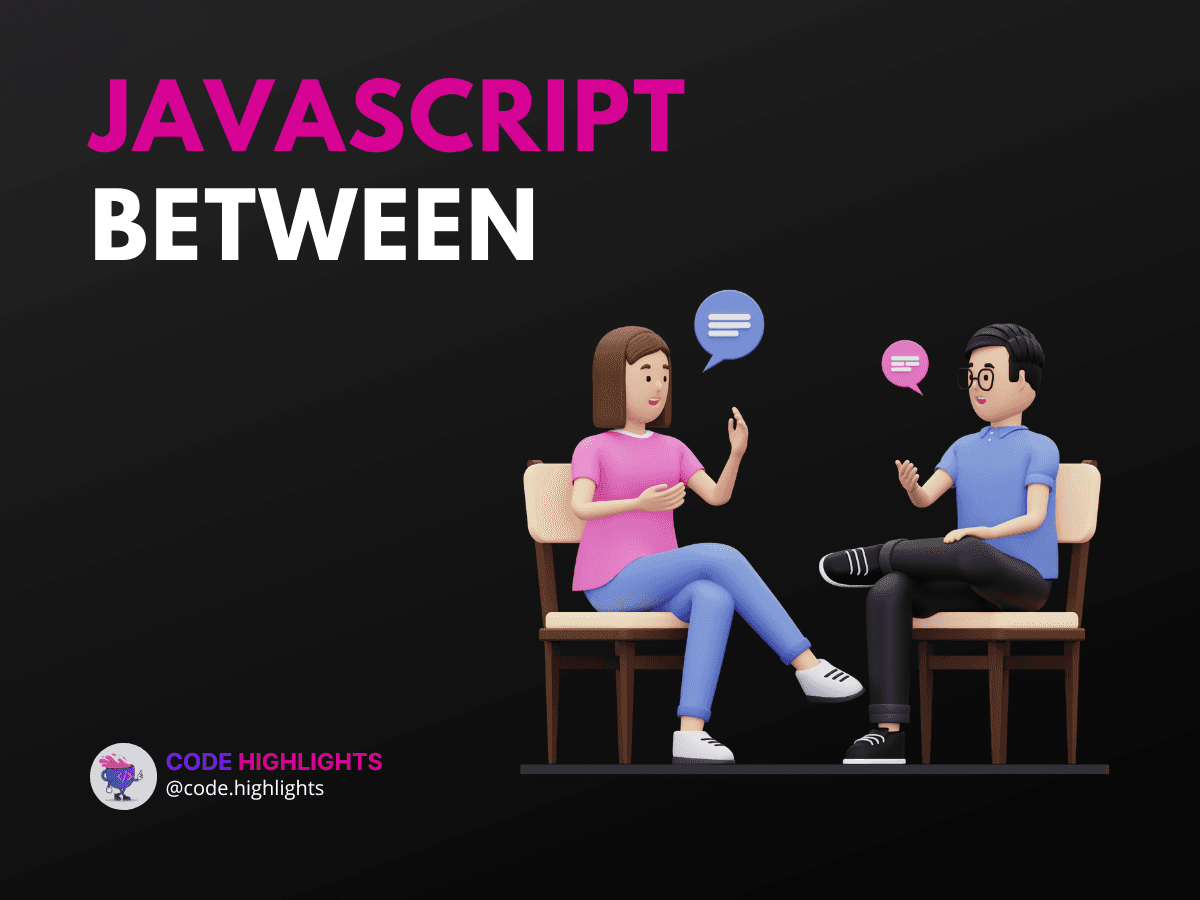
- Quick Code Example
- Understanding the Syntax
- Parameters:
- Return Value:
- Example 1: Checking Number Range
- Title: Check if a Number Falls Within a Range
- Example 2: Extracting Substrings
- Title: Get String Between Parentheses
- Example 3: Using Array Filter
- Title: Filter Array Values
- Example 4: Date Comparison
- Title: Check If a Date is Within a Range
- Example 5: Working with Objects
- Title: Filter Objects Based on Property Value
- Browser Compatibility
- Summary
JavaScript is a versatile programming language used for web development. One common task developers face is checking if a value lies between two numbers or extracting specific substrings. This tutorial covers five essential methods related to the concept of "between" in JavaScript. By mastering these techniques, you can enhance your coding skills and streamline your projects.
