What is JavaScript double question mark (??) - nullish coalescing operator

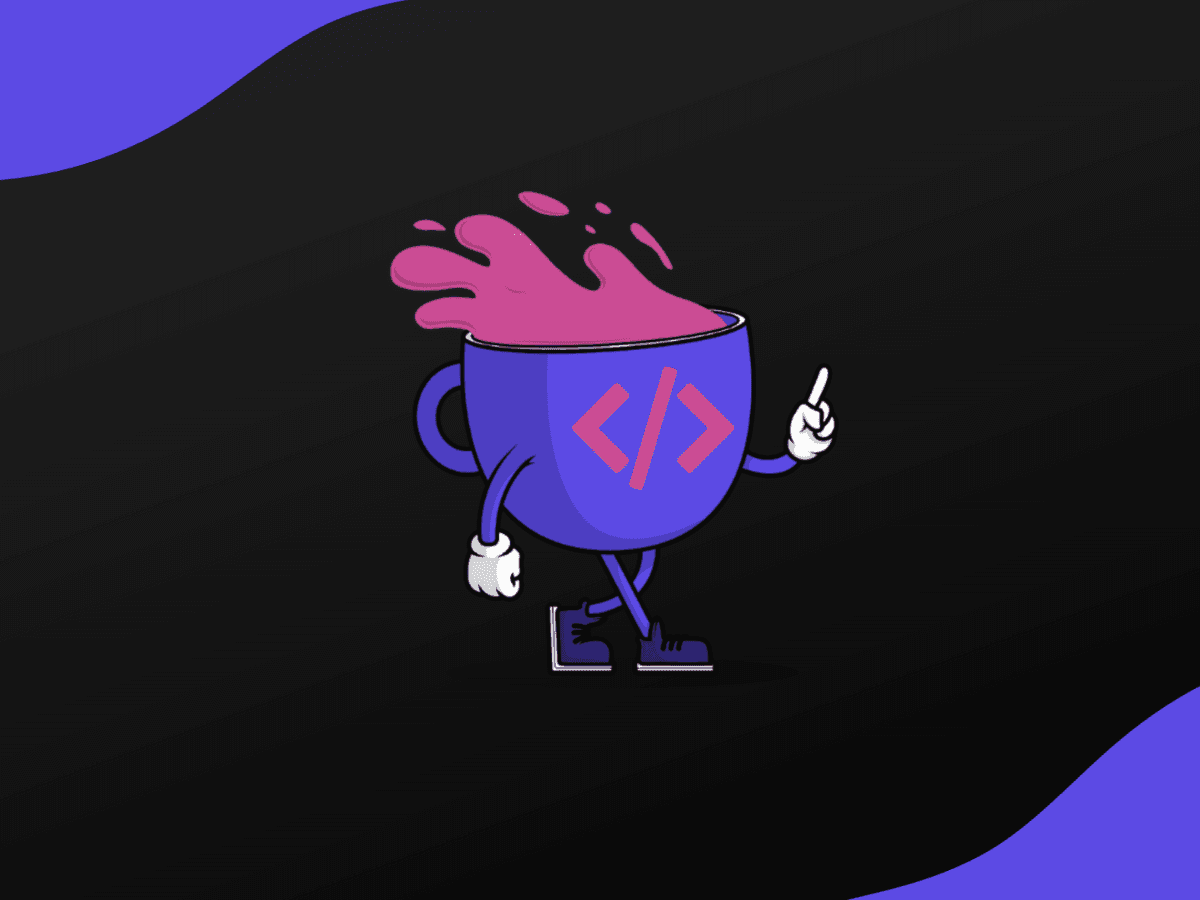
- Introduction to JavaScript Double Question Mark (??)
- Understanding the Nullish Coalescing Operator (??)
- Use Cases of Nullish Coalescing Operator (??)
- JavaScript Ternary Operator (?:)
- Browser Compatibility
Introduction to JavaScript Double Question Mark (??)
In JavaScript, the double question mark (??
