How to Use JavaScript Dynamic Variable Name for Flexibility

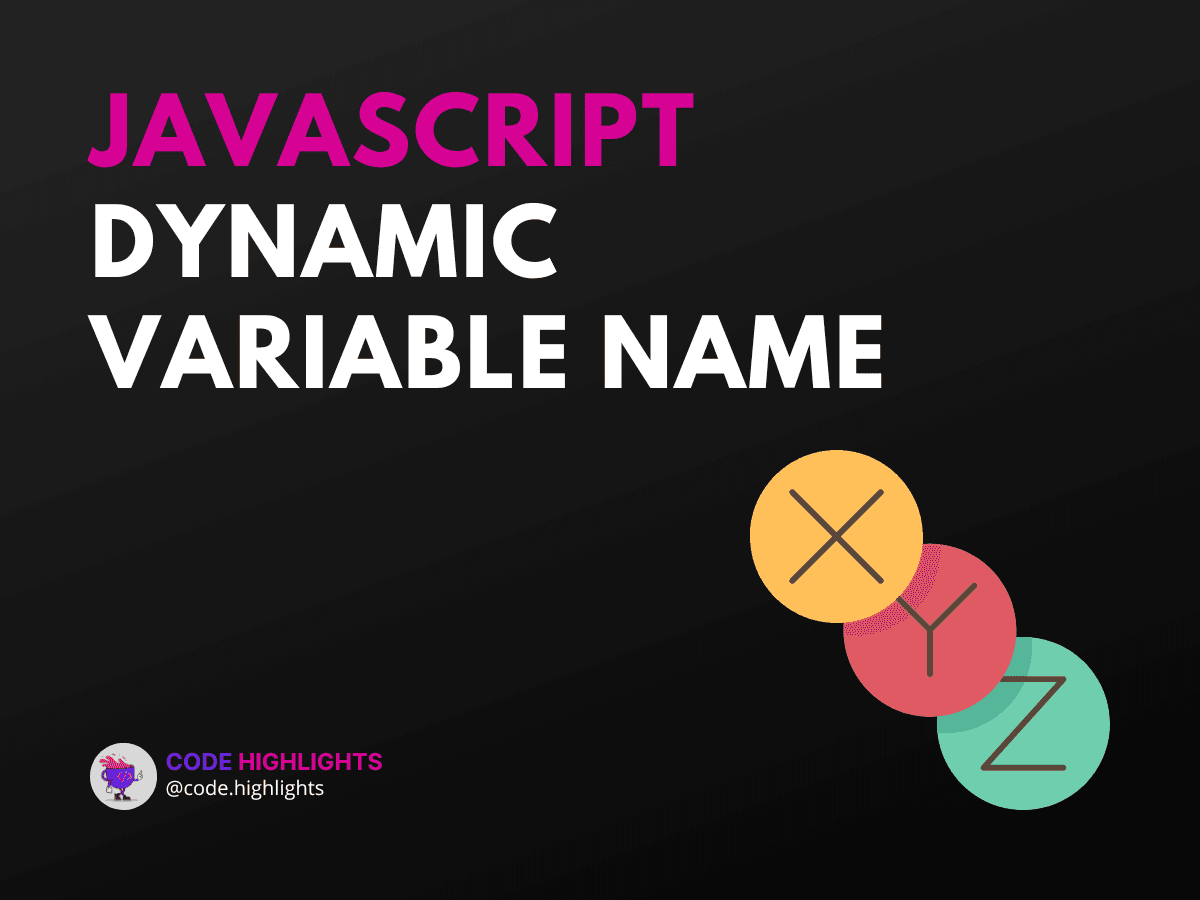
- Quick Code Example
- Understanding Syntax
- Syntax:
- Return Values
- Examples of Dynamic Variable Names
- Example 1: Setting Dynamic Variable Names
- Example 2: Passing Dynamic Object Names
- Example 3: Declaring a Dynamic Variable
- Example 4: Using Dynamic Function Names
- Browser Compatibility
- Summary
JavaScript is a powerful programming language that gives developers a lot of flexibility. One interesting feature is the ability to use dynamic variable names. This means you can create variable names on the fly, allowing your code to adapt to different situations easily. In this tutorial, we'll explore how to implement JavaScript dynamic variable names with practical examples.
