JavaScript Enums: Are They Essential for Developers?

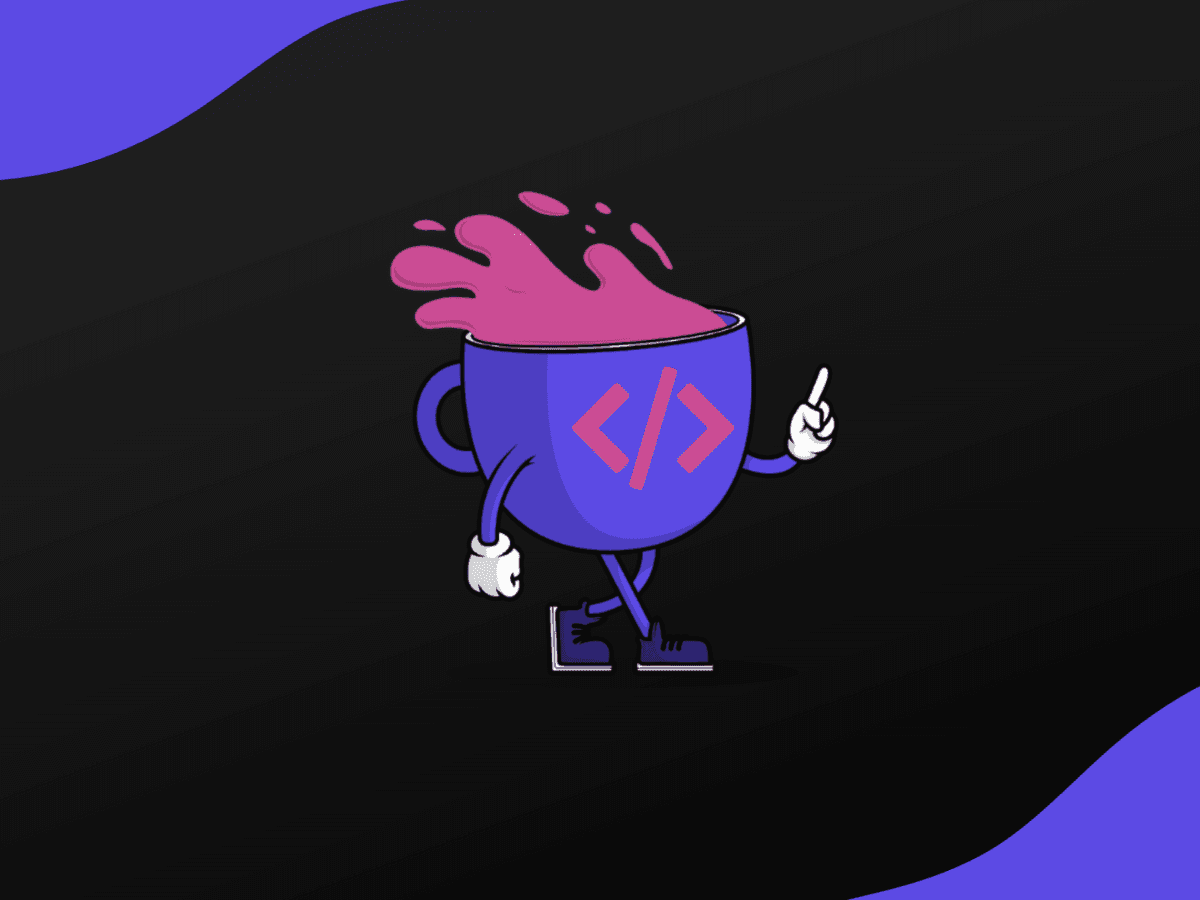
- Why Use Enums in JavaScript?
- Javascript Enums for readability
- Javascript Enums for code less prone to errors
- Create Javascipt Enums
- Implementing Javascript Enums Using Plain Objects
- Implementing Javascript Enums Using Object.freeze()
- Implementing Javascript Enums Using TypeScript
- Are Enums Essential?
- Browser Compatibility
- Conclusion
In JavaScript, Enums or Enumerations are a new data type that allows for a collection of related values that can be numeric or string values. However, JavaScript doesn't natively support enums. But with a bit of creativity, we can emulate enum-like behavior.
1const DaysOfWeek = {
2 MONDAY: "Monday",
3 TUESDAY: "Tuesday",
4 WEDNESDAY: "Wednesday",
5 // ... rest of the week
6};
This way, we've created an object that serves as an enum. Now let's delve into why and how to use them effectively.
Why Use Enums in JavaScript?
Enums help to reduce errors in your code by limiting the possible values for variables. They make your code more readable and maintainable.
Javascript Enums for readability
Imagine you're working on a project where you handle days of the week frequently. Instead of using strings everywhere, which can be prone to typos, you can use the enum DaysOfWeek
.
1function planMyWeek(day) {
2 if (day === DaysOfWeek.MONDAY) {
3 // Plan for Monday
4 }
5 // ... rest of the week
6}
Javascript Enums for code less prone to errors
They can make your code more readable and less error-prone.
1function validateDay(day) {
2 if (!Object.values(day).includes(day)) {
3 throw new Error("Invalid day");
4 }
5 // ...
6}
Create Javascipt Enums
Implementing Javascript Enums Using Plain Objects
Using an object is the easiest approach to create an enum in JavaScript.
1const DaysOfWeek = {
2 MONDAY: "Monday",
3 TUESDAY: "Tuesday",
4 WEDNESDAY: "Wednesday",
5 // ... rest of the week
6};
Implementing Javascript Enums Using Object.freeze()
Enums in JavaScript can be created using Object.freeze() to make them immutable.
1const DaysOfWeek = Object.freeze({
2 MONDAY: "Monday",
3 TUESDAY: "Tuesday",
4 // ... rest of the week
5});
Implementing Javascript Enums Using TypeScript
If you're using TypeScript, you have access to a true enum
type.
1enum DaysOfWeek {
2 MONDAY,
3 TUESDAY,
4 // ... rest of the week
5}
To learn more about it, checkout: TypeScript Enums.
Are Enums Essential?
While enums
can be helpful, they're not essential in JavaScript.
There are other ways to achieve similar functionality, such as using objects or sets.
Browser Compatibility
All methods mentioned in this tutorial work in all modern browsers.
Conclusion
While JavaScript doesn't natively support enums, they can be emulated using objects. Enums can help make your code more readable, maintainable, and less prone to errors.
For more JavaScript learning resources, visit our Learn JavaScript course.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
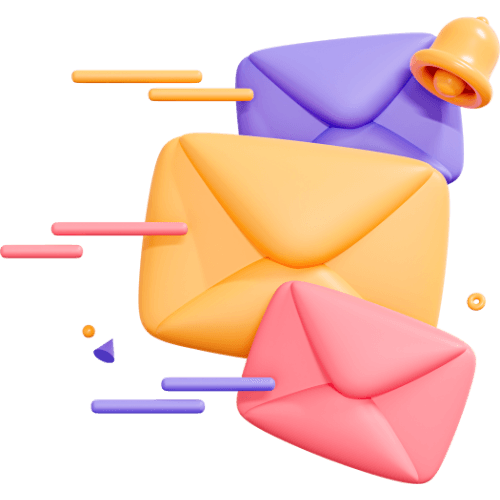
Related articles
9 Articles
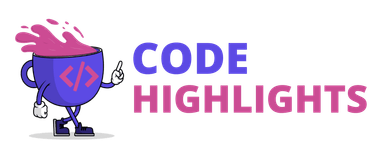
Copyright © Code Highlights 2025.