How to Achieve JavaScript Case Insensitive String Comparison Easily

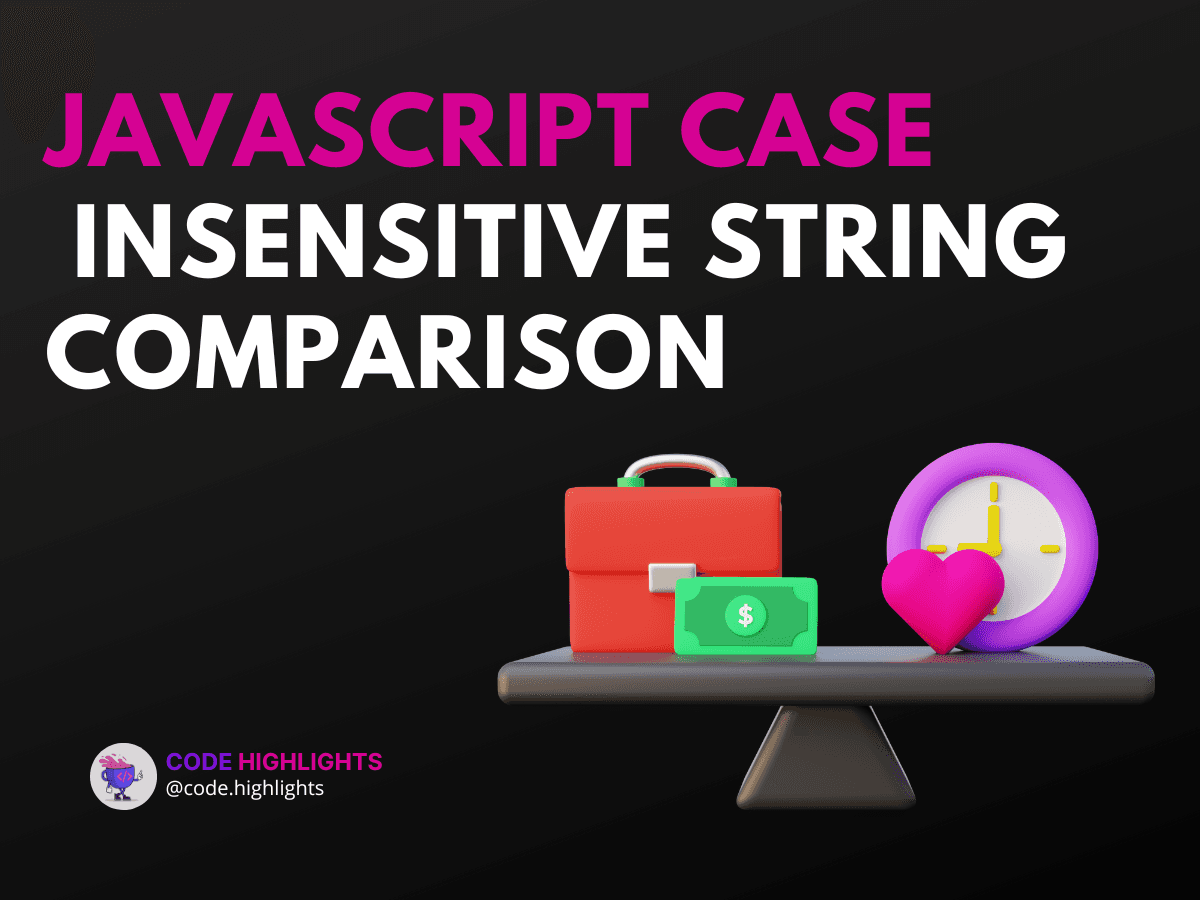
- Quick Example
- Understanding the Syntax
- Basic Comparison Example
- Unique Use Cases
- 1. Checking for Substrings
- 2. Case-Insensitive Array Search
- 3. Comparing User Input
- Compatibility with Browsers
- Summary
When working with text in JavaScript, you may need to compare strings. Sometimes, the case of the letters doesn't matter. For instance, "Hello" and "hello" should be seen as equal. This is where JavaScript case insensitive string comparison comes into play. In this tutorial, we will explore how to easily compare strings without worrying about their case.
