The Ultimate Guide to the Filter Method in JavaScript!

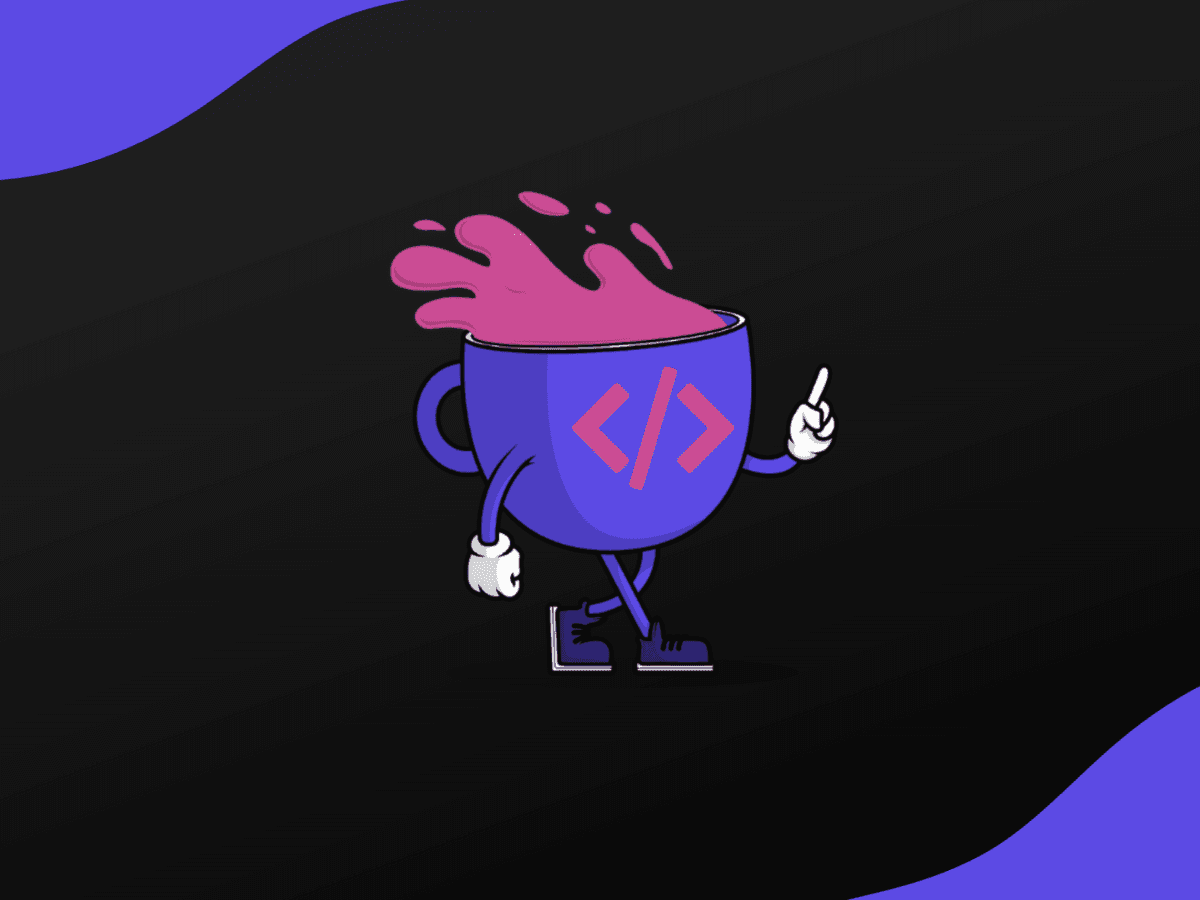
- How to Use filter() Method
- Filtering JavaScript Objects in an Array
- Filtering Two Arrays in JavaScript
- Browser Compatibility
In JavaScript, arrays are versatile data structures that can hold any type of data. They come with a variety of built-in methods for manipulation and traversal. One such powerful method is the filter()
method.
1const array = [1, 2, 3, 4, 5];
2const filteredArray = array.filter(num => num > 3);
3console.log(filteredArray); // Will log `[4, 5]`
The filter()
method creates a new array with all elements that pass the test implemented by the provided function. It's an elegant way to filter out elements from an array.
How to Use filter()
Method
The filter()
method is called on an array and takes a callback function as an argument. This callback function is executed for each element in the array and if the function returns true
, the current value is pushed to a new array. If the function returns false
, the element is discarded.
1const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
2const result = words.filter(word => word.length > 6);
3console.log(result); // Will log `["exuberant", "destruction", "present"]`
Filtering JavaScript Objects in an Array
The filter()
method can also be used to filter objects in an array. Let's say we have an array of objects representing a group of people. We can use the filter()
method to find all people who are above a certain age.
1const people = [
2 { name: 'John', age: 23 },
3 { name: 'Jane', age: 20 },
4 { name: 'Oliver', age: 32 },
5 { name: 'Emma', age: 30 }
6];
7
8const adults = people.filter(person => person.age > 21);
9console.log(adults); // Will log `[{ name: 'John', age: 23 }, { name: 'Oliver', age: 32 }, { name: 'Emma', age: 30 }]`
Filtering Two Arrays in JavaScript
To filter two arrays in JavaScript, you can use the filter()
method in combination with the includes()
method.
1const arr1 = [1, 2, 3, 4, 5];
2const arr2 = [0, 2, 4, 6, 8];
3const filtered = arr1.filter(num => arr2.includes(num));
4console.log(filtered); // Will log `[2, 4]`
Browser Compatibility
The filter()
method works in all modern browsers, and IE9 and up. If you found this post useful, you might also like my JavaScript Basics course, which features a ton of useful methods like these.
You can also refer to MDN Web Docs for more detailed information on the filter()
method.
This tutorial should give you a good understanding of how to use the filter method in JavaScript. Remember, the key to mastering JavaScript is practice. So, try to use these concepts in your projects as much as possible.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
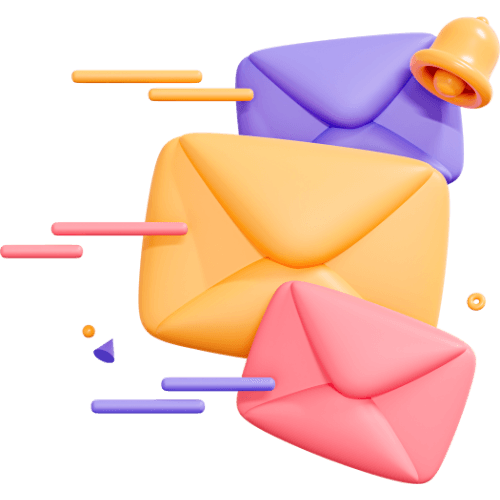
Related articles
9 Articles
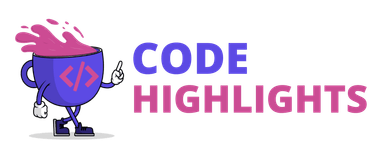
Copyright © Code Highlights 2025.