Quick Code Example
Here's a simple example that shows how to check if a number is between two values:
1function isBetween(num, min, max) {
2 return num >= min && num <= max;
3}
4
5console.log(isBetween(5, 1, 10)); // true
This function checks if num
is between min
and max
.
Understanding the Syntax
In JavaScript, there isn't a built-in "between" function. However, we can create our own using comparison operators. The basic syntax for our custom function looks like this:
Parameters:
value
: The number to check.lowerBound
: The minimum value.upperBound
: The maximum value.
Return Value:
- Returns
true
ifvalue
is betweenlowerBound
andupperBound
; otherwise, returnsfalse
.
Example 1: Checking Number Range
Title: Check if a Number Falls Within a Range
Let's look at a practical example where we check if a score falls within a passing range:
1function isPassing(score) {
2 return isBetween(score, 60, 100);
3}
4
5console.log(isPassing(75)); // true
6console.log(isPassing(55)); // false
In this case, we determine if a score is passing by checking if it's between 60 and 100.
Example 2: Extracting Substrings
Title: Get String Between Parentheses
You might need to extract text between parentheses in a string. Here's how you can do that:
1function getStringBetween(str) {
2 const start = str.indexOf('(') + 1;
3 const end = str.indexOf(')');
4 return str.substring(start, end);
5}
6
7console.log(getStringBetween("Hello (world)")); // world
This function finds the text between the first pair of parentheses.
Example 3: Using Array Filter
Title: Filter Array Values
You can also use a custom "between" function with arrays. For example, filtering numbers within a specific range:
1const numbers = [1, 5, 8, 12, 15, 20];
2
3const filteredNumbers = numbers.filter(num => isBetween(num, 5, 15));
4console.log(filteredNumbers); // [5, 8, 12, 15]
This code filters the array to include only values between 5 and 15.
Example 4: Date Comparison
Title: Check If a Date is Within a Range
Another application is checking if a date falls within a specific range. Here’s how to do it:
1function isDateInRange(date, startDate, endDate) {
2 return date >= startDate && date <= endDate;
3}
4
5const today = new Date();
6const start = new Date('2024-01-01');
7const end = new Date('2024-12-31');
8
9console.log(isDateInRange(today, start, end)); // true or false
This function checks if today's date is within the year 2024.
Example 5: Working with Objects
Title: Filter Objects Based on Property Value
You can also filter objects based on a property value using our "between" logic:
1const people = [
2 { name: 'Alice', age: 25 },
3 { name: 'Bob', age: 30 },
4 { name: 'Charlie', age: 35 },
5];
6
7const filteredPeople = people.filter(person => isBetween(person.age, 28, 34));
8console.log(filteredPeople); // [{ name: 'Bob', age: 30 }]
This example filters an array of objects to find people within a certain age range.
Browser Compatibility
Most modern web browsers support these JavaScript features. You can expect consistent behavior across Chrome, Firefox, Safari, and Edge. However, always test your code in different environments to ensure compatibility.
Summary
In this tutorial, we explored essential concepts related to the idea of "between" in JavaScript. We learned how to create custom functions to check if numbers fall within a range and how to extract substrings. We provided examples that demonstrated various applications, from filtering arrays to working with dates and objects. Mastering these techniques will help you write cleaner and more efficient code. For further learning, consider checking out our JavaScript course or our HTML fundamentals course.
By understanding how to use these methods, you'll be better equipped to handle a variety of programming challenges. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
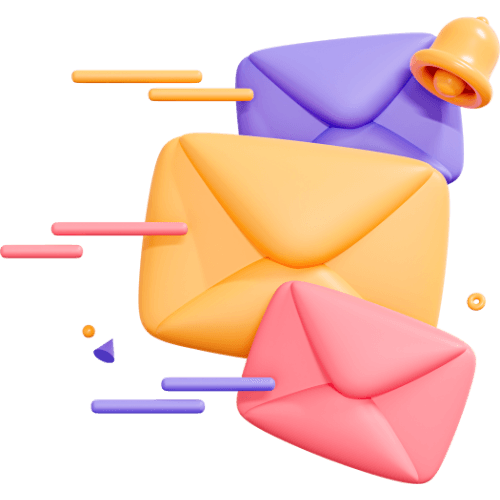
Related articles
9 Articles
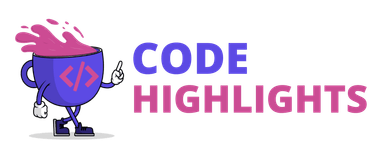
Copyright © Code Highlights 2025.