5 Quick Ways to Check If Key Exists in JSON Using JavaScript

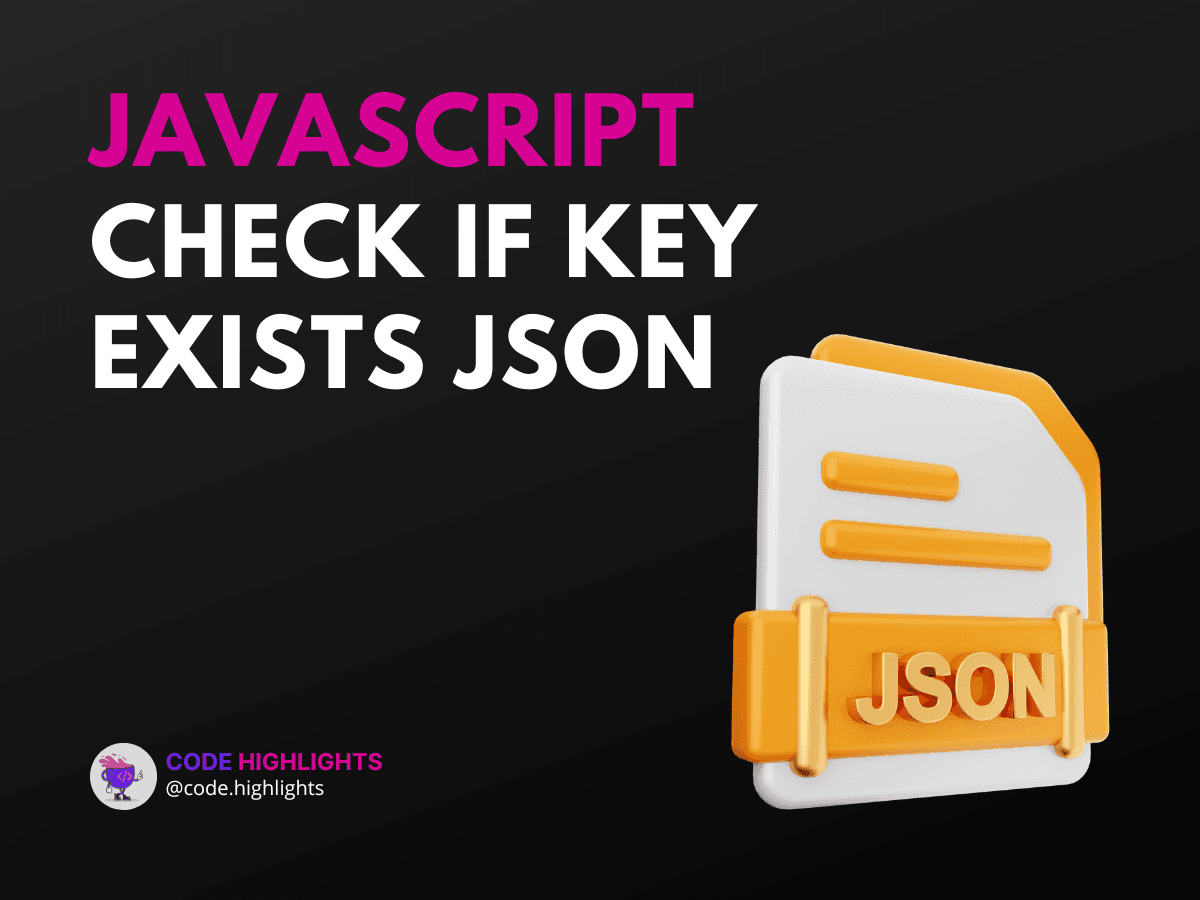
- Example Code Snippet
- Method 1: Using the in Operator
- Syntax
- Example
- Method 2: Using hasOwnProperty()
- Syntax
- Example
- Method 3: Using Object.keys()
- Syntax
- Example
- Method 4: Checking for a Key in Nested JSON
- Example Function
- Method 5: Using JSON.stringify()
- Example
- Compatibility with Major Browsers
- Summary
JSON (JavaScript Object Notation) is a popular format for data exchange. Sometimes, you need to check if a specific key exists in a JSON object. This can help prevent errors when accessing data. In this tutorial, we will explore five quick methods to do just that using JavaScript. Let's dive in!
