5 Quick Tips: JavaScript Find Index of Substring

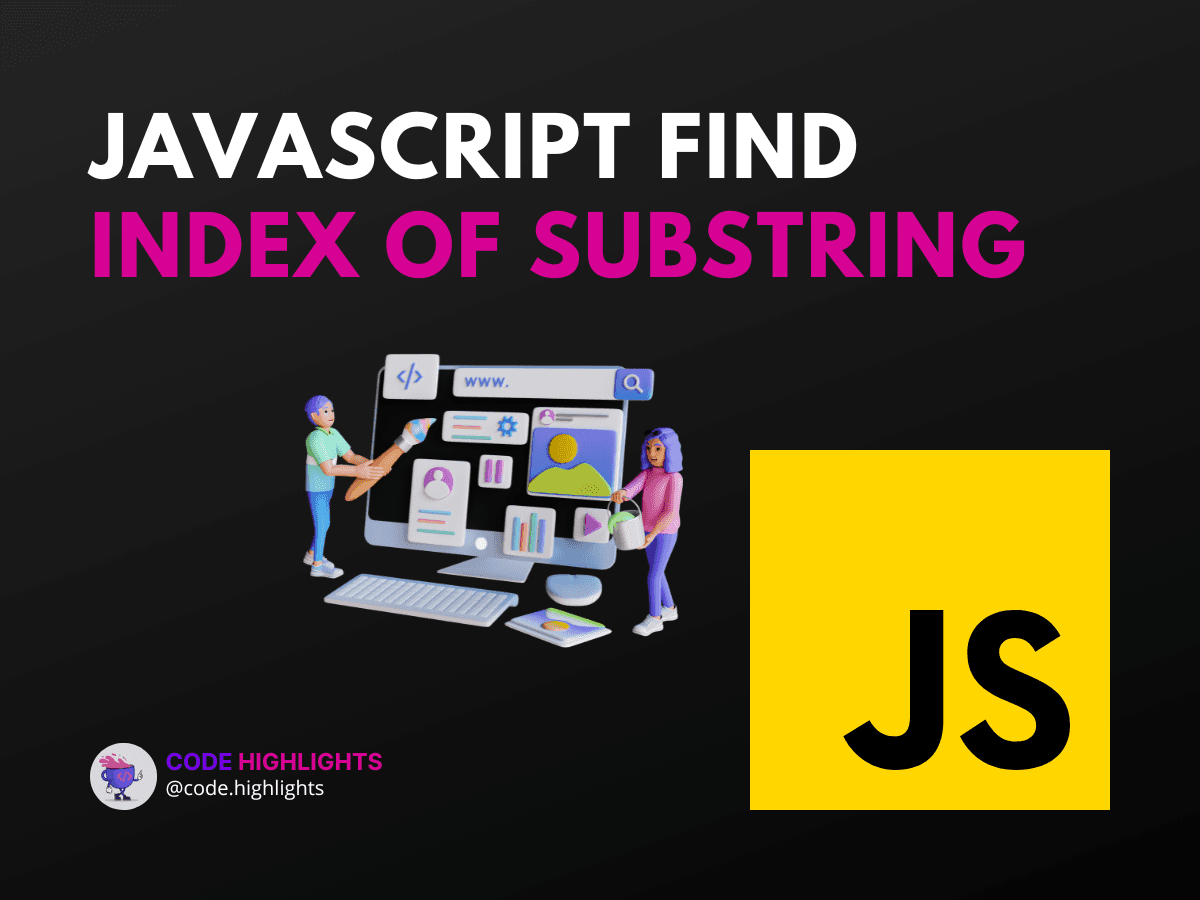
- Tip 1: Understand the Basics of Substring Indexing
- Tip 2: Finding a Substring Using indexOf()
- Tip 3: Get a Substring by Index Using slice()
- Tip 4: Searching for a Substring Using includes()
- Tip 5: Regular Expressions for Advanced Searches
Have you ever found yourself sifting through a haystack of text, looking for that elusive needle of a substring? In the world of web development, this scenario is all too common. Whether you're analyzing user input or processing text data, knowing how to locate a substring within a string is a fundamental skill in JavaScript. Let's dive into some quick tips that will help you master the art of pinpointing substrings with ease.
