How to disable a button in JavaScript

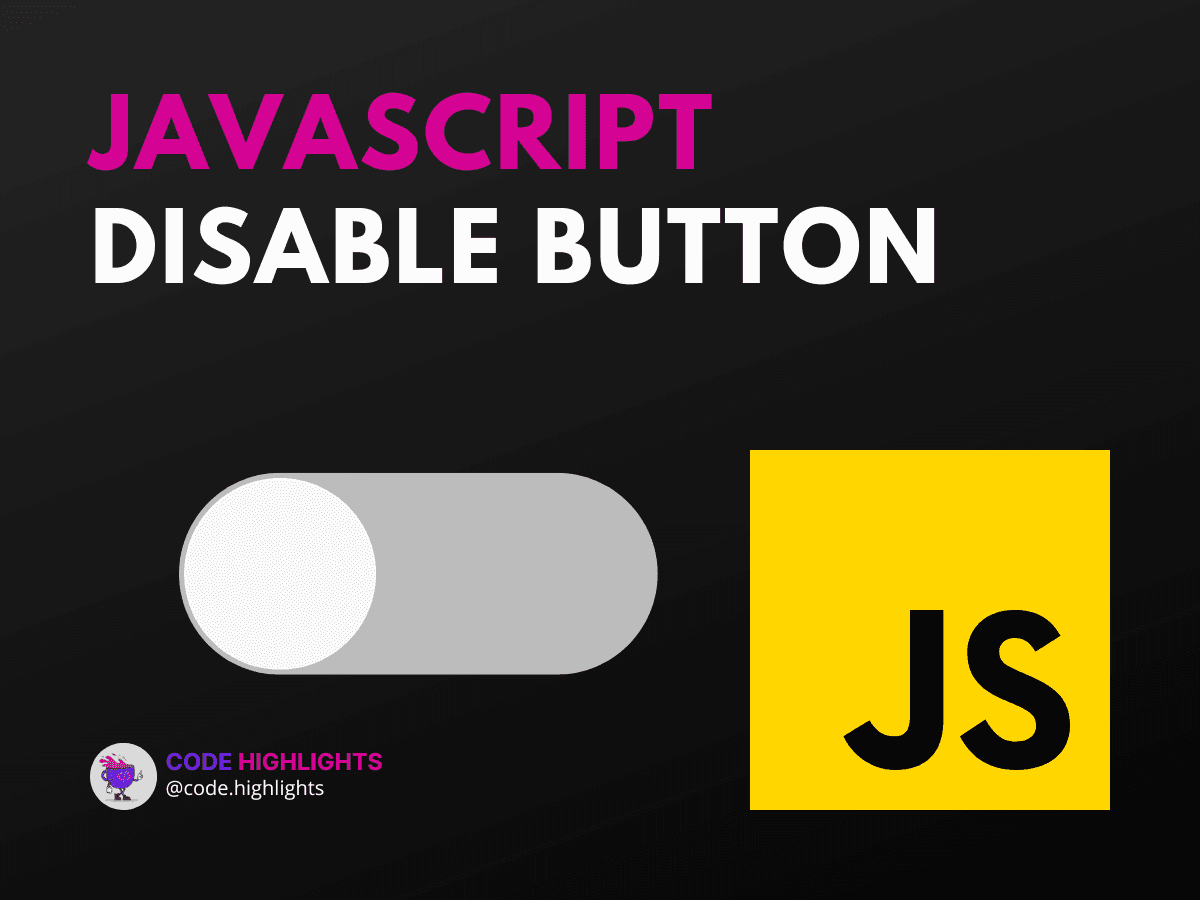
- Step-by-Step Guide to Disabling a Button
- 1. Selecting the Button Element
- 2. Disabling the Button
- 3. Conditional Disabling
- 4. Enabling a Disabled Button
- 5. Handling Multiple Buttons
- Common Questions Answered
- Enhancing Your Skills
- Conclusion
JavaScript is an essential tool in the web developer's toolkit, and one of its many uses is controlling the behavior of HTML elements on a webpage. Sometimes, you need to prevent users from clicking a button after it has been used, or under certain conditions. This tutorial will guide you through the process of disabling a button using JavaScript, ensuring that your web applications respond appropriately to user interactions.
