How to Concatenate Strings in JavaScript Like a Pro

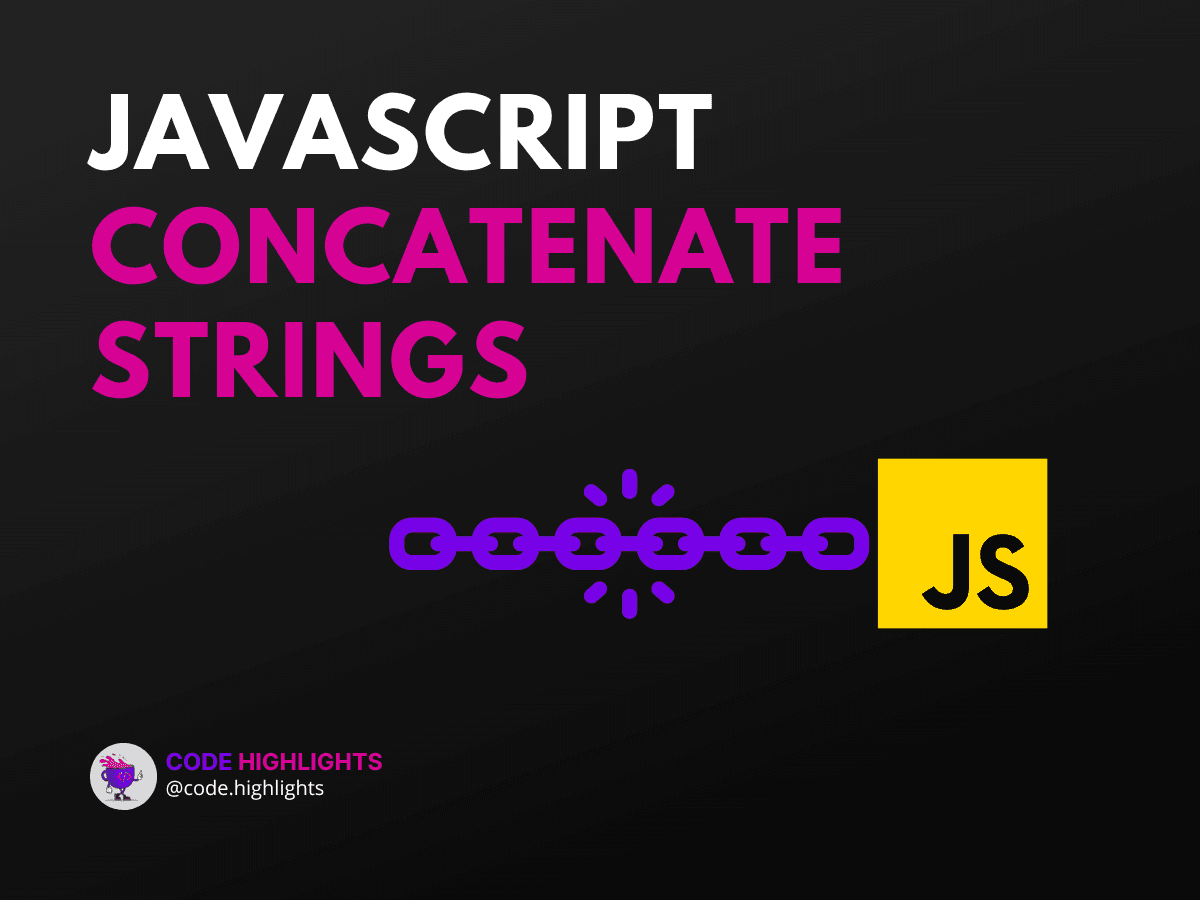
- Understanding String Concatenation in JavaScript
- Using the Plus Operator (+)
- The concat() Method
- Template Literals (ES6+)
- Joining Multiple Strings
- Best Practices for Concatenating Strings
- Conclusion
Whether you're building dynamic web content, managing form inputs, or simply manipulating text, understanding how to join strings together is a fundamental skill in programming. Before we delve deeper, let's take a quick look at a simple example:
1let greeting = "Hello, ";
2let name = "world!";
3let welcomeMessage = greeting + name;
4console.log(welcomeMessage); // Outputs: Hello, world!
