Ultimate Guide: Filter Objects by Key Value in JavaScript

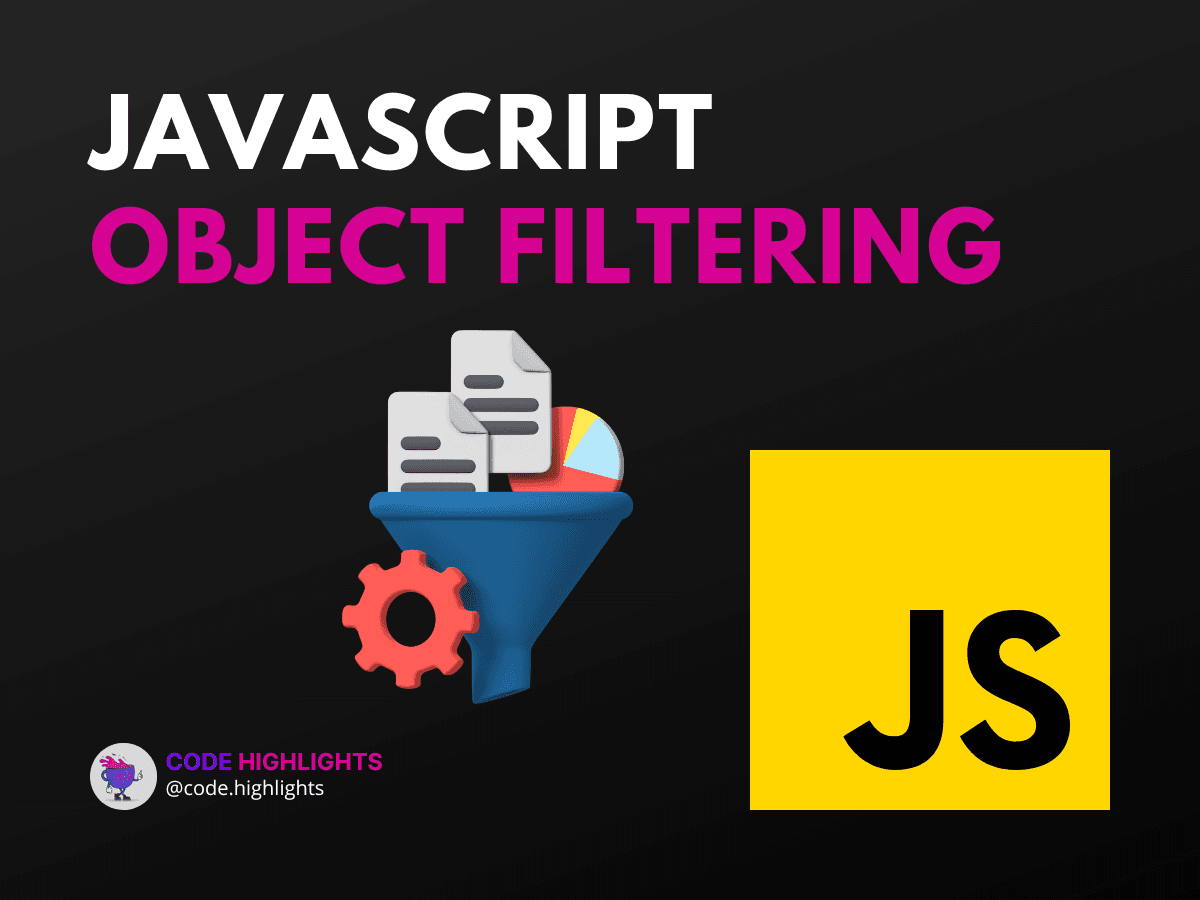
- Step-by-Step Tutorial
- Understanding Objects in JavaScript
- Converting Objects to Arrays
- Filtering the Array
- Reconstructing the Object
- Putting It All Together
- Best Practices and Tips
- Conclusion
JavaScript is a powerful language that allows you to manipulate data structures in various ways. One common task you might encounter is the need to filter an object by key value. Whether you're dealing with user inputs, API responses, or just organizing data, knowing how to filter objects effectively is a valuable skill in web development.
