3 Simple Methods to JavaScript Clear Array in 2024

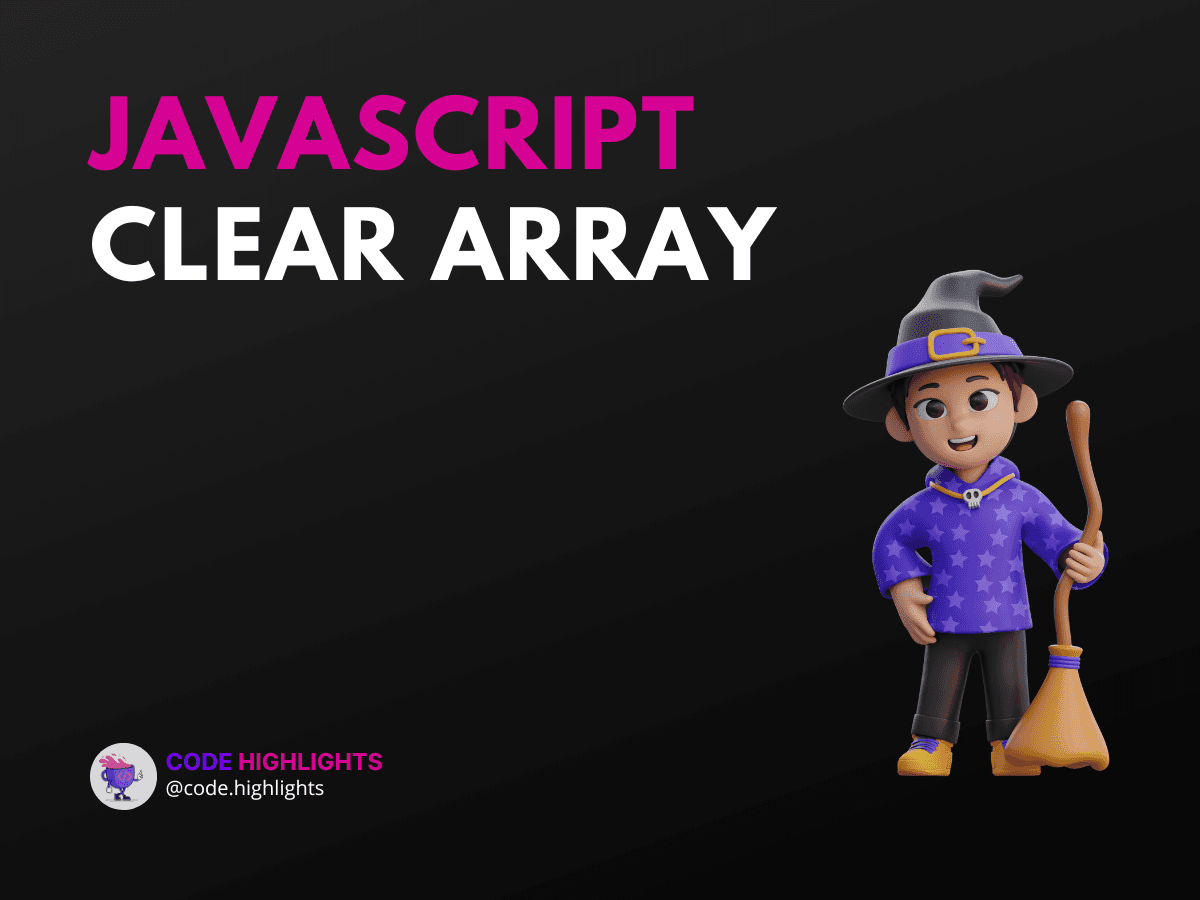
- Introduction Code Example
- Method 1: Setting the Length to Zero
- Method 2: Using the splice Method
- Method 3: Reassigning to an Empty Array
- Compatibility with Major Web Browsers
- Summary
Clearing an array in JavaScript is a common task that often puzzles beginners. Whether you're working on a web application or just practicing coding, knowing how to clear an array efficiently can save you time and effort. In this tutorial, we'll explore three simple methods to clear an array in JavaScript.
Introduction Code Example
Now, let's dive into the methods to clear this array.
Method 1: Setting the Length to Zero
One of the quickest ways to clear an array is by setting its length property to zero.
Syntax
Example
This method is efficient and straightforward, making it a popular choice among developers.
Method 2: Using the splice
Method
The splice
method can also be used to clear an array by removing all its elements.
Syntax
Example
1let myArray = [1, 2, 3, 4, 5];
2myArray.splice(0, myArray.length);
3console.log(myArray); // Output: []
This method is versatile as splice
can also be used to remove specific elements from the array.
Method 3: Reassigning to an Empty Array
Another simple way to clear an array is by reassigning it to a new empty array.
Syntax
Example
While this method effectively clears the array, it may not affect other references to the original array.
Compatibility with Major Web Browsers
All these methods are compatible with major web browsers, including Chrome, Firefox, Safari, and Edge. They adhere to standard JavaScript behavior, ensuring consistent performance across different environments.
Summary
In this tutorial, we explored three simple methods to clear an array in JavaScript:
- Setting the length to zero.
- Using the
splice
method. - Reassigning to an empty array.
Each method has its own advantages and can be used based on the specific requirements of your project. Clearing arrays efficiently is a fundamental skill in JavaScript, and mastering these methods will enhance your coding proficiency.
For further learning, consider diving deeper into JavaScript through our Learn JavaScript course. Additionally, understanding HTML and CSS is crucial for web development, so check out our HTML Fundamentals Course and Introduction to CSS.
For more information on JavaScript arrays, you can visit MDN Web Docs on Arrays and W3Schools Array Methods.
By mastering these techniques, you'll be well-equipped to handle arrays in your JavaScript projects, making your code cleaner and more efficient. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
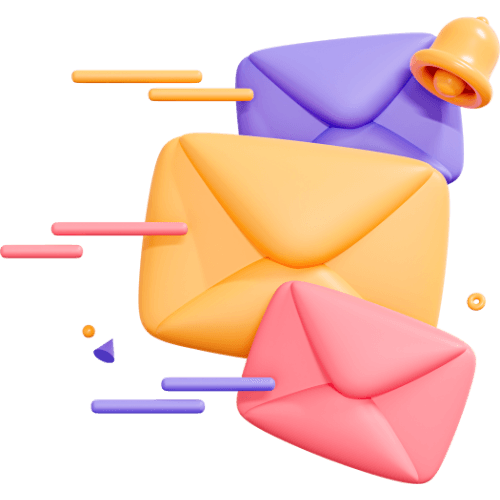
Related articles
9 Articles
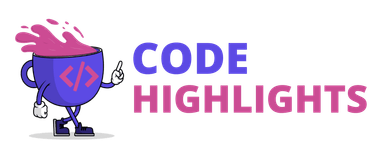
Copyright © Code Highlights 2025.