5 Quick Ways to Copy Arrays in JavaScript

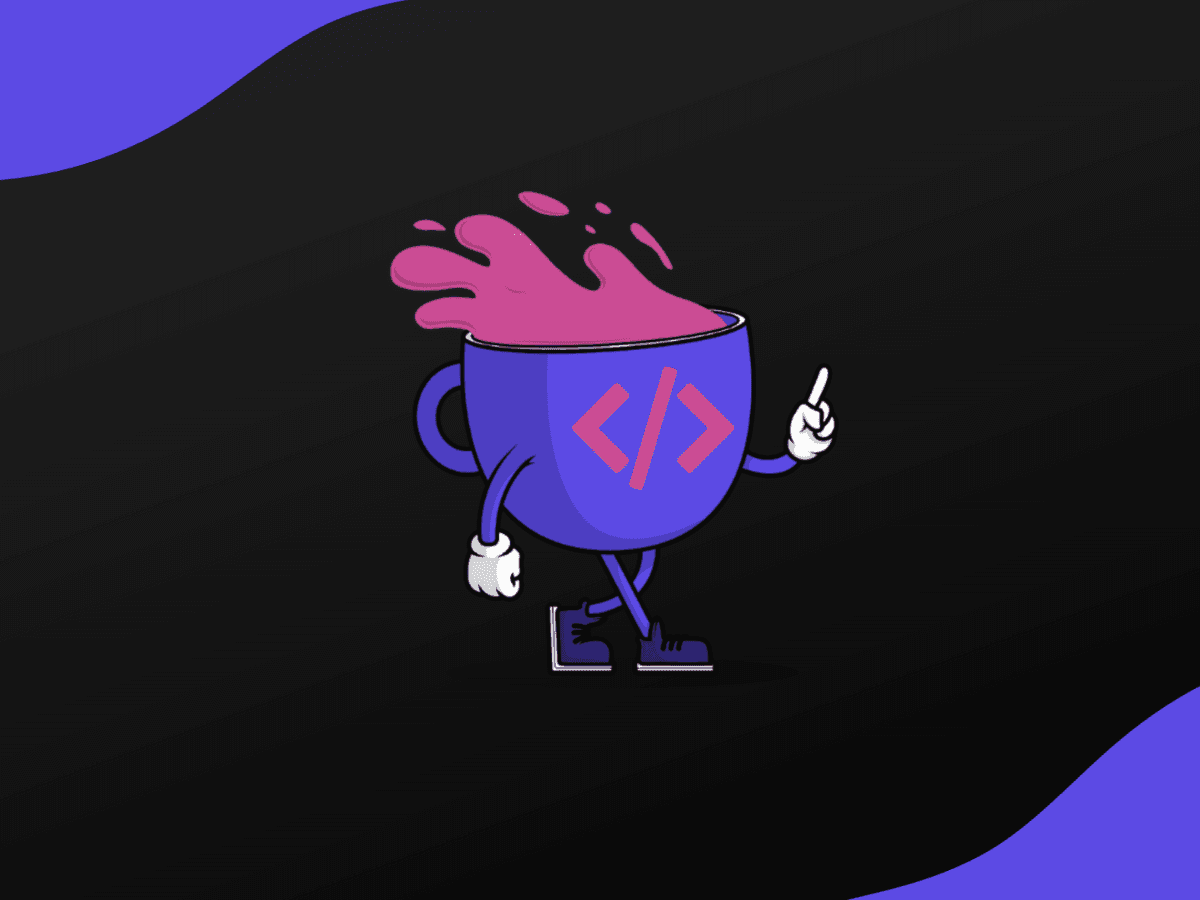
- Array.prototype.slice()
- Array.prototype.concat()
- Spread Operator
- Array.from()
- Deep Copying
In JavaScript, an array can be copied in several ways. However, it's crucial to understand the difference between shallow and deep copying. Let's explore some of these methods.
Array.prototype.slice()
The slice()
method returns a shallow copy of an array into a new array object.
1var arr1 = ['a', 'b', 'c'];
2var arr2 = arr1.slice();
3console.log(arr2); // Will log ['a', 'b', 'c']
This method is fast, but only creates a shallow copy. So, for arrays containing objects, the copied objects are shared between the original and copied array.
Array.prototype.concat()
Like slice()
, the concat()
method also returns a shallow copy of an array.
1var arr1 = ['a', 'b', 'c'];
2var arr2 = arr1.concat();
3console.log(arr2); // Will log ['a', 'b', 'c']
Spread Operator
ES6 introduced the spread operator ...
which can be used to create a shallow copy of an array.
This is the fastest way to copy an array in JavaScript.
Array.from()
Array.from()
method creates a new array instance from a given array.
1var arr1 = ['a', 'b', 'c'];
2var arr2 = Array.from(arr1);
3console.log(arr2); // Will log ['a', 'b', 'c']
Deep Copying
For deep copying, we can use JSON.parse()
and JSON.stringify()
methods.
1var arr1 = ['a', 'b', 'c'];
2var arr2 = JSON.parse(JSON.stringify(arr1));
3console.log(arr2); // Will log ['a', 'b', 'c']
In our JavaScript course, you can learn more about these and other JavaScript features. If you're new to web development, start with our introduction to web development course.
For a deeper understanding of arrays, check out this MDN Web Docs guide. If you want to dive into the ES6 spread operator, this JavaScript.info article is a great resource.
Remember, practice is key in mastering JavaScript. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
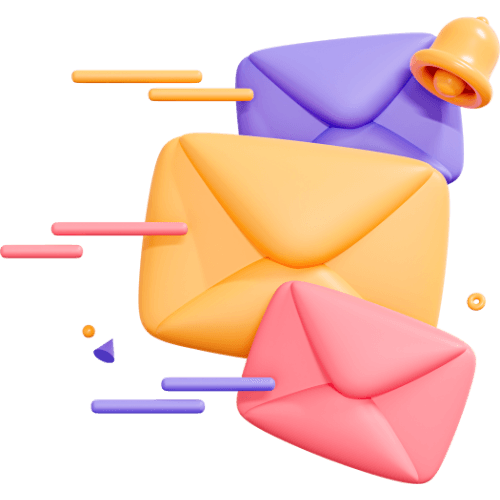
Related articles
9 Articles
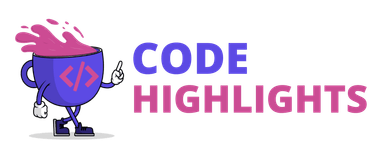
Copyright © Code Highlights 2025.