JavaScript Date Subtract Minutes: The Ultimate How-To

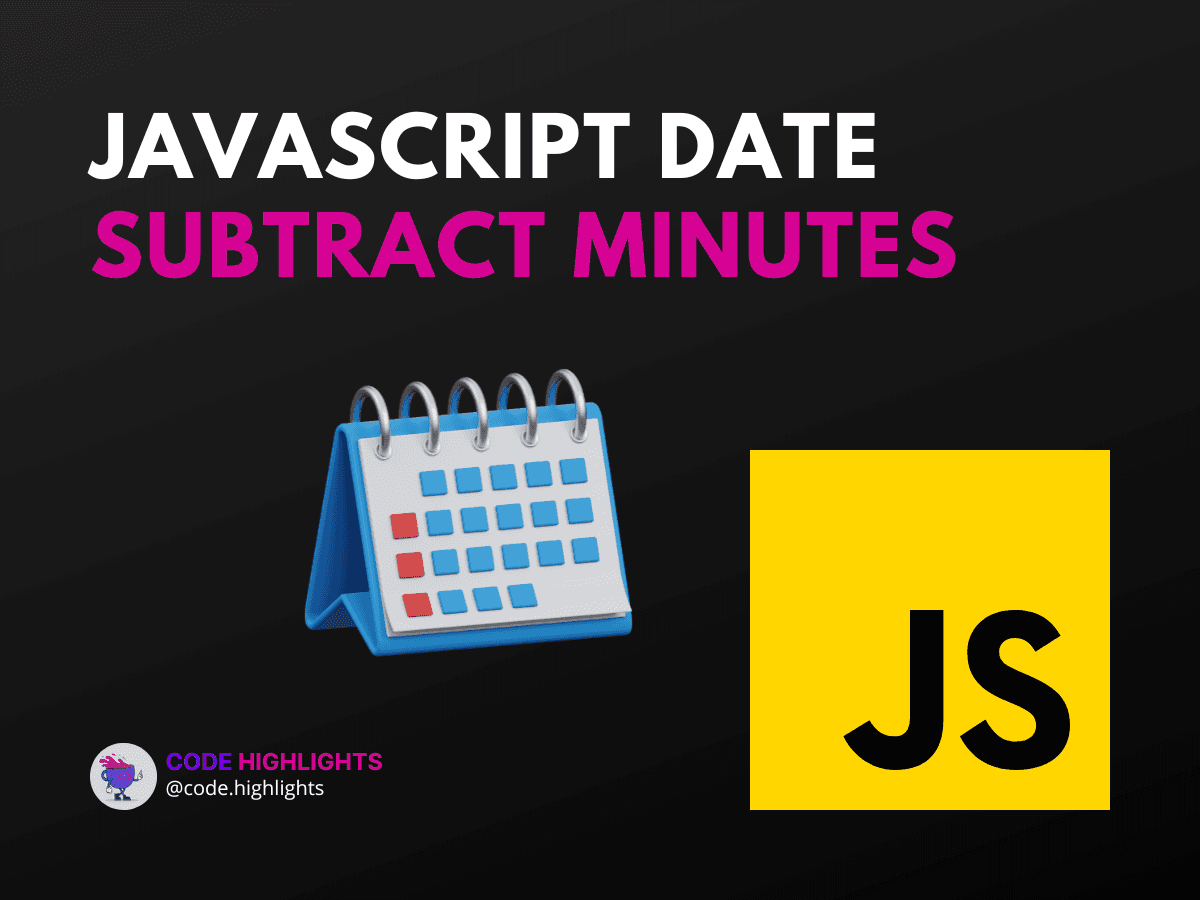
- Subtracting Minutes from a Date in JavaScript
- Extracting Minutes from a Date
- Adding Minutes to a Date
- Subtracting Hours from a Date
Welcome to our comprehensive guide on manipulating dates and times in JavaScript! Whether you're scheduling events, creating timers, or simply tracking time, understanding how to handle dates is a crucial skill for any web developer. In this tutorial, we'll dive into the specifics of how to subtract minutes from a JavaScript Date
object, along with other handy date manipulation techniques.
1let now = new Date();
2console.log("Current time:", now);
3now.setMinutes(now.getMinutes() - 1);
4console.log("One minute ago:", now);
The above snippet is just a teaser of what you can achieve with JavaScript's Date
object. By the end of this tutorial, you'll be comfortable performing various date-related operations with ease. Let's get started!
Subtracting Minutes from a Date in JavaScript
When working with dates, it's common to need to adjust the time by a certain number of minutes. Perhaps you're implementing a countdown timer or adjusting for time zone differences. Here's how to subtract 1 minute from a date in JavaScript:
This code takes the current date and time, retrieves the minutes, and then sets the minutes to one less than the current value. It's that simple!
Extracting Minutes from a Date
Sometimes, you may just want to extract the minutes from a date to display them or use them in calculations. Here's how you can do that:
1let currentTime = new Date();
2let minutes = currentTime.getMinutes();
3console.log("Minutes:", minutes);
By calling getMinutes()
on our Date
object, we can easily retrieve the current minutes. This method returns a value between 0 and 59, representing the minutes of the hour.
Adding Minutes to a Date
Adding minutes is just as straightforward as subtracting them. To add 2 minutes to a date in JavaScript, you can use the following code:
This will adjust the Date
object to represent a time exactly 2 minutes in the future from the original date.
Subtracting Hours from a Date
Subtracting hours follows the same principle. To subtract 5 hours from a date in JavaScript:
This modifies the Date
object to reflect a time 5 hours before the original date.
Throughout this tutorial, we've explored the versatility of the Date
object. If you're looking to deepen your JavaScript knowledge, consider checking out our JavaScript course. For those of you interested in the broader scope of web development, including HTML and CSS, our introduction to web development course is a fantastic resource.
Remember, practice makes perfect. Try implementing these methods in your projects and see how you can control time with a few lines of code! For more foundational knowledge, our HTML fundamentals course and CSS introduction are excellent starting points.
For further reading and best practices on JavaScript date manipulation, you might want to visit reputable sources such as MDN Web Docs, W3Schools, and Stack Overflow.
By mastering these techniques, you'll be well-equipped to handle any feature that requires date and time manipulation. Keep experimenting, keep learning, and most importantly, keep coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
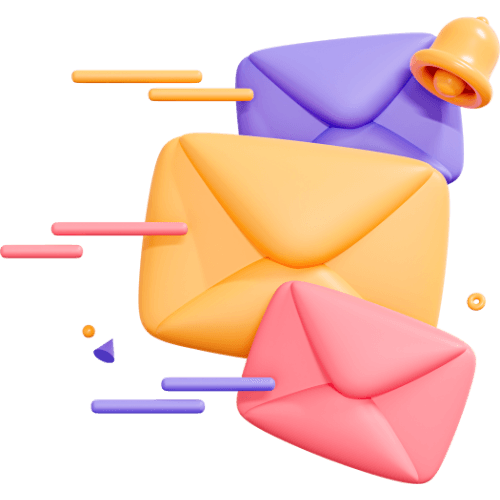
Related articles
9 Articles
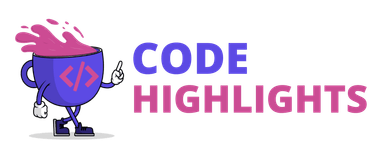
Copyright © Code Highlights 2025.