How to Easily Compare Dates with JavaScript

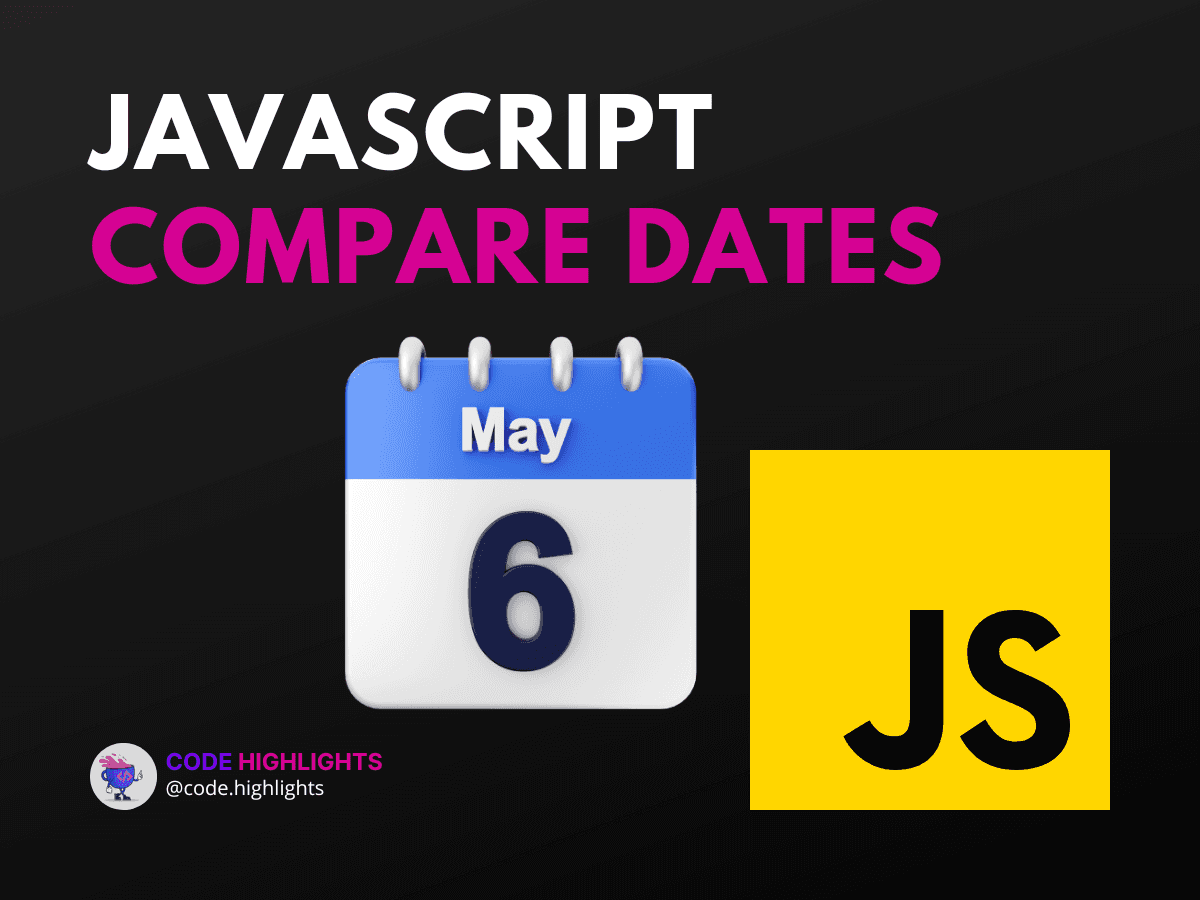
- Understanding JavaScript Date Objects
- Comparing Dates in JavaScript
- Checking Equality
- Finding the Earlier or Later Date
- Calculating the Difference Between Dates
- Best Practices and Pitfalls
- Conclusion
When working with web applications, it's quite common to need to compare dates. Whether you're checking if one date is before another, calculating the difference between dates, or simply sorting a list of dates, JavaScript provides you with several methods to achieve this task efficiently. In this tutorial, we'll explore how to compare dates in JavaScript, ensuring that your web projects handle time-sensitive data accurately.