1let users = {
2 1: { name: 'Alice', age: 30 },
3 2: { name: 'Bob', age: 25 },
4 3: { name: 'Carol', age: 33 }
5};
6
7let filteredUsers = Object.fromEntries(
8 Object.entries(users).filter(([key, value]) => value.age > 30)
9);
10
11console.log(filteredUsers); // { "3": { "name": "Carol", "age": 33 } }
In this code snippet, we demonstrate how to filter an object's properties based on the age of the users. But how does this work? Let's dive into the steps and learn how to harness the power of JavaScript to filter objects by key value.
Step-by-Step Tutorial
Understanding Objects in JavaScript
Before we filter anything, it's important to understand what JavaScript objects are. If you're new to JavaScript, consider taking a JavaScript course to get up to speed.
Converting Objects to Arrays
To filter an object by key value, we first need to convert it to an array since objects do not have a filter method. We use Object.entries(obj)
which returns an array of a given object's own enumerable string-keyed property [key, value]
pairs.
Filtering the Array
With our array of key-value pairs, we can now use the filter()
method. This method creates a new array with all elements that pass the test implemented by the provided function.
1let ageCriteria = 30;
2let filteredEntries = entries.filter(([key, value]) => value.age > ageCriteria);
Reconstructing the Object
After filtering, we convert the array back into an object using Object.fromEntries(array)
.
Putting It All Together
Now, let's put all these steps together in a function that filters an object by key value:
1function filterObjectByValue(obj, key, value) {
2 return Object.fromEntries(
3 Object.entries(obj).filter(([k, v]) => v[key] === value)
4 );
5}
6
7let usersFilteredByName = filterObjectByValue(users, 'name', 'Alice');
8console.log(usersFilteredByName); // { "1": { "name": "Alice", "age": 30 } }
This function is versatile and can be used to filter objects by any key-value pair.
Best Practices and Tips
- Ensure your JavaScript knowledge is solid. Brush up on the basics with an HTML fundamentals course or a CSS introduction.
- Always validate and sanitize input if the filtering criteria come from user input to avoid potential security issues.
- Use meaningful variable names for better readability and maintainability of your code.
Conclusion
Filtering objects by key value is a task that may seem daunting at first, but with the right approach, it becomes a straightforward process. By converting the object to an array, applying the filter, and then reconstructing the object, you can achieve this with ease.
For those looking to deepen their understanding of web development, consider enrolling in an introduction to web development course.
Ready to take your JavaScript skills to the next level? Explore external resources for in-depth explanations and best practices:
Remember, practice makes perfect. Keep experimenting with different data sets and filtering criteria to become proficient at manipulating objects in JavaScript. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
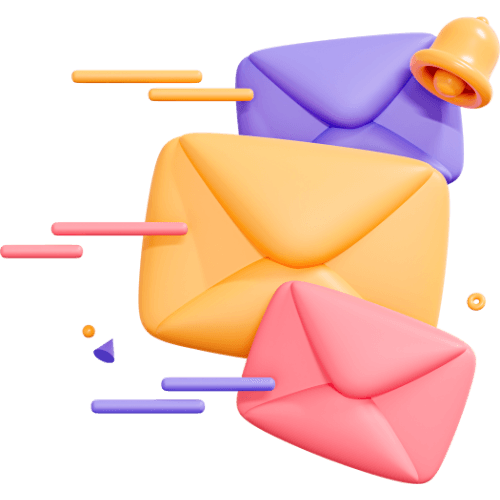
Related articles
114 Articles
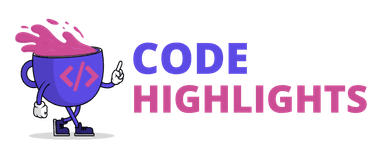
Copyright © Code Highlights 2024.