How to Determine if a JavaScript Object is Empty

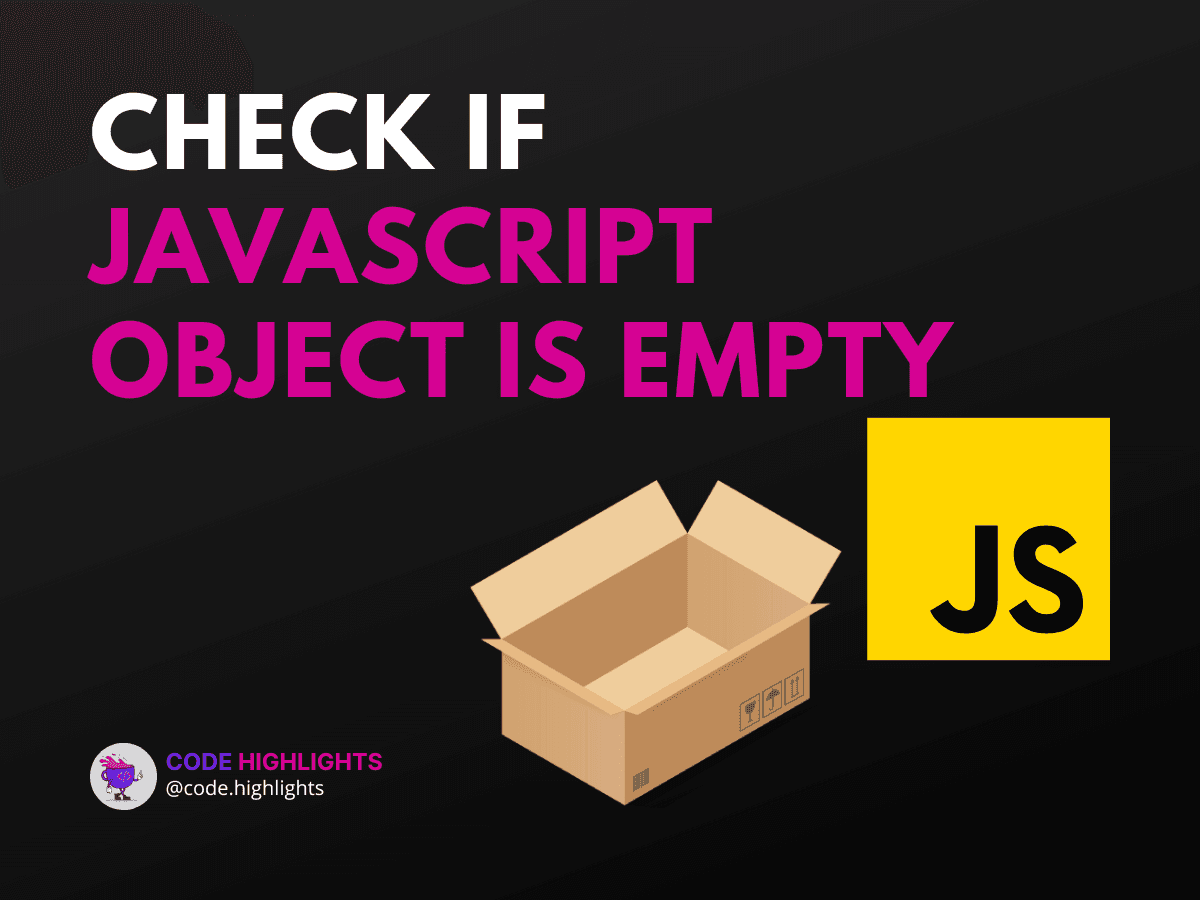
- Checking for an Empty Object in JavaScript
- Performance Considerations
- Undefined or Null Objects
- Checking in TypeScript
- External Resources for Further Reading
- Conclusion
In the realm of web development, working with objects is as common as using HTML to structure a webpage or CSS to style it. But sometimes, you may encounter a situation where you need to check if an object in JavaScript is devoid of properties—a truly empty object. This tutorial will guide you through various methods to accurately perform a javascript check if object is empty
, ensuring your code runs efficiently and error-free.
Let's start with a simple object and our first method of checking its emptiness:
1let exampleObject = {};
2
3function isEmpty(obj) {
4 return Reflect.ownKeys(obj).length === 0;
5}
6
7console.log(isEmpty(exampleObject)); // true
This introductory example uses the Reflect.ownKeys()
method to check if there are any properties within our object. If you're ready to dive deeper into this topic, let's begin!
Checking for an Empty Object in JavaScript
Performance Considerations
When it comes to checking if an object is empty in JavaScript performance is key. You don't want your method to slow down your application, especially if this check is done frequently. One efficient way is to use the Object.keys()
method:
This function returns true
if there are no keys in the object, meaning it is empty. It's a clean and fast solution that works well in most cases.
Undefined or Null Objects
Sometimes, objects might not just be empty; they could also be undefined
or null
. To check if an object is undefined or empty in JavaScript, you can enhance the previous function:
1function isObjectNullOrUndefinedOrEmpty(obj) {
2 return obj == null || Object.keys(obj).length === 0;
3}
This function checks if the object is null
or undefined
before checking for emptiness. It's a more robust solution that handles different cases.
Checking in TypeScript
TypeScript, a superset of JavaScript, provides static typing, which can help catch errors at compile time. To check if an object is empty in TypeScript, you can use a similar approach to JavaScript but with type annotations:
1function isObjectEmpty(obj: object): boolean {
2 return Object.keys(obj).length === 0;
3}
In TypeScript, you can specify that the obj
parameter is of type object
, making your intentions clear and your code safer.
External Resources for Further Reading
To solidify your understanding, consider reading about JavaScript objects at MDN Web Docs, exploring TypeScript basics, or reviewing performance tips for JavaScript.
Conclusion
By now, you should have a good grasp on how to determine if a JavaScript object is empty, whether you're working with plain JavaScript or TypeScript. Remember, an empty object is one without enumerable properties, and checking for this condition is a common task in web development.
If you're looking to deepen your knowledge in web development, consider exploring HTML fundamentals, CSS introduction, or furthering your JavaScript expertise.
Now, it's time to apply what you've learned and write clean, efficient code that handles objects like a pro!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
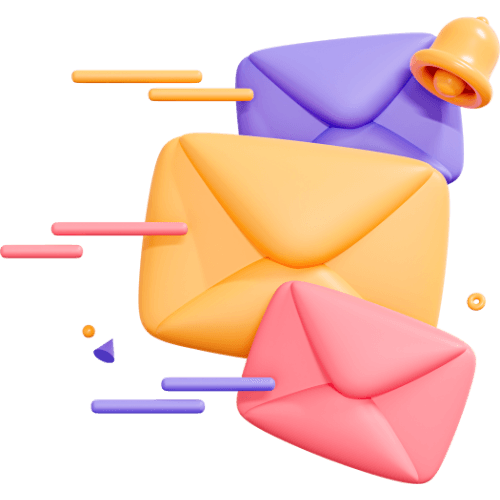
Related articles
9 Articles
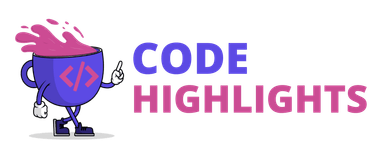
Copyright © Code Highlights 2024.