Javascript check if undefined or null is value

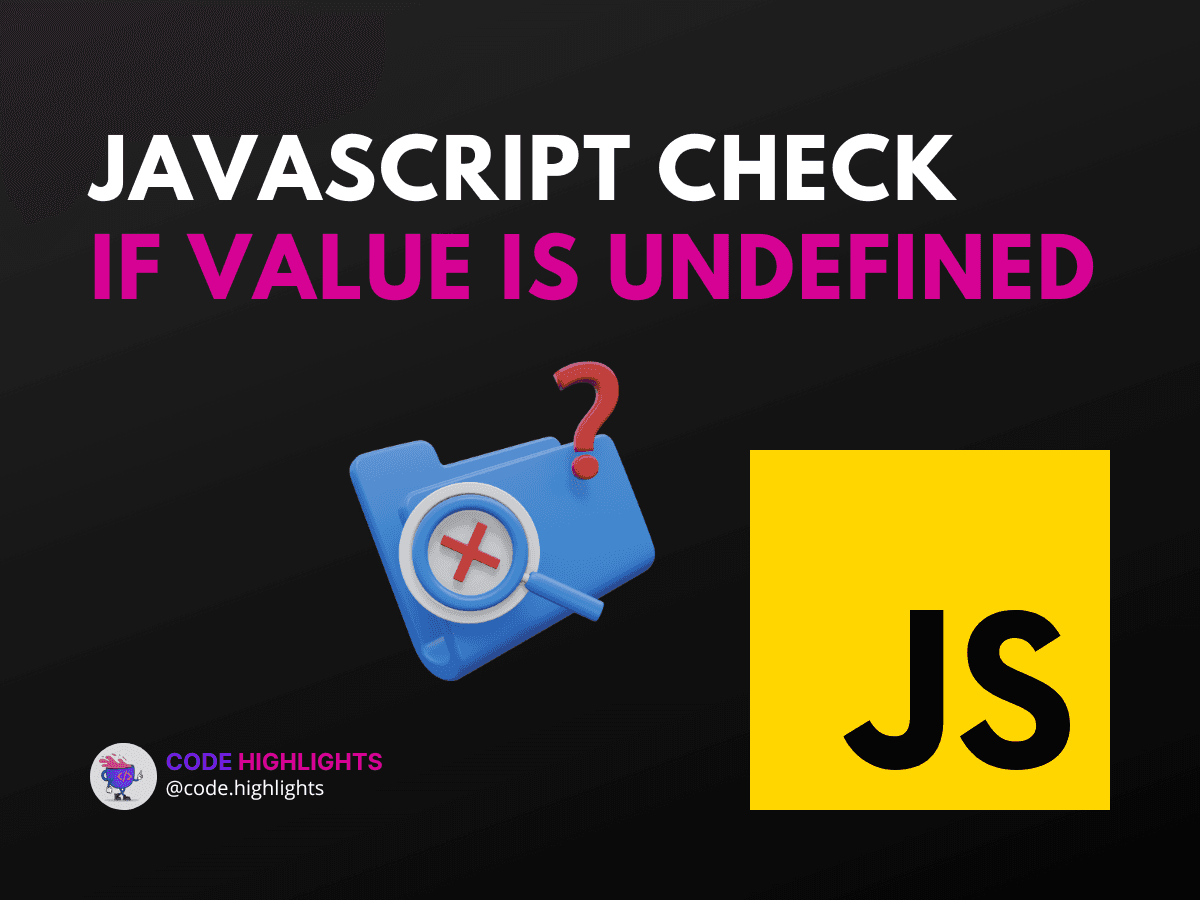
- Understanding undefined and null in JavaScript
- Checking for undefined
- Checking for null
- Checking for Both undefined and null
- Best Practices
- Conclusion
In the world of JavaScript, handling data types like undefined
and null
is a common task. Whether you're a beginner just starting out with JavaScript or an experienced developer brushing up on the fundamentals, understanding how to check for undefined
or null
values is crucial. Let's dive in with an introductory code snippet:
1let exampleVariable;
2console.log(typeof exampleVariable === "undefined"); // true
3
4exampleVariable = null;
5console.log(exampleVariable === null); // true
This tutorial will guide you through different methods to determine if a variable in JavaScript holds an undefined
or null
value. By mastering these techniques, you'll ensure your programs handle these cases gracefully, avoiding potential bugs and errors.
Understanding undefined
and null
in JavaScript
Before we proceed, it's essential to understand what undefined
and null
represent in JavaScript. An undefined
variable is one that has been declared but not assigned a value. On the other hand, null
is an assignment value that represents the intentional absence of any object value.
Checking for undefined
To check if a variable is undefined
, we can use the typeof
operator:
The typeof
operator returns a string indicating the type of the unevaluated operand. If the variable has not been assigned a value, it will return "undefined"
.
Checking for null
To check for null
, a strict equality check (===
) suffices:
Since null
is a primitive value, comparing using strict equality ensures that you are not mistaking it for undefined
or any other falsy value.
Checking for Both undefined
and null
Sometimes, you might want to check if a variable is either undefined
or null
. You can do this with a double equality check (==
):
1let myVar;
2// myVar is undefined
3if (myVar == null) {
4 console.log("myVar is undefined or null");
5}
6
7myVar = null;
8// myVar is now null
9if (myVar == null) {
10 console.log("myVar is undefined or null");
11}
Using ==
instead of ===
allows you to catch both undefined
and null
because null
and undefined
are equal in value when using loose equality.
Best Practices
When checking for undefined
or null
, consider the following best practices:
- Use
===
for comparisons to avoid unintentional type coercion. - When checking for both
undefined
andnull
,== null
is generally safe and concise. - Remember that
undefined
andnull
have different meanings, so treat them accordingly in your logic.
Conclusion
Dealing with undefined
and null
is a part of everyday coding in JavaScript. By understanding and correctly implementing checks for these values, you can write more robust and error-free code. Continue your learning journey by exploring more about HTML, CSS, and web development to build upon your JavaScript knowledge.
For further reading on JavaScript best practices and handling undefined
and null
, consider these reputable sources:
By integrating these checks into your JavaScript code, you're on your way to creating more reliable and maintainable web applications. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
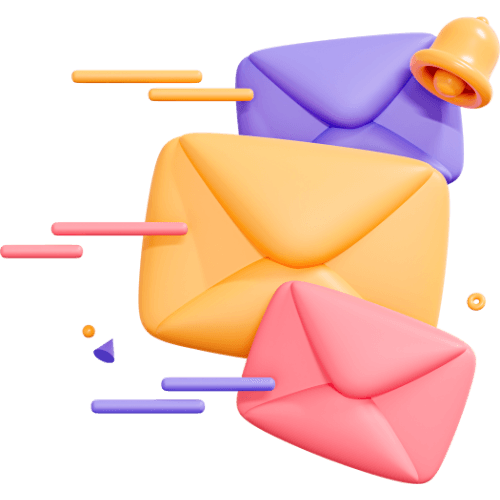
Related articles
114 Articles
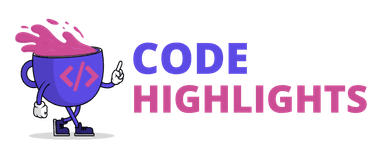
Copyright © Code Highlights 2024.