The Ultimate Guide to JavaScript Count Occurrences in Array Like a Pro

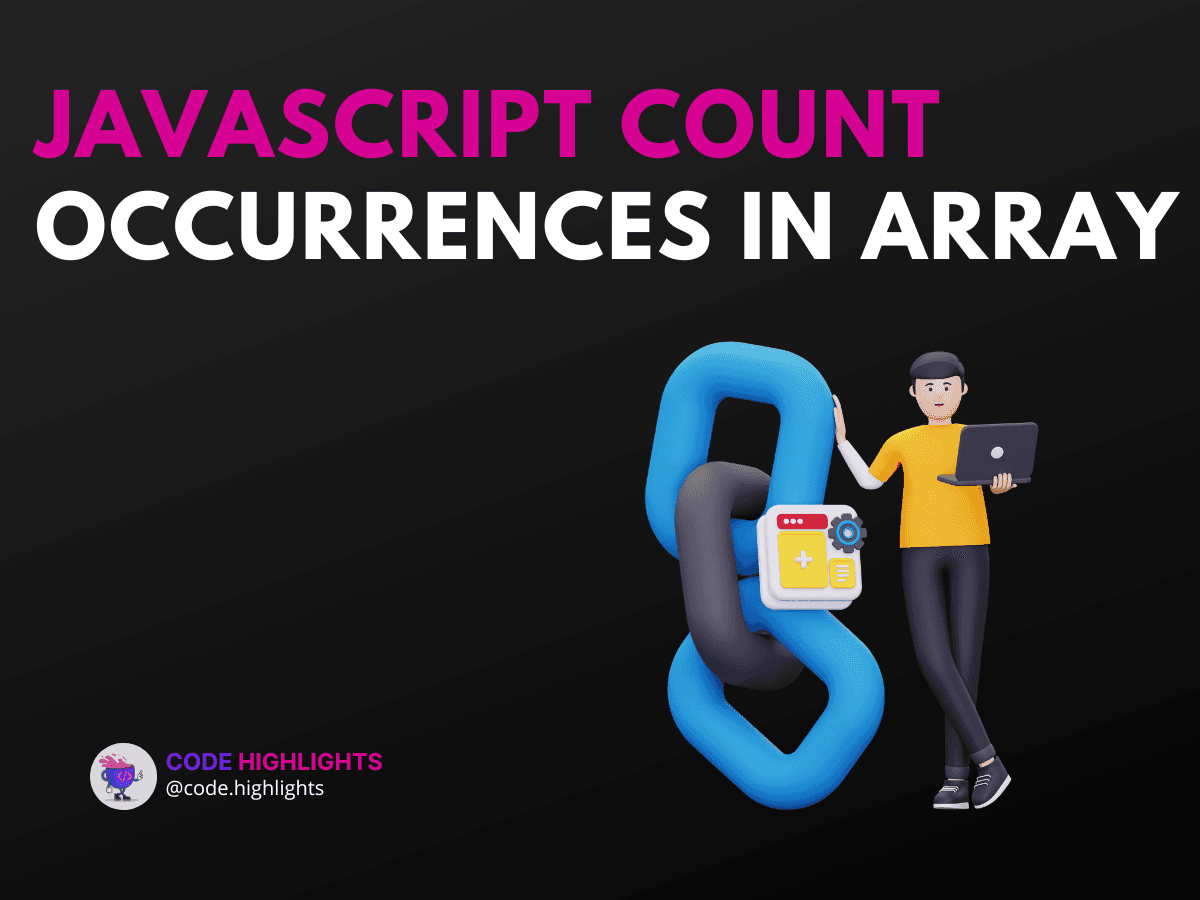
- Quick Code Example
- Understanding the Syntax
- Key Points:
- Variations of the Syntax
- Using a Loop
- Using Reduce
- Unique Use Cases
- Counting All Element Occurrences
- Counting Strings in an Array
- Browser Compatibility
- Summary
Counting occurrences in an array is a common task in programming. In JavaScript, this can be done easily and efficiently. Whether you want to find out how many times a specific element appears or get the count of all elements, understanding how to count occurrences in an array can enhance your coding skills. Let’s dive into this topic and explore different methods to achieve this.