In this tutorial, we will explore various ways to javascript concatenate strings
, ensuring your code is not only functional but also clean and efficient. By the end, you'll be concatenating strings like a pro!
Understanding String Concatenation in JavaScript
String concatenation is the process of appending one string to another to create a single string. In JavaScript, this can be achieved in several ways.
Using the Plus Operator (+
)
The most common method to concatenate two strings is by using the +
operator:
1let firstName = "Jane";
2let lastName = "Doe";
3let fullName = firstName + " " + lastName;
4console.log(fullName); // Outputs: Jane Doe
This method is straightforward and works well for simple concatenations.
The concat()
Method
For those wondering what concat()
in JavaScript is, it's a method provided by the String prototype that combines two or more strings:
1let str1 = "Hello";
2let str2 = " world!";
3let combinedStr = str1.concat(str2);
4console.log(combinedStr); // Outputs: Hello world!
This method is elegant and expressive, making it clear that you are performing string concatenation.
Template Literals (ES6+)
With the introduction of ES6, template literals provide a new way to concatenate strings using backticks and ${}
placeholders:
1let user = "Alice";
2let greetingMessage = `Welcome back, ${user}!`;
3console.log(greetingMessage); // Outputs: Welcome back, Alice!
Template literals can include expressions, making them incredibly powerful for creating dynamic strings.
Joining Multiple Strings
When you need to concatenate string plus more strings, you can chain the +
operator or use the concat()
method multiple times:
1let part1 = "JavaScript ";
2let part2 = "is ";
3let part3 = "awesome!";
4let sentence = part1 + part2 + part3;
5console.log(sentence); // Outputs: JavaScript is awesome!
Or with concat()
:
1let sentence = part1.concat(part2, part3);
2console.log(sentence); // Outputs: JavaScript is awesome!
Best Practices for Concatenating Strings
While there are several ways to concatenate strings in JavaScript, choosing the right method depends on your specific use case. For readability and maintainability, template literals are often the preferred choice for complex concatenations. However, for simple appends, the +
operator is quick and convenient.
If you're interested in learning more about JavaScript, check out our JavaScript course. And if you're new to web development, our introduction to web development course covers the essentials you need to get started.
To strengthen your front-end skills, consider exploring our HTML fundamentals course and our CSS introduction course.
Conclusion
Now that you know how to concatenate strings in JavaScript, you can start implementing this knowledge in your projects. Remember, practice makes perfect. Try out different methods and see which one you prefer. Happy coding!
For further reading on JavaScript and string manipulation, consider these external sources:
Concatenating strings is just the beginning. As you continue to learn and grow as a developer, you'll find that these skills form the foundation for more advanced JavaScript techniques. Keep exploring, keep coding, and watch your applications come to life!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
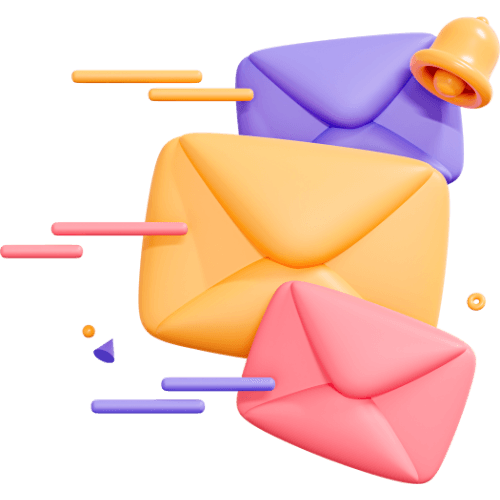
Related articles
9 Articles
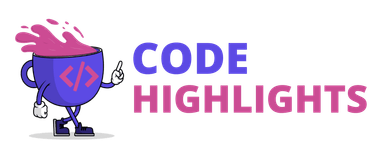
Copyright © Code Highlights 2025.