Example Code Snippet
Here's a simple code snippet to get us started:
1const jsonData = {
2 "name": "Alice",
3 "age": 30,
4 "city": "New York"
5};
6
7console.log('name' in jsonData); // true
This example checks if the key name
exists in the jsonData
object.
Method 1: Using the in
Operator
The in
operator is a straightforward way to check for keys in an object. It returns true
if the key exists and false
otherwise.
Syntax
- Parameters:
key
: The key you want to check.object
: The JSON object you're checking against.
- Return Value: Boolean (
true
orfalse
).
Example
1if ('age' in jsonData) {
2 console.log('Key exists!');
3} else {
4 console.log('Key does not exist.');
5}
This will print "Key exists!" since age
is present.
Method 2: Using hasOwnProperty()
Another method to check for a key is by using the hasOwnProperty()
function. This method only checks for properties that belong directly to the object, not inherited ones.
Syntax
-
Parameters:
key
: The key you want to check.
-
Return Value: Boolean.
Example
This confirms that the city
key is indeed in jsonData
.
Method 3: Using Object.keys()
You can also use Object.keys()
to retrieve an array of keys from the object and then check if your desired key is included.
Syntax
-
Parameters:
object
: The JSON object.key
: The key you're looking for.
-
Return Value: Boolean.
Example
1const keys = Object.keys(jsonData);
2if (keys.includes('name')) {
3 console.log('Name key exists!');
4}
This will confirm the existence of the name
key.
Method 4: Checking for a Key in Nested JSON
Sometimes, JSON objects are nested. To check for a key in a nested structure, you can use a function.
Example Function
1function checkNested(obj, key) {
2 return key.split('.').reduce((o, x) => (o == null ? null : o[x]), obj) !== null;
3}
4
5const nestedData = {
6 user: {
7 details: {
8 name: 'Bob',
9 age: 25
10 }
11 }
12};
13
14console.log(checkNested(nestedData, 'user.details.name')); // true
This function splits the key string and checks each level of the object.
Method 5: Using JSON.stringify()
You can also convert your JSON object to a string and check if it contains the key. However, this method is less efficient.
Example
While this works, it's not the best practice due to performance issues.
Compatibility with Major Browsers
All these methods are compatible with major web browsers like Chrome, Firefox, and Safari. They follow standard JavaScript practices, ensuring consistent behavior across platforms.
Summary
In this tutorial, we explored five quick ways to check if a key exists in JSON using JavaScript. We covered the in
operator, hasOwnProperty()
, Object.keys()
, a custom function for nested JSON, and the JSON.stringify()
method. Each approach has its own use cases and advantages. Understanding these methods is essential for effective JavaScript programming, especially when dealing with JSON data.
For more about JavaScript, consider checking out our JavaScript course. If you're new to web development, you might find our HTML fundamentals course and CSS introduction course helpful. For a broader understanding, explore our introduction to web development.
Feel free to explore additional resources like MDN Web Docs on JSON for a deeper understanding of JSON and its usage in JavaScript.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
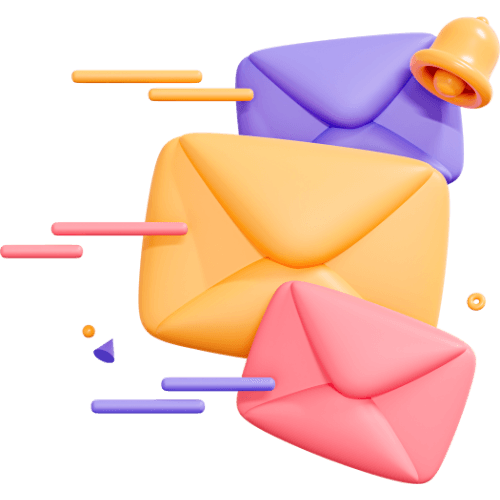
Related articles
9 Articles
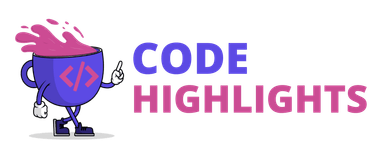
Copyright © Code Highlights 2025.