5 Quick Ways to Check If Key Exists in JavaScript

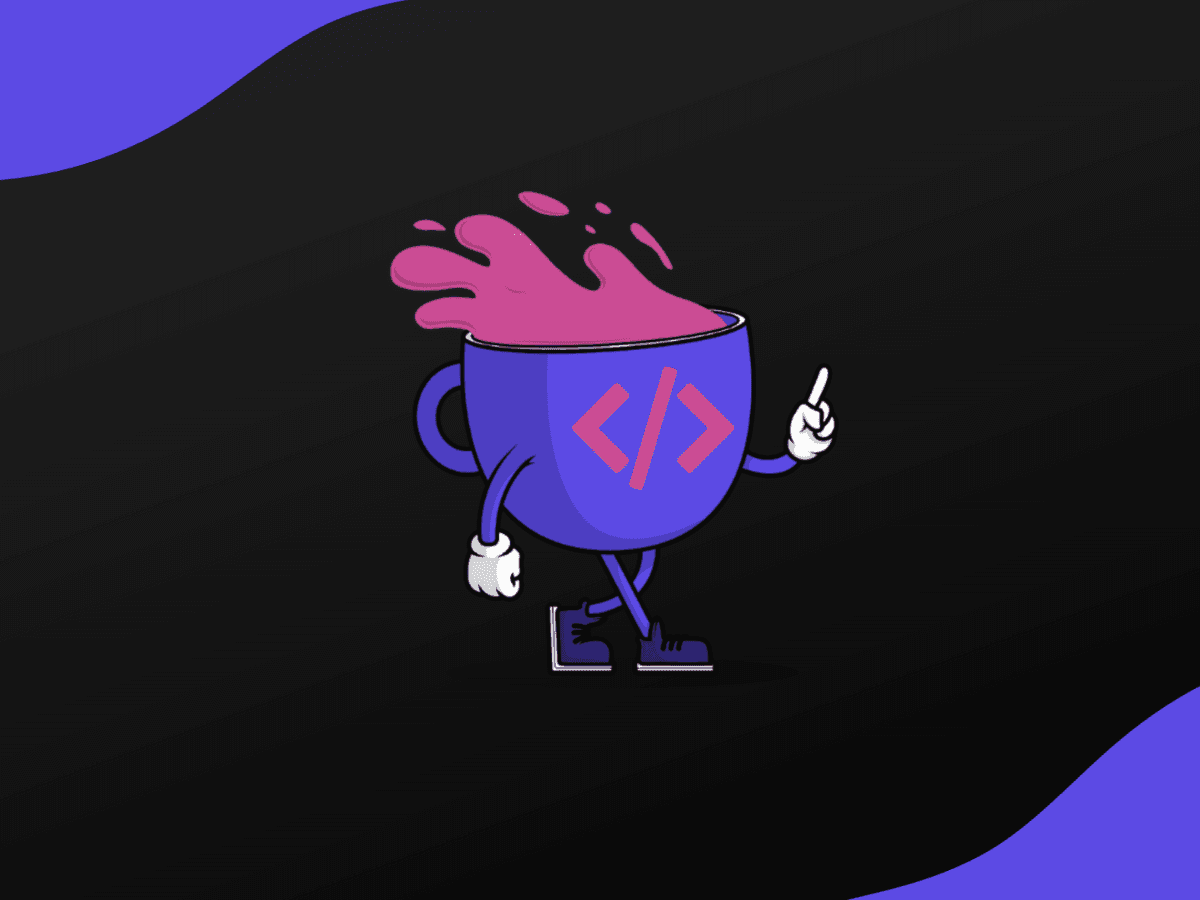
- in Operator
- hasOwnProperty()
- Object.keys()
- Object.getOwnPropertyNames()
- Map.has()
In JavaScript, objects and maps are commonly used to store data as key-value pairs. There are several ways to check if a key exists in these data structures. This tutorial will guide you through five quick methods.
in
Operator
The in
operator checks if a property is in an object. It returns true
if the property is found, false
otherwise.
1var obj = { key1: "value1" };
2console.log("key1" in obj); // returns true
3console.log("key2" in obj); // returns false
hasOwnProperty()
The hasOwnProperty()
method also checks if a property is in an object. Unlike the in
operator, it doesn't check the prototype chain.
1var obj = { key1: "value1" };
2console.log(obj.hasOwnProperty("key1")); // returns true
3console.log(obj.hasOwnProperty("key2")); // returns false
Object.keys()
The Object.keys()
method returns an array of an object's own property names. You can use the includes()
method to check if a key exists.
1var obj = { key1: "value1" };
2console.log(Object.keys(obj).includes("key1")); // returns true
3console.log(Object.keys(obj).includes("key2")); // returns false
Object.getOwnPropertyNames()
The Object.getOwnPropertyNames()
method returns an array of all properties found directly upon a given object. You can also use includes()
to check for a key.
1var obj = { key1: "value1" };
2console.log(Object.getOwnPropertyNames(obj).includes("key1")); // returns true
3console.log(Object.getOwnPropertyNames(obj).includes("key2")); // returns false
Map.has()
The Map.has()
method checks if an element with the specified key exists in a Map object.
1var map = new Map();
2map.set("key1", "value1");
3console.log(map.has("key1")); // returns true
4console.log(map.has("key2")); // returns false
For more JavaScript tutorials and tips, check out our Learn JavaScript course.
External Resources:
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
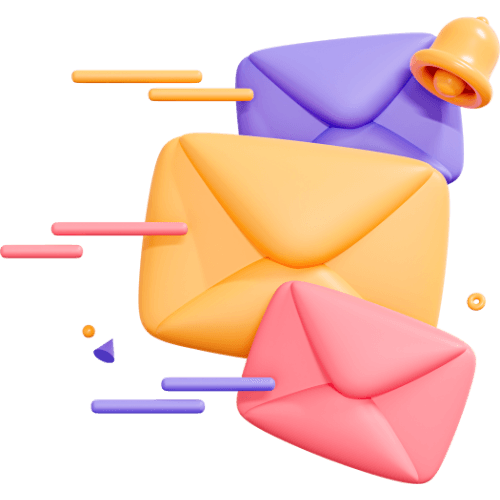
Related articles
9 Articles
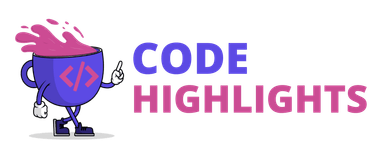
Copyright © Code Highlights 2025.