Quick Example
Here's a simple code snippet to show how we can compare two strings without considering their case:
1function compareStrings(str1, str2) {
2 return str1.toLowerCase() === str2.toLowerCase();
3}
4
5console.log(compareStrings("Hello", "hello")); // true
This function converts both strings to lowercase before comparing them. Let's dive deeper into the syntax and variations!
Understanding the Syntax
To compare strings in a case-insensitive manner, we typically use the toLowerCase()
or toUpperCase()
methods. Here's how it works:
- Parameters: The function takes two strings as parameters.
- Return Value: It returns
true
if the strings are equal (ignoring case) andfalse
otherwise.
Basic Comparison Example
Here’s a quick example demonstrating the basic syntax:
1const str1 = "JavaScript";
2const str2 = "javascript";
3
4if (str1.toLowerCase() === str2.toLowerCase()) {
5 console.log("The strings are equal.");
6} else {
7 console.log("The strings are not equal.");
8}
Unique Use Cases
1. Checking for Substrings
Sometimes, you might want to check if one string contains another, regardless of case.
1function containsSubstring(mainStr, subStr) {
2 return mainStr.toLowerCase().includes(subStr.toLowerCase());
3}
4
5console.log(containsSubstring("Learning JavaScript", "java")); // true
2. Case-Insensitive Array Search
When searching through an array, you may want to find a match without case sensitivity.
1const fruits = ["Apple", "banana", "Cherry"];
2const searchFruit = "BANANA";
3
4const found = fruits.find(fruit => fruit.toLowerCase() === searchFruit.toLowerCase());
5
6console.log(found ? "Fruit found!" : "Fruit not found."); // Fruit found!
3. Comparing User Input
User input can often vary in case. Here’s how to handle that:
1function validateInput(userInput, correctAnswer) {
2 return userInput.toLowerCase() === correctAnswer.toLowerCase();
3}
4
5console.log(validateInput("Yes", "yes")); // true
Compatibility with Browsers
JavaScript's string methods like toLowerCase()
and toUpperCase()
are widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. You can confidently use these methods in your projects.
Summary
In this tutorial, we learned how to perform JavaScript case insensitive string comparison efficiently. We covered various techniques, including checking for equality, searching for substrings, and validating user input. These methods are essential for creating user-friendly applications that handle text input gracefully.
For those looking to deepen their understanding of JavaScript, consider exploring our JavaScript courses. If you're also interested in building web pages, check out our HTML Fundamentals Course and CSS Introduction.
For further reading on string comparisons in other programming languages, you might find this article on case-insensitive comparisons in Go helpful.
By mastering these concepts, you'll be better equipped to handle string comparisons in your web development projects. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
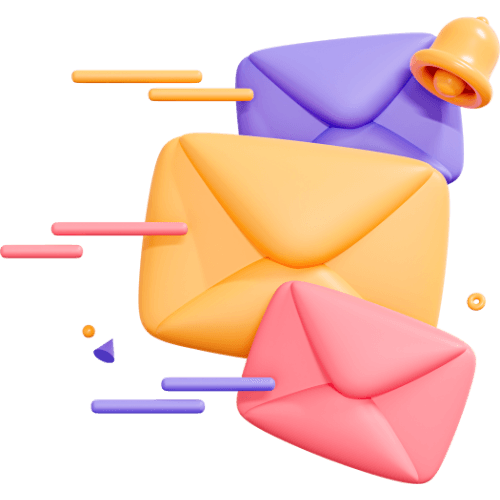
Related articles
9 Articles
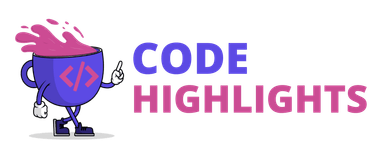
Copyright © Code Highlights 2025.