7 Easy Ways to Check if an Element Exists in JavaScript

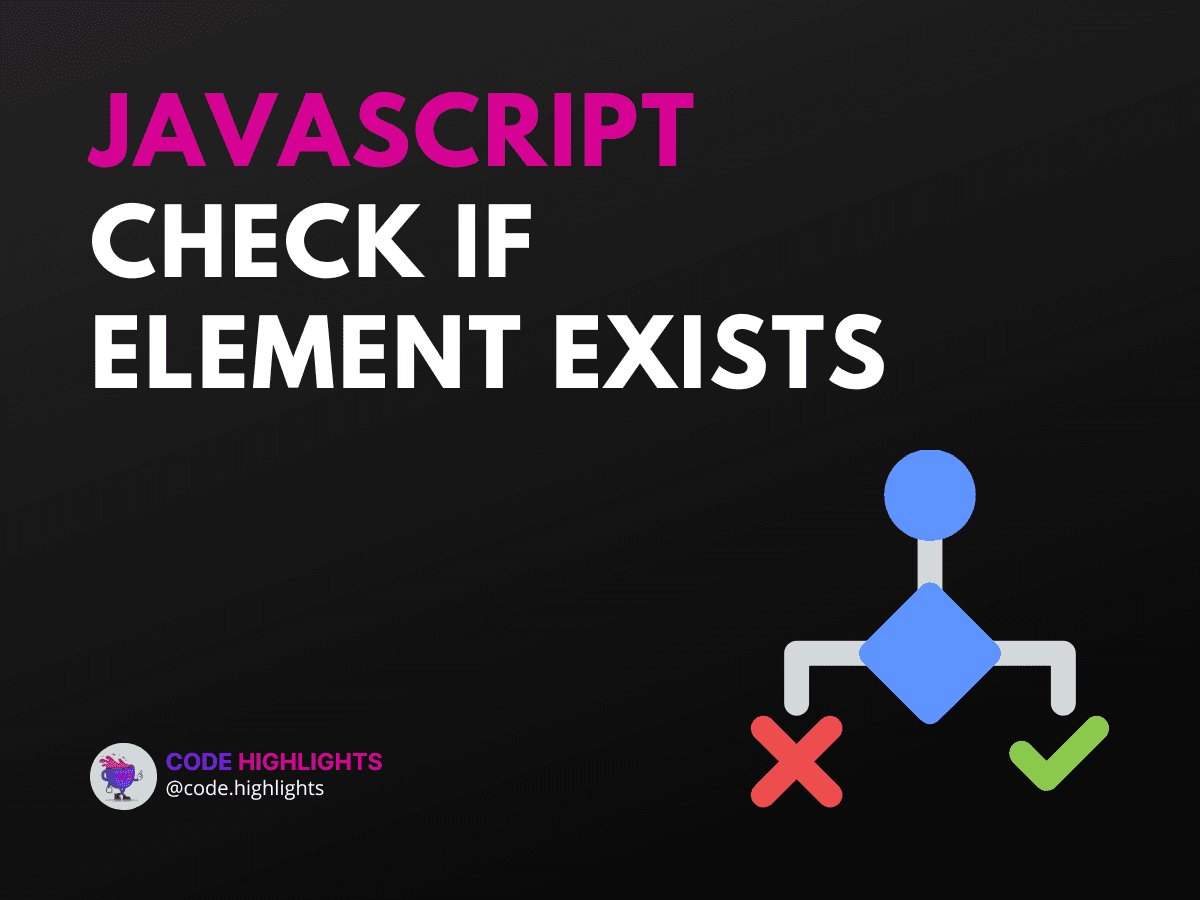
- 1. Using getElementById()
- Syntax:
- Example:
- 2. Using querySelector()
- Syntax:
- Example:
- 3. Using getElementsByClassName()
- Syntax:
- Example:
- 4. Using getElementsByTagName()
- Syntax:
- Example:
- 5. Using contains() Method
- Syntax:
- Example:
- 6. Using Array.from() with querySelectorAll()
- Syntax:
- Example:
- 7. Using length Property on NodeList
- Example:
- Browser Compatibility
- Summary
When working with web development, you often need to check if a specific element is present in the DOM (Document Object Model). This is crucial for ensuring your scripts run smoothly without errors. In this tutorial, we will explore seven easy methods to javascript check if element exists. Let’s dive right in with a quick code example to see how it works.