Quick Code Example
Here’s a quick example to get you started:
1let dynamicVarName = "myVariable";
2window[dynamicVarName] = "Hello, World!";
3console.log(window.myVariable); // Outputs: Hello, World!
This snippet shows how to set a dynamic variable name using the window
object.
Understanding Syntax
In JavaScript, you can create dynamic variable names using bracket notation. This involves using an object (like window
or an ordinary object) and assigning a value to a property using a string as the key.
Syntax:
- object: The object where you want to create the dynamic variable.
- propertyName: A string representing the name of the variable you want to create.
- value: The data you want to store in that variable.
Return Values
The return value is the value assigned to that dynamic variable.
Examples of Dynamic Variable Names
Example 1: Setting Dynamic Variable Names
In this example, we create a dynamic variable name userAge
and assign it a value of 25
. This can be useful for storing user information without hardcoding variable names.
Example 2: Passing Dynamic Object Names
1function setDynamicProperty(obj, propName, value) {
2 obj[propName] = value;
3}
4
5let person = {};
6setDynamicProperty(person, "name", "Alice");
7console.log(person.name); // Outputs: Alice
Here, we define a function that sets a property on an object dynamically. This allows us to pass different property names when calling the function.
Example 3: Declaring a Dynamic Variable
1let varPrefix = "item";
2for (let i = 1; i <= 3; i++) {
3 window[varPrefix + i] = i * 10;
4}
5console.log(item1, item2, item3); // Outputs: 10 20 30
This example demonstrates how to declare multiple dynamic variables in a loop. Each variable is named item1
, item2
, and so on, with values assigned based on the loop index.
Example 4: Using Dynamic Function Names
1function sayHello() {
2 console.log("Hello!");
3}
4
5let funcName = "sayHello";
6window[funcName](); // Outputs: Hello!
In this case, we call a function using its name stored in a dynamic variable. This technique can help in situations where function names are determined at runtime.
Browser Compatibility
Most modern web browsers, including Chrome, Firefox, Safari, and Edge, support JavaScript dynamic variable names. However, it's always good to test your code across different environments to ensure compatibility.
Summary
In this tutorial, we explored how to use JavaScript dynamic variable names to add flexibility to your code. We covered the syntax, provided several examples, and discussed browser compatibility. Dynamic variable names can significantly enhance your programming capabilities, especially when dealing with varying data structures or user inputs.
If you want to dive deeper into JavaScript, check out our JavaScript course and learn more about the fundamentals of web development in our introduction to web development course.
By understanding how to declare and use dynamic variable names, you can create more adaptable and efficient code. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
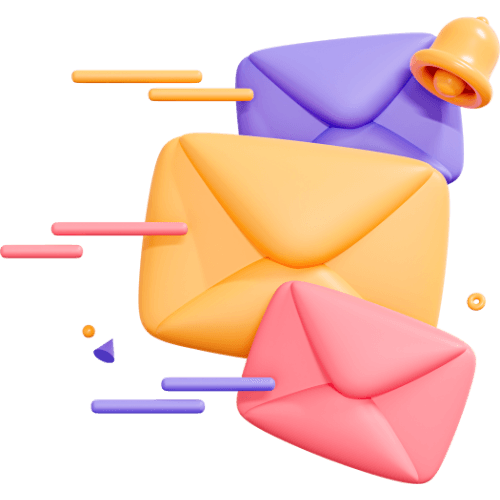
Related articles
9 Articles
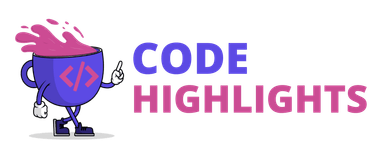
Copyright © Code Highlights 2025.