How to JavaScript Capitalize First Letter of Each Word

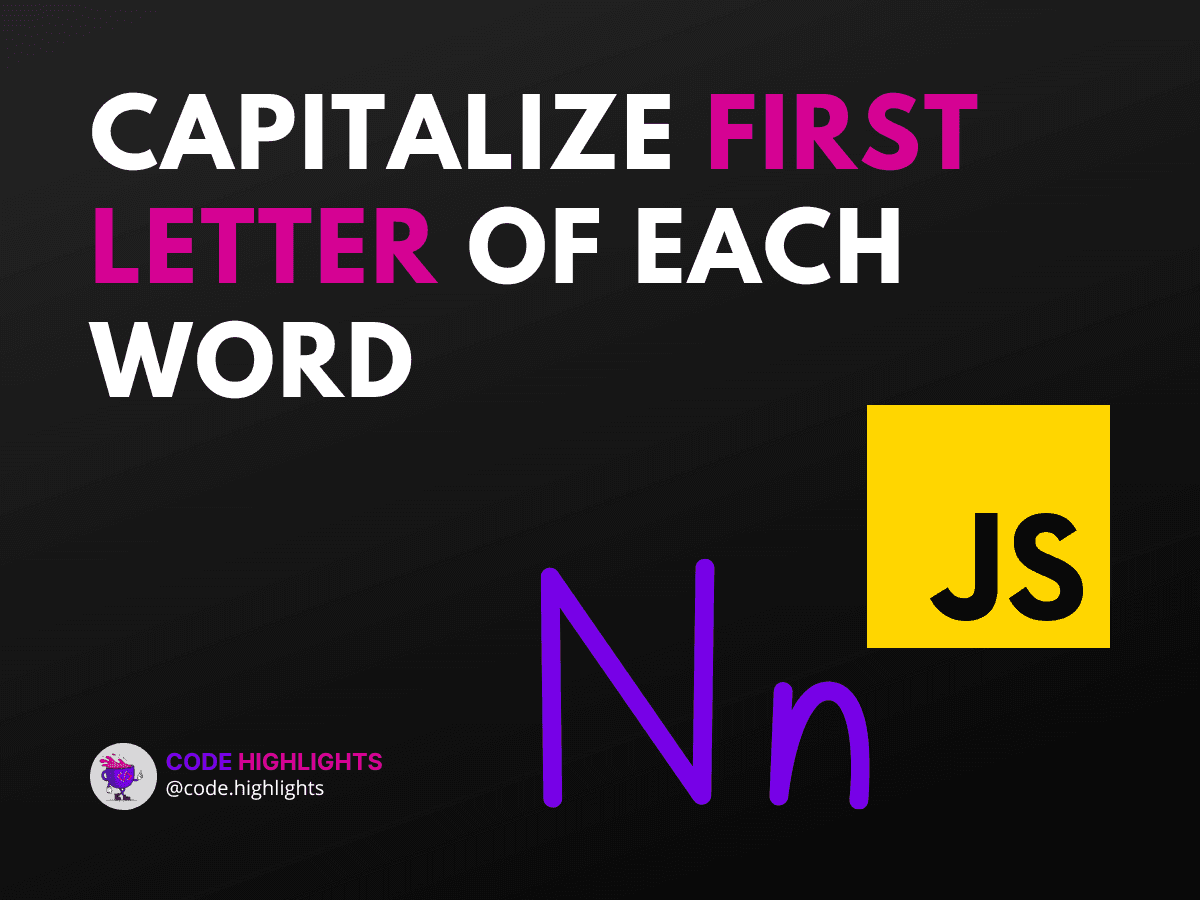
- Step-by-Step Guide to Capitalizing Words in JavaScript
- Understanding String Manipulation
- Capitalizing the First Letter
- Applying to Each Word
- Practical Examples
- Addressing Common Questions
- Further Learning
- Conclusion
When working with strings in JavaScript, you might come across a situation where you need to present text in a more readable or stylistically pleasing way. One common task is capitalizing the first letter of each word, often seen in titles or names. In this tutorial, we'll explore how to accomplish this using JavaScript, ensuring that even beginners can follow along and apply these techniques in their projects.
