7 Essential JavaScript Find Methods to Use in 2024

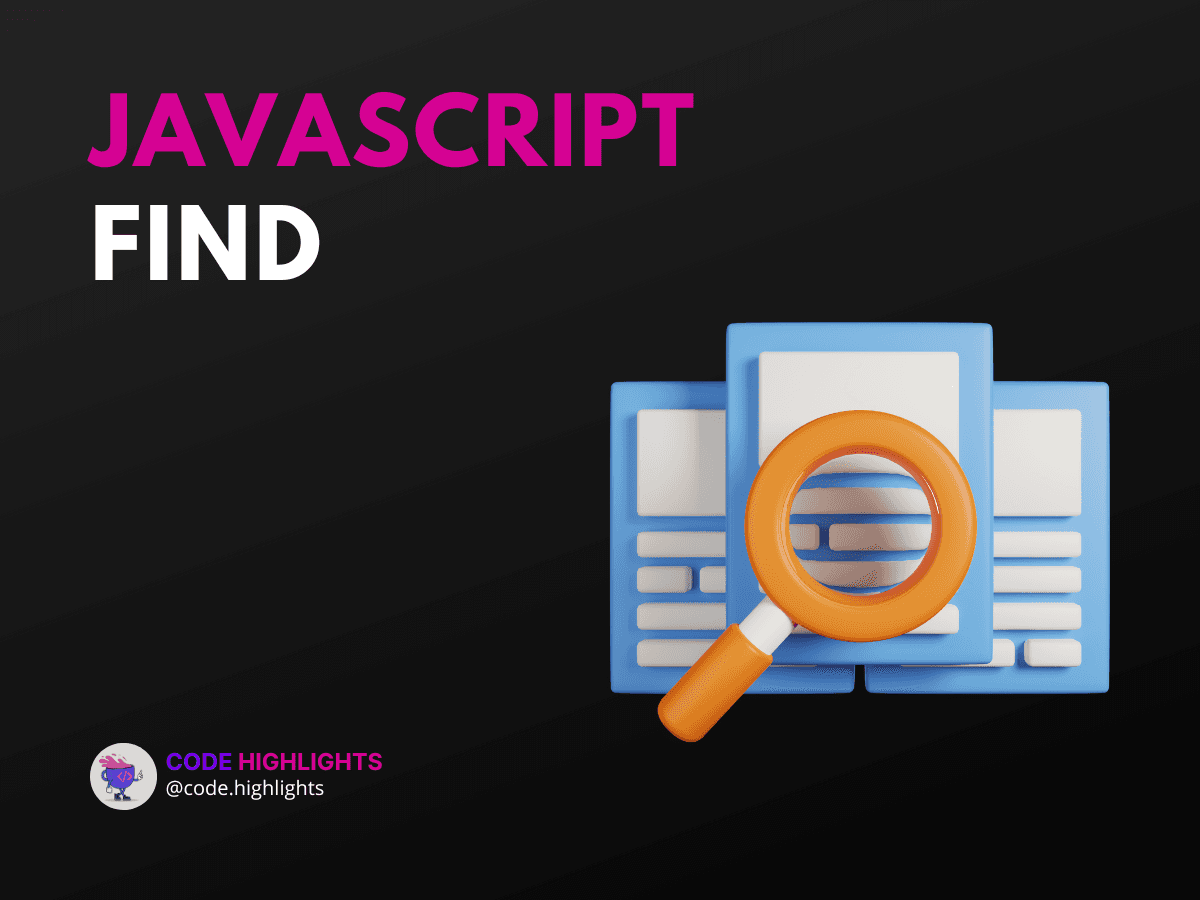
- Introduction to JavaScript Find Methods
- Syntax of JavaScript Find Methods
- Example 1: Finding an Object in an Array
- Example 2: Using Index Parameter
- Example 3: Using findIndex()
- Example 4: Combining find() with Other Methods
- Example 5: Finding in Nested Arrays
- Example 6: Using find() with Objects
- Example 7: Handling Undefined Values
- Compatibility with Major Web Browsers
- Summary
JavaScript is a versatile language with numerous methods to manipulate arrays. One such powerful method is find()
, which helps you locate elements in an array. In this tutorial, we will explore seven essential find
methods that are crucial for any developer in 2024.
