3 Quick Tips: Master JavaScript Trim Function!

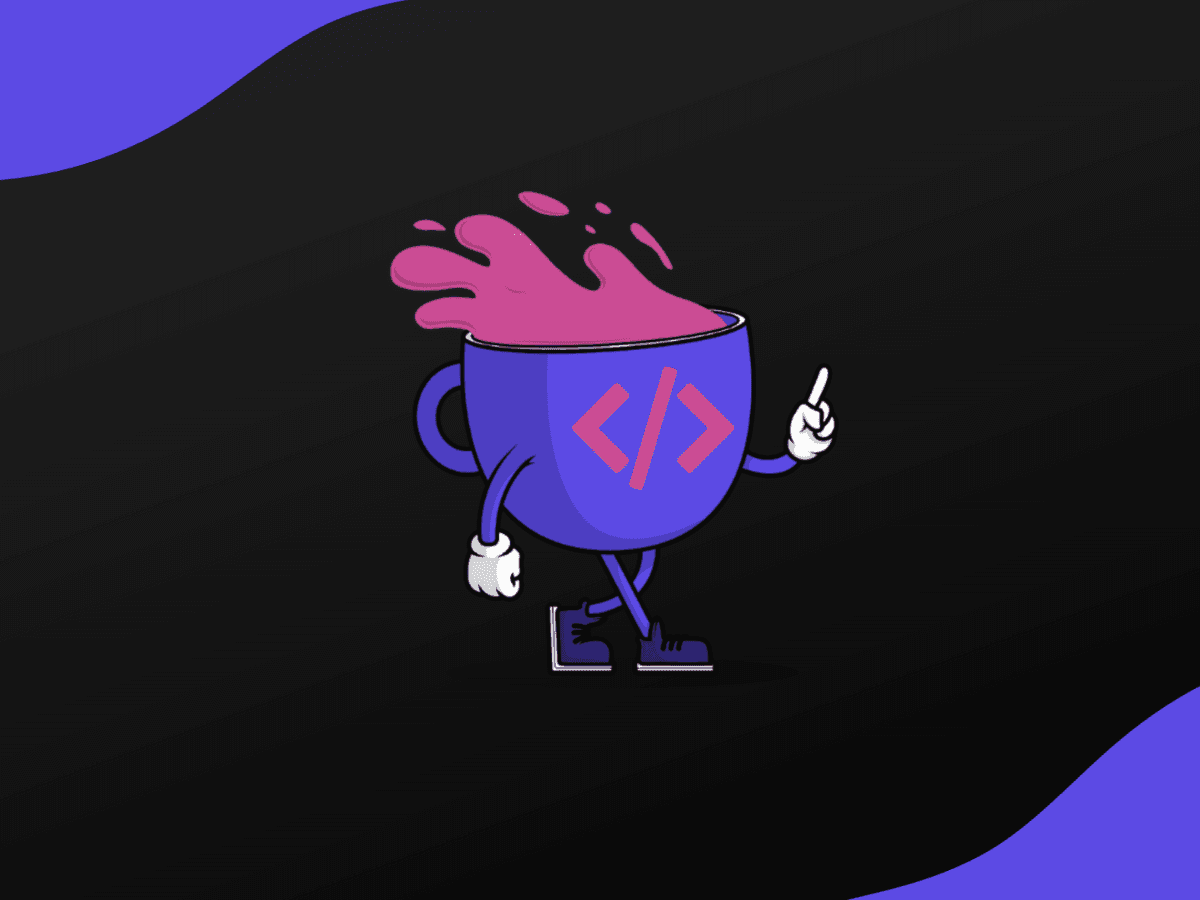
- Understanding the trim() Method
- Common Uses of trim()
- trimStart() and trimEnd()
- Browser Compatibility
In JavaScript, strings often contain unwanted whitespace at the start or end. This can occur when receiving user input, reading file data, or working with web APIs. The trim()
method is a built-in JavaScript function that removes these unnecessary spaces, making your data cleaner and more predictable.
Let's dive deeper into how the trim()
method works.
Understanding the trim()
Method
The trim()
method doesn't take any parameters, and it doesn't alter the original string. It creates a new string where the leading and trailing spaces have been removed.
1var str = " JavaScript trim tutorial ";
2console.log(str.trim()); // Returns "JavaScript trim tutorial"
3console.log(str); // Returns " JavaScript trim tutorial "
Common Uses of trim()
The trim()
method is commonly used to clean up user input when building forms. This prevents errors and inconsistencies in data storage and processing.
1var userInput = " javascript trim ";
2var cleanInput = userInput.trim();
3console.log(cleanInput); // Returns "javascript trim"
trimStart()
and trimEnd()
JavaScript also provides the trimStart()
and trimEnd()
methods to remove whitespace from only the beginning or end of a string.
1var str = " JavaScript trim tutorial ";
2console.log(str.trimStart()); // Returns "JavaScript trim tutorial "
3console.log(str.trimEnd()); // Returns " JavaScript trim tutorial"
Browser Compatibility
All modern browsers support the trim()
, trimStart()
, and trimEnd()
methods. For older browsers, you might need to use a polyfill.
If you found this tutorial helpful, check out our JavaScript course for more in-depth learning.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
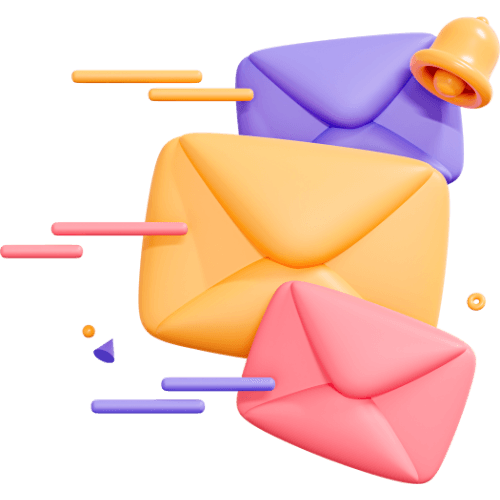
Related articles
9 Articles
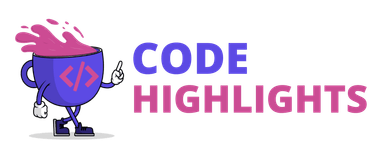
Copyright © Code Highlights 2025.