1function capitalizeFirstLetter(string) {
2 return string.charAt(0).toUpperCase() + string.slice(1);
3}
4console.log(capitalizeFirstLetter('hello world')); // Hello world
This introductory code snippet demonstrates the basic syntax for capitalizing the first letter of a string. However, our goal is to apply this concept to each word in a sentence. Let's dive into the details and learn how to javascript capitalize first letter
of every word.
Step-by-Step Guide to Capitalizing Words in JavaScript
Understanding String Manipulation
Before we tackle the problem, let's refresh our understanding of some fundamental JavaScript string methods that we'll use:
charAt()
: Returns the character at the specified index.toUpperCase()
: Converts the character(s) to uppercase.slice()
: Extracts a part of a string and returns it as a new string.split()
: Divides a string into an ordered list of substrates.
Capitalizing the First Letter
To capitalize the first letter of a string in JavaScript, we can follow these steps:
- Access the first character of the string with
charAt(0)
. - Convert this character to uppercase using
toUpperCase()
. - Concatenate the uppercase character with the rest of the string, excluding the first character, using
slice(1)
.
Here's a function that applies these steps:
1function capitalizeFirstLetter(string) {
2 return string.charAt(0).toUpperCase() + string.slice(1).toLowerCase();
3}
Applying to Each Word
Now, let's extend this logic to every word in a sentence:
1function capitalizeWords(sentence) {
2 return sentence.split(' ').map(word =>
3 word.charAt(0).toUpperCase() + word.slice(1).toLowerCase()
4 ).join(' ');
5}
In this function, we split
the sentence into words, map
over each word to capitalize the first letter, and then join
them back into a single string.
Practical Examples
Let's put our function to the test:
This result shows that our function correctly capitalizes the first letter of each word in the sentence.
Addressing Common Questions
-
How to make 1st letter capital in JavaScript? You can use the
capitalizeFirstLetter
function shown earlier to capitalize the first letter of a string. -
How do you capitalize first letter? Access the first character using
charAt(0)
, convert it to uppercase, and concatenate it with the rest of the string. -
How to uppercase in JavaScript? Use the
toUpperCase()
method to convert any character or string to uppercase. -
How to capitalize first letter using map in JavaScript? Use the
map
function on an array of words, applying the capitalization logic to each word, as demonstrated in thecapitalizeWords
function.
Further Learning
If you're interested in expanding your JavaScript knowledge, consider checking out our Learn JavaScript course, which dives deeper into the language's features and best practices.
For those looking to strengthen their web development foundations, our Introduction to Web Development course covers essential topics including HTML, CSS, and JavaScript.
And if you want to refine your understanding of HTML and CSS, our HTML Fundamentals Course and Learn CSS Introduction are great resources to explore.
Conclusion
Capitalizing the first letter of each word in JavaScript is a simple yet powerful string manipulation technique. By following the steps outlined in this tutorial, you can easily implement this functionality in your web applications. Remember to practice and experiment with the code examples provided to solidify your understanding.
For further reading and best practices, visit reputable sources like MDN Web Docs or W3Schools to deepen your knowledge of JavaScript string methods and their applications.
By integrating these techniques into your coding toolkit, you'll be well-equipped to handle text formatting challenges with ease. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
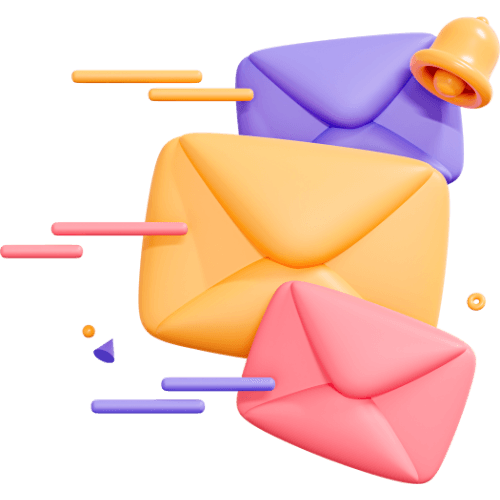
Related articles
9 Articles
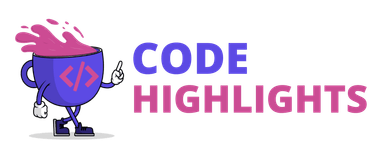
Copyright © Code Highlights 2025.