) is a logical operator known as the Nullish Coalescing operator. This operator returns its right-hand side operand when its left-hand side operand is null
or undefined
, otherwise it returns the left-hand side operand.
1let value = null;
2let defaultValue = "Default Value";
3console.log(value ?? defaultValue); // Will log `Default Value`
Understanding the Nullish Coalescing Operator (??)
The Nullish Coalescing Operator (??
) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand. This can be contrasted with the logical OR (||
) operator, which returns the right-hand side operand if the left operand is any falsy value, not only null or undefined.
1let value = 0;
2console.log(value ?? "Default Value"); // Will log `0`
3console.log(value || "Default Value"); // Will log `Default Value`
In the first example, the ??
operator sees 0
as a valid value and therefore returns it. In the second example, the ||
operator sees 0
as a falsy value and therefore returns "Default Value"
.
Use Cases of Nullish Coalescing Operator (??)
The ??
operator is particularly useful when dealing with values that may be null
or undefined
. It allows for setting default values in a more intuitive way than using the ||
operator.
1let userSettings = {
2 theme: null,
3};
4
5let defaultSettings = {
6 theme: "Light",
7};
8
9console.log(userSettings.theme ?? defaultSettings.theme); // Will log `Light`
In this example, the user's theme preference is null
, so the ??
operator returns the default theme.
JavaScript Ternary Operator (?:)
The ternary operator (?:
) is another JavaScript operator that can be used for conditional programming. It takes three operands: a condition, a value to return if the condition is true, and a value to return if the condition is false.
In this example, if the value is greater than 0
, the string "Positive"
is logged. Otherwise, the string "Non-positive"
is logged.
Browser Compatibility
All modern browsers support the Nullish Coalescing Operator (??
). However, for Internet Explorer, a transpiler like Babel will be needed. You can learn more about JavaScript and its features in our JavaScript course.
FAQs
Can I use JavaScript Double Question Mark in all Browsers?
Can I use JavaScript Double Question Mark in all Browsers?
Yes, the double question mark operator is supported in all modern browsers. However, it may not be supported in older versions of Internet Explorer.
Can I Chain Multiple JavaScript Double Question Marks Together?
Can I Chain Multiple JavaScript Double Question Marks Together?
No, chaining multiple double question marks together is not supported and may result in a syntax error. The operator is intended to handle a single operand for nullish coalescing.
Is JavaScript Double Question Mark a Standard Feature in JavaScript?
Is JavaScript Double Question Mark a Standard Feature in JavaScript?
Yes, the double question mark operator is part of the ECMAScript 2020 specification, making it a standard feature in JavaScript.
What Happens if Both Expressions are Null or Undefined in JavaScript Double Question Mark?
What Happens if Both Expressions are Null or Undefined in JavaScript Double Question Mark?
If both expressions in the double question mark operator are null or undefined, the operator will return the value of the left-hand side expression.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
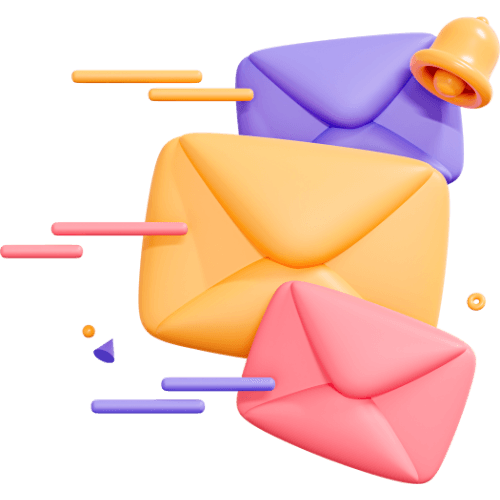
Related articles
125 Articles
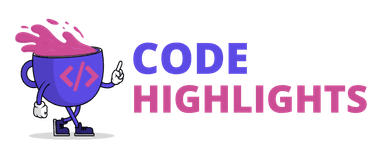
Copyright © Code Highlights 2024.