1if (document.getElementById('myElement')) {
2 console.log('Element exists!');
3} else {
4 console.log('Element does not exist.');
5}
1. Using getElementById()
The most straightforward way to check for an element is by using document.getElementById()
. This method returns the element with the specified ID or null
if it doesn’t exist.
Syntax:
- Parameters:
id
: A string representing the ID of the element.
- Return Value: The element object or
null
.
Example:
1const element = document.getElementById('header');
2if (element) {
3 console.log('Header exists');
4} else {
5 console.log('Header does not exist');
6}
2. Using querySelector()
Another way to check for an element is by using document.querySelector()
. This method allows you to select elements using CSS selectors.
Syntax:
- Parameters:
selector
: A string containing a CSS selector.
- Return Value: The first matching element or
null
.
Example:
1const element = document.querySelector('.myClass');
2if (element) {
3 console.log('Element with class "myClass" exists');
4} else {
5 console.log('Element with class "myClass" does not exist');
6}
3. Using getElementsByClassName()
You can also use document.getElementsByClassName()
to check if any elements with a specific class exist.
Syntax:
- Parameters:
className
: A string representing the class name.
- Return Value: An HTMLCollection of found elements.
Example:
1const elements = document.getElementsByClassName('item');
2if (elements.length > 0) {
3 console.log('Items exist');
4} else {
5 console.log('No items found');
6}
4. Using getElementsByTagName()
Similar to the previous method, document.getElementsByTagName()
checks for elements by their tag name.
Syntax:
- Parameters:
tagName
: A string representing the tag name.
- Return Value: An HTMLCollection of found elements.
Example:
1const paragraphs = document.getElementsByTagName('p');
2if (paragraphs.length > 0) {
3 console.log('Paragraphs exist');
4} else {
5 console.log('No paragraphs found');
6}
5. Using contains()
Method
If you have a parent element and want to check if it contains a specific child element, you can use the contains()
method.
Syntax:
- Parameters:
child
: The element to be checked.
- Return Value:
true
orfalse
.
Example:
1const parent = document.getElementById('parent');
2const child = document.getElementById('child');
3if (parent.contains(child)) {
4 console.log('Child exists within parent');
5} else {
6 console.log('Child does not exist within parent');
7}
6. Using Array.from()
with querySelectorAll()
You can convert a NodeList from querySelectorAll()
into an array and check its length.
Syntax:
- Parameters:
nodeList
: A NodeList returned byquerySelectorAll()
.
- Return Value: An array of elements.
Example:
1const elements = Array.from(document.querySelectorAll('.example'));
2if (elements.length > 0) {
3 console.log('Example elements exist');
4} else {
5 console.log('No example elements found');
6}
7. Using length
Property on NodeList
Lastly, when using querySelectorAll()
, you can directly check the length of the returned NodeList.
Example:
1const elements = document.querySelectorAll('.test');
2if (elements.length) {
3 console.log('Test elements exist');
4} else {
5 console.log('No test elements found');
6}
Browser Compatibility
All methods discussed are widely supported across major web browsers, including Chrome, Firefox, Safari, and Edge. However, always check compatibility for specific features if you're targeting older browsers.
Summary
In this tutorial, we explored javascript check if element exists using various methods like getElementById()
, querySelector()
, and more. Each method has its unique use case, making it essential to choose the right one based on your needs. Understanding these techniques is crucial for building robust web applications.
For further learning, consider checking out our courses on JavaScript, HTML Fundamentals, and CSS Introduction.
For more information on web development, visit MDN Web Docs and W3Schools. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
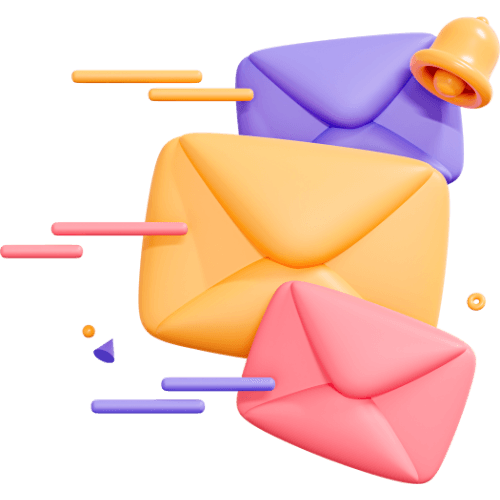
Related articles
9 Articles
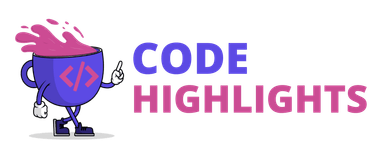
Copyright © Code Highlights 2024.