How to create a JavaScript Dictionary? [2023]

![How to create a JavaScript Dictionary? [2023] How to create a JavaScript Dictionary? [2023]](/_next/image?url=https%3A%2F%2Fstatic.code-hl.com%2Fresources%2Farticles%2Fmain%2Ftutorials-main.png&w=1200&q=75)
- Accessing Dictionary Values
- Searching a Dictionary
- Browser Compatibility
In JavaScript, objects are used to store keyed collections of various data and more complex entities. In simple words, objects allow us to create a single entity that stores data by key. A Javascript object is a lot like a dictionary.
Objects are created with figure brackets {…}
and should immediately be filled with properties. A property is a “key: value” pair. The key in this case would be the word and the value would be the definition.
Accessing Dictionary Values
To access the values of the dictionary, you can use the dot notation or the bracket notation.
1console.log(dictionary.Apple); // returns "A fruit"
2console.log(dictionary["Ball"]); // returns "A round object"
Searching a Dictionary
If you want to check if a property exists in a dictionary, you can use the hasOwnProperty()
method.
1console.log(dictionary.hasOwnProperty("Apple")); // returns true
2console.log(dictionary.hasOwnProperty("Cat")); // returns false
This method checks if the property exists in the dictionary and returns a Boolean value.
Browser Compatibility
All the methods mentioned in this tutorial work in all modern browsers, and IE6 and up. If you're new to JavaScript, check out our Introduction to Web Development and Learn JavaScript courses.
For further reading on JavaScript objects, Mozilla's JavaScript Object Basics is a great resource. If you want to dive deeper into the intricacies of object properties, this JavaScript.info tutorial covers it in detail.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
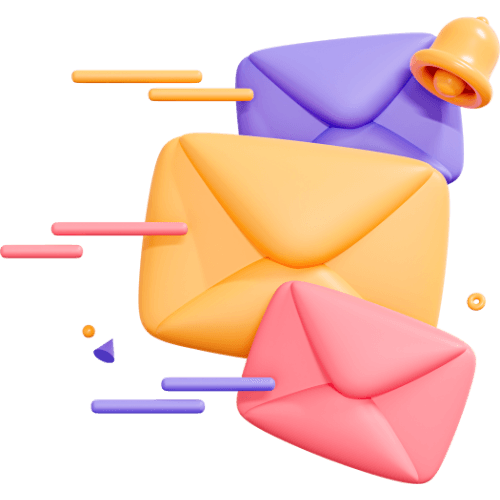
Related articles
9 Articles
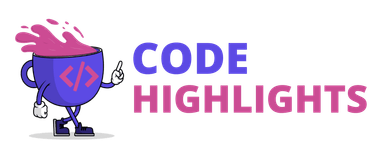
Copyright © Code Highlights 2025.