Introduction to JavaScript Find Methods
The find()
method in JavaScript is used to search an array and return the first element that satisfies a given condition. Here's a quick example to get started:
1const numbers = [1, 2, 3, 4, 5];
2const found = numbers.find(element => element > 3);
3console.log(found); // Output: 4
In this example, the find()
method searches through the numbers
array and returns the first element greater than 3.
Syntax of JavaScript Find Methods
The basic syntax of the find()
method is straightforward:
callback
: A function that tests each element.element
: The current element being processed.index
(optional): The index of the current element.array
(optional): The arrayfind()
was called upon.thisArg
(optional): Value to use asthis
when executing the callback.
Example 1: Finding an Object in an Array
1const users = [
2 { id: 1, name: 'Alice' },
3 { id: 2, name: 'Bob' },
4 { id: 3, name: 'Charlie' }
5];
6const user = users.find(user => user.id === 2);
7console.log(user); // Output: { id: 2, name: 'Bob' }
Example 2: Using Index Parameter
1const numbers = [5, 12, 8, 130, 44];
2const foundIndex = numbers.find((element, index) => {
3 console.log(`Checking index ${index}`);
4 return element > 10;
5});
6console.log(foundIndex); // Output: 12
Example 3: Using findIndex()
The findIndex()
method returns the index of the first element that satisfies the condition.
1const fruits = ['apple', 'banana', 'mango', 'orange'];
2const index = fruits.findIndex(fruit => fruit === 'mango');
3console.log(index); // Output: 2
Example 4: Combining find() with Other Methods
You can combine find()
with other array methods like map()
or filter()
for more complex operations.
1const products = [
2 { name: 'Laptop', price: 1000 },
3 { name: 'Phone', price: 500 },
4 { name: 'Tablet', price: 700 }
5];
6const expensiveProduct = products.filter(product => product.price > 600).find(product => product.name === 'Tablet');
7console.log(expensiveProduct); // Output: { name: 'Tablet', price: 700 }
Example 5: Finding in Nested Arrays
1const nestedArray = [
2 [1, 2, 3],
3 [4, 5, 6],
4 [7, 8, 9]
5];
6const foundNested = nestedArray.find(subArray => subArray.includes(5));
7console.log(foundNested); // Output: [4, 5, 6]
Example 6: Using find() with Objects
1const inventory = [
2 { name: 'apples', quantity: 2 },
3 { name: 'bananas', quantity: 0 },
4 { name: 'cherries', quantity: 5 }
5];
6const result = inventory.find(item => item.quantity === 0);
7console.log(result); // Output: { name: 'bananas', quantity: 0 }
Example 7: Handling Undefined Values
If no element satisfies the condition, find()
returns undefined
.
1const numbers = [1, 2, 3, 4, 5];
2const notFound = numbers.find(element => element > 10);
3console.log(notFound); // Output: undefined
Compatibility with Major Web Browsers
The find()
method is well-supported across all major web browsers, including Chrome, Firefox, Safari, Edge, and Opera. This ensures that your code will run smoothly for most users.
Summary
In this tutorial, we explored the versatility of the JavaScript find()
method through various examples. From finding objects in arrays to handling nested arrays and combining with other methods, find()
is an indispensable tool for developers. For further learning, consider exploring our JavaScript course, HTML fundamentals course, and CSS introduction course.
For more detailed information, you can visit resources like MDN Web Docs and W3Schools.
By mastering these find
methods, you'll be well-equipped to handle array manipulations efficiently in your JavaScript projects in 2024 and beyond.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
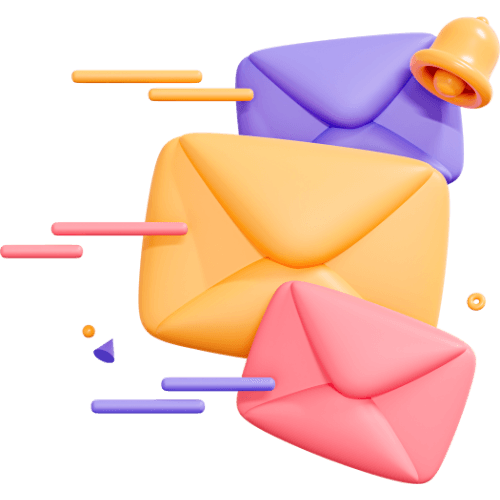
Related articles
9 Articles
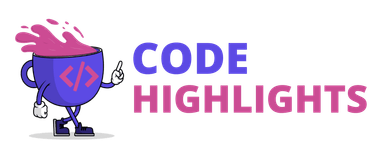
Copyright © Code Highlights 2024.