Quick Code Example
Here’s a simple code snippet to get you started with counting occurrences in an array:
1const array = [1, 2, 2, 3, 4, 4, 4];
2const countOccurrences = (arr, value) => arr.filter(item => item === value).length;
3
4console.log(countOccurrences(array, 4)); // Output: 3
This example shows how to count the occurrences of the number 4
in the array.
Understanding the Syntax
The function countOccurrences
takes two parameters: arr
(the array) and value
(the element you want to count). It uses the .filter()
method to create a new array containing only the elements that match value
, and then returns the length of that array.
Key Points:
- Parameters:
arr
: An array of elements.value
: The element to count.
- Return Value: The number of times
value
appears inarr
.
Variations of the Syntax
You can also count occurrences using other methods. Here are a few variations:
Using a Loop
1const countWithLoop = (arr, value) => {
2 let count = 0;
3 for (let item of arr) {
4 if (item === value) {
5 count++;
6 }
7 }
8 return count;
9};
10
11console.log(countWithLoop(array, 2)); // Output: 2
Using Reduce
1const countWithReduce = (arr, value) => {
2 return arr.reduce((acc, item) => (item === value ? acc + 1 : acc), 0);
3};
4
5console.log(countWithReduce(array, 1)); // Output: 1
Unique Use Cases
Counting All Element Occurrences
If you want to count how many times each element appears in the array, you can use an object to store the counts:
1const countAllOccurrences = (arr) => {
2 return arr.reduce((acc, item) => {
3 acc[item] = (acc[item] || 0) + 1;
4 return acc;
5 }, {});
6};
7
8console.log(countAllOccurrences(array)); // Output: { '1': 1, '2': 2, '3': 1, '4': 3 }
Counting Strings in an Array
You can also count occurrences of strings within an array:
1const stringArray = ['apple', 'banana', 'apple', 'orange'];
2const countStringOccurrences = (arr, value) => arr.filter(item => item === value).length;
3
4console.log(countStringOccurrences(stringArray, 'apple')); // Output: 2
Browser Compatibility
JavaScript functions for counting occurrences work well across all major web browsers, including Chrome, Firefox, Safari, and Edge. Always ensure your browser is updated to the latest version to avoid any compatibility issues.
Summary
In this tutorial, we explored how to javascript count occurrences in array using various methods like filtering, loops, and reduce. We also covered counting all elements and specific types, such as strings. Mastering these techniques can significantly improve your JavaScript skills and allow you to handle data more effectively.
For further learning, consider checking out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. For those interested in a broader perspective, our Introduction to Web Development course is a great resource.
To learn more about counting occurrences in arrays, you can also refer to MDN Web Docs for detailed explanations and examples. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
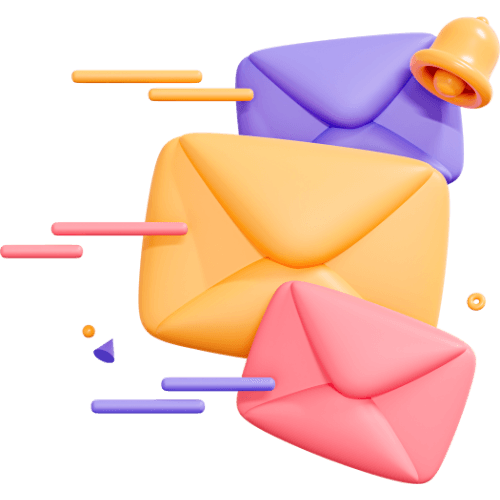
Related articles
9 Articles
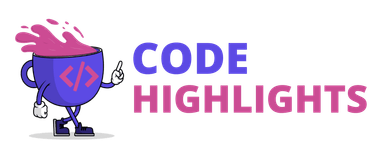
Copyright © Code Highlights 2025.