1// Introductory code example
2let str = "Hello, world!";
3let index = str.indexOf("world");
4console.log(index); // Output: 7
In the above snippet, we use the indexOf
method to find the starting position of the substring "world" within our string. Now, let's explore more ways and answer some burning questions on how to effectively search for substrings in JavaScript.
Tip 1: Understand the Basics of Substring Indexing
Before we delve deeper, it's essential to grasp what we mean by the index of a string substring. In JavaScript, strings are indexed with the first character starting at zero. When we search for a substring, we're looking for the starting index where this substring occurs.
For instance, if you want to know more about string manipulation and indexing, consider enrolling in a JavaScript course that covers these fundamentals.
Tip 2: Finding a Substring Using indexOf()
The indexOf()
method is your go-to function when you need to find the index of a substring in JavaScript. It returns the index of the first occurrence of a specified value or -1 if the substring is not found.
1let phrase = "Searching in strings is fun!";
2let searchTerm = "strings";
3let position = phrase.indexOf(searchTerm);
4console.log(position); // Output: 13
This method is case-sensitive, so ensure that your search term matches the case of the substring you're seeking.
Tip 3: Get a Substring by Index Using slice()
Once you have the index, you might want to extract the substring. The slice()
method comes in handy here. It takes two arguments: the starting index and the ending index (not included in the result).
1let text = "Don't forget to subscribe!";
2let start = text.indexOf("subscribe");
3let end = start + "subscribe".length;
4let extracted = text.slice(start, end);
5console.log(extracted); // Output: subscribe
In the example, we get the substring in JavaScript by index, which allows us to isolate the word "subscribe" from the rest of the text.
Tip 4: Searching for a Substring Using includes()
If you're less concerned about the index and more about the presence of a substring, includes()
is what you need. It checks if a string contains a specified substring and returns true or false.
1let sentence = "Is there a match?";
2let wordToFind = "match";
3let result = sentence.includes(wordToFind);
4console.log(result); // Output: true
This method simplifies the process when you're searching for a substring in JavaScript without needing the exact index.
Tip 5: Regular Expressions for Advanced Searches
For more complex search patterns, regular expressions (regex) are extremely powerful. They allow you to search for patterns within strings, which can include specific characters, ranges, or sequences.
1let data = "Error: Not found at index 42.";
2let regexPattern = /\bindex \d+/;
3let match = data.match(regexPattern);
4console.log(match[0]); // Output: index 42
Regular expressions can be daunting at first, but they're invaluable for tasks that go beyond simple substring searches.
In conclusion, mastering the ways to find and manipulate substrings in JavaScript opens up a myriad of possibilities for string processing. From simple searches with indexOf()
to advanced pattern matching with regex, JavaScript provides a rich set of tools for dealing with text.
To further enhance your skills, check out resources like MDN Web Docs for in-depth explanations and W3Schools for practical examples. And remember, practice makes perfect—so keep experimenting with these methods to become a JavaScript substring-finding ninja!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
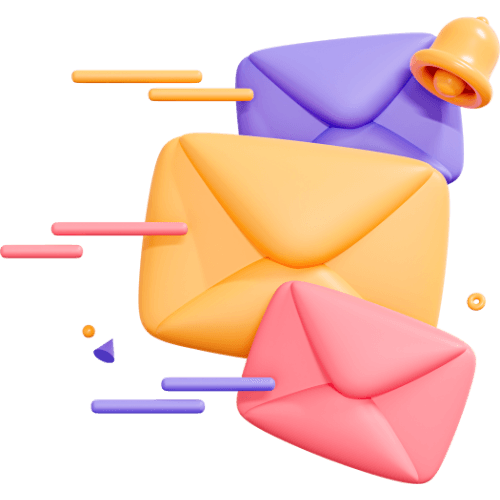
Related articles
9 Articles
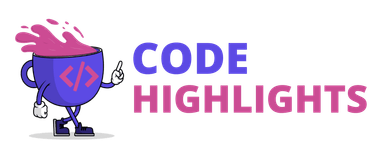
Copyright © Code Highlights 2025.