The Ultimate Guide to Javascript Compare Strings Ignore Case

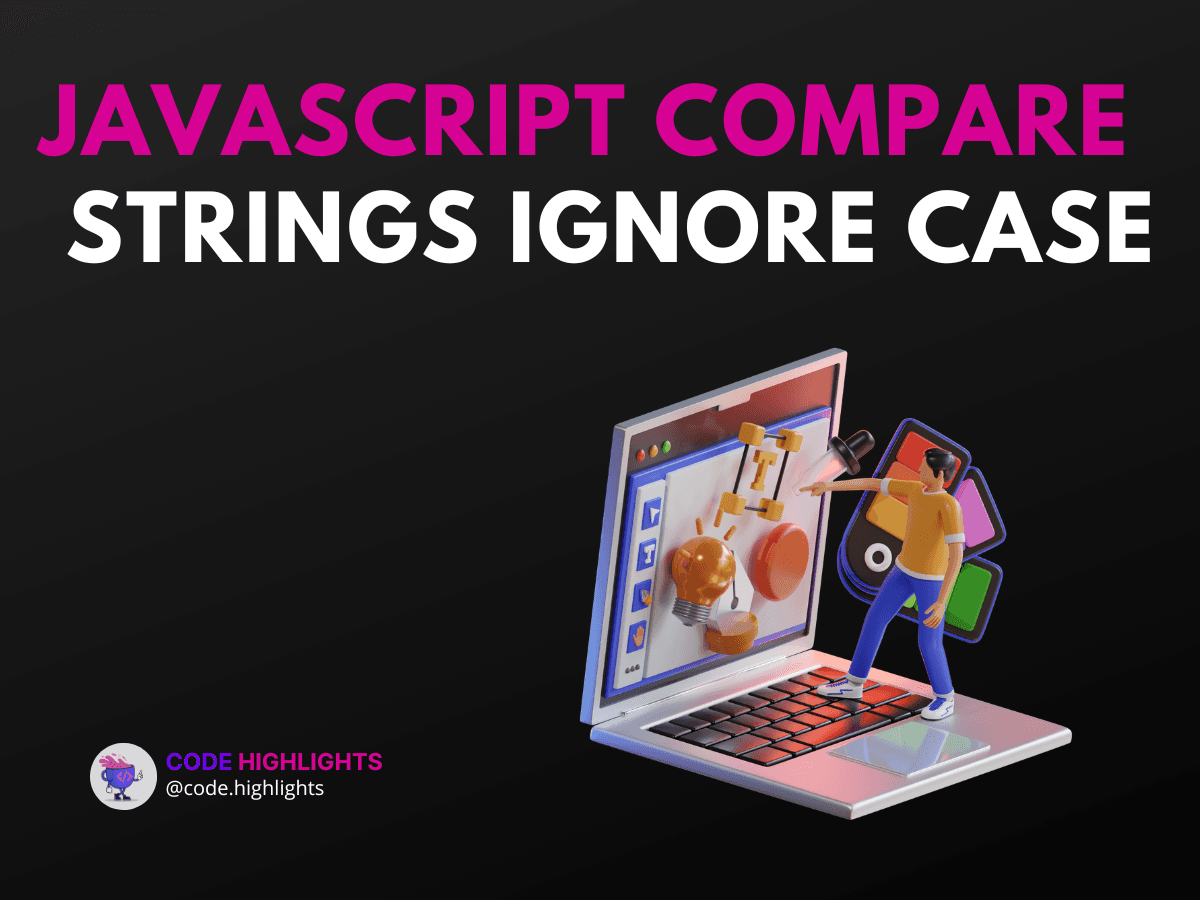
- Basic Syntax for String Comparison
- How to Use .toLowerCase() and .toUpperCase()
- Syntax Explanation
- Code Snippet Example
- Example 1: Comparing User Input
- Scenario: Case-Insensitive Login Check
- Example 2: Searching in an Array
- Scenario: Finding Matches in a List
- Example 3: Sorting Strings Ignoring Case
- Scenario: Alphabetical Sorting
- Browser Compatibility
- Conclusion
When working with strings in JavaScript, comparing them can sometimes be tricky, especially when you want to ignore case differences. For instance, "hello" and "HELLO" should be considered equal. In this guide, we will explore how to effectively compare strings while ignoring their case. This is useful in various applications, such as user input validation and search functionalities.