Let's start with a simple example:
1let date1 = new Date('2021-03-25');
2let date2 = new Date('2021-04-01');
3
4console.log(date1 < date2); // true
In the code above, we create two Date
objects and then use a comparison operator to check if date1
is before date2
. This is just a teaser of what you can do with JavaScript when comparing dates.
Before we dive deeper, consider brushing up on your JavaScript skills, as well as the basics of HTML and CSS if you plan to implement these comparisons in a web environment. For a more comprehensive understanding, you might also want to check out this introduction to web development.
Understanding JavaScript Date Objects
To compare dates effectively, you need to understand how JavaScript handles dates. The Date
object in JavaScript is used to work with dates and times. Here's how you can create a new Date
object:
This snippet creates a new Date
object for the current date and time. You can also initialize a Date
object with specific date strings or values.
Comparing Dates in JavaScript
Now, let's look at how to compare two different dates to see which comes first, or if they represent the same moment in time.
Checking Equality
To check if two dates are equal in JavaScript:
We use the getTime
method which returns the number of milliseconds since the Unix Epoch (January 1, 1970). It's a reliable way to compare dates because it converts them into numbers.
Finding the Earlier or Later Date
To find out which date is earlier or later:
1let isEarlier = date1.getTime() < date2.getTime();
2let isLater = date1.getTime() > date2.getTime();
3
4console.log(isEarlier); // true
5console.log(isLater); // false
Calculating the Difference Between Dates
Sometimes you might want to know the difference between two dates:
1let diff = date2.getTime() - date1.getTime();
2let daysDifference = diff / (1000 * 3600 * 24);
3
4console.log(daysDifference); // 7
In this example, we calculate the difference in milliseconds and then convert it to days.
Best Practices and Pitfalls
When comparing dates, be aware of time zones and daylight saving time changes. These factors can affect date comparisons. Always consider using libraries like Moment.js or Date-fns if you need to handle complex date manipulations.
Conclusion
Comparing dates in JavaScript is straightforward once you understand how the Date
object works. By using methods like getTime
, you can easily compare dates to sort them, check equality, or calculate differences.
Remember, the key to mastering JavaScript date comparisons is practice. Try out these examples in your own projects, and soon you'll be handling dates like a pro. If you found this tutorial helpful, consider deepening your knowledge with more advanced JavaScript courses.
For further reading on JavaScript and date manipulation, check out these resources:
By following these steps, you should now be able to confidently compare dates in JavaScript, ensuring your web applications handle temporal data with ease. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
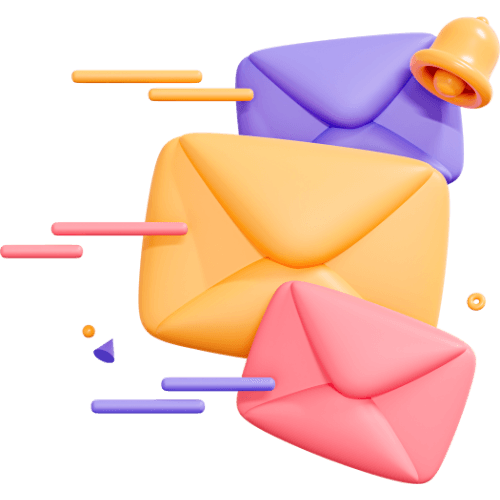
Related articles
9 Articles
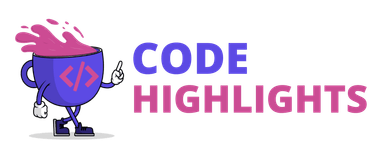
Copyright © Code Highlights 2025.