Let's start with a simple example to illustrate the syntax:
1<button id="myButton" onclick="disableButton()">Click Me!</button>
2
3<script>
4function disableButton() {
5 document.getElementById('myButton').disabled = true;
6}
7</script>
In this snippet, we have a button that, when clicked, calls the disableButton
function. This function uses JavaScript to set the disabled
property of the button to true
, rendering it unclickable.
Step-by-Step Guide to Disabling a Button
1. Selecting the Button Element
To disable a button, you first need to select the HTML element. This can be done using various methods, but getElementById
is one of the most common and straightforward:
2. Disabling the Button
Once you have a reference to the button, you can set its disabled
attribute to true
:
This effectively disables the button, preventing any further clicks from triggering its associated events.
3. Conditional Disabling
You might want to disable a button only under certain conditions. For instance, you could disable a "Submit" button on a form until all fields are filled out correctly. Here's how you could do that:
1function checkForm() {
2 // Assume we have a function that checks the form validity
3 if (isFormValid()) {
4 document.getElementById('submitButton').disabled = false;
5 } else {
6 document.getElementById('submitButton').disabled = true;
7 }
8}
4. Enabling a Disabled Button
If you need to re-enable a button, simply set the disabled
attribute back to false
:
5. Handling Multiple Buttons
If your page has multiple buttons that need to be disabled, you can use querySelectorAll
to select them all and then loop through the NodeList to disable each one:
1var buttons = document.querySelectorAll('.disable-me');
2buttons.forEach(function(button) {
3 button.disabled = true;
4});
Common Questions Answered
-
How do you stop a button click in JavaScript? To stop a button click, you can remove the event listener or set the
disabled
property of the button totrue
. -
How do I make a button not clickable? Simply set the
disabled
attribute of the button totrue
. This can be done inline with HTML (<button disabled>
) or with JavaScript as shown above.
Enhancing Your Skills
Mastering the manipulation of DOM elements like buttons is just one aspect of becoming proficient in JavaScript. If you're looking to expand your knowledge, consider taking a JavaScript course or diving into HTML fundamentals and CSS introduction to round out your web development skills. And for a broader overview, explore what it takes to build websites from scratch with an introduction to web development.
Conclusion
Disabling a button in JavaScript is a simple yet powerful way to control user interactions on your website. By following the steps outlined in this tutorial, you can ensure that your buttons behave exactly as you intend, enhancing the usability and functionality of your web applications.
For further reading and best practices, check out these resources:
- Mozilla Developer Network (MDN) on the disabled attribute
- W3Schools JavaScript DOM Tutorial
- JavaScript.info for deep dives into JavaScript concepts
Remember, practice makes perfect. So, get coding and experiment with different scenarios where you might need to control the state of a button. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
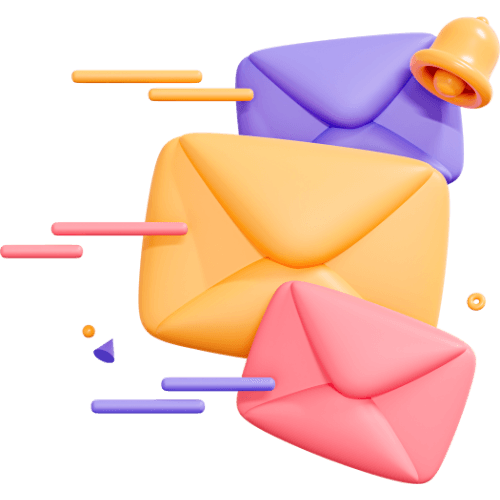
Related articles
9 Articles
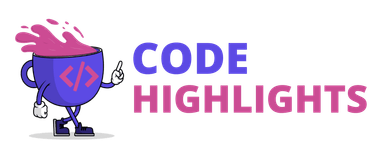
Copyright © Code Highlights 2025.