Basic Syntax for String Comparison
To compare two strings in JavaScript while ignoring their case, we can use the .toLowerCase()
or .toUpperCase()
methods. Here's a simple example to get started:
1let str1 = "Hello";
2let str2 = "hello";
3
4if (str1.toLowerCase() === str2.toLowerCase()) {
5 console.log("The strings are equal!");
6} else {
7 console.log("The strings are not equal.");
8}
In this code, both strings are converted to lowercase before comparison.
How to Use .toLowerCase()
and .toUpperCase()
Syntax Explanation
- Parameters: No parameters are needed for these methods.
- Return Value: Both methods return a new string with all characters converted to lower or upper case.
Code Snippet Example
1let originalString = "JavaScript";
2let lowerCaseString = originalString.toLowerCase(); // "javascript"
3let upperCaseString = originalString.toUpperCase(); // "JAVASCRIPT"
This demonstrates how to use these methods to transform strings for comparison.
Example 1: Comparing User Input
Scenario: Case-Insensitive Login Check
In a login system, you might want to check if the username entered by the user matches a stored username regardless of case:
1let storedUsername = "User123";
2let inputUsername = "user123";
3
4if (storedUsername.toLowerCase() === inputUsername.toLowerCase()) {
5 console.log("Access granted!");
6} else {
7 console.log("Access denied!");
8}
This ensures that the login process is user-friendly.
Example 2: Searching in an Array
Scenario: Finding Matches in a List
You can also compare strings to find matches in an array while ignoring case. Here’s how:
1let names = ["Alice", "Bob", "Charlie"];
2let searchName = "bob";
3
4let found = names.some(name => name.toLowerCase() === searchName.toLowerCase());
5
6console.log(found ? "Name found!" : "Name not found.");
In this example, we use the .some()
method to check if any name matches the search term.
Example 3: Sorting Strings Ignoring Case
Scenario: Alphabetical Sorting
When sorting strings, you might want to ignore case as well. Here’s a quick way to do this:
1let fruits = ["banana", "Apple", "cherry", "apple"];
2
3fruits.sort((a, b) => a.toLowerCase().localeCompare(b.toLowerCase()));
4
5console.log(fruits); // ["Apple", "apple", "banana", "cherry"]
This shows how to sort an array of strings without considering their case.
Browser Compatibility
The methods .toLowerCase()
and .toUpperCase()
are widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. This means you can confidently use these methods in your web applications.
Conclusion
In summary, comparing strings in JavaScript while ignoring case is straightforward using the .toLowerCase()
and .toUpperCase()
methods. This technique is essential for developing user-friendly applications, ensuring that inputs are treated fairly regardless of how they are typed. Whether you’re validating user input or searching through data, this approach will enhance your coding efficiency.
For more in-depth learning, consider exploring our JavaScript courses or dive into HTML fundamentals and CSS introduction.
For further reading on string comparison and related topics, check out resources from MDN Web Docs and W3Schools.
By mastering how to compare strings in JavaScript, you’ll be better equipped to handle various programming challenges!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
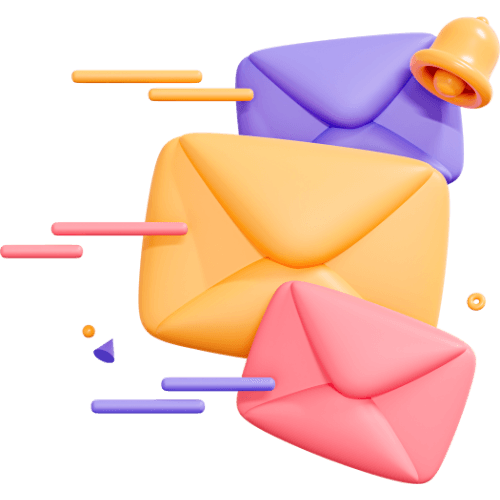
Related articles
9 Articles
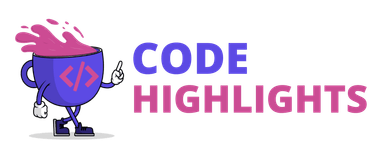
Copyright © Code Highlights 2024.