5 Easy Ways to Convert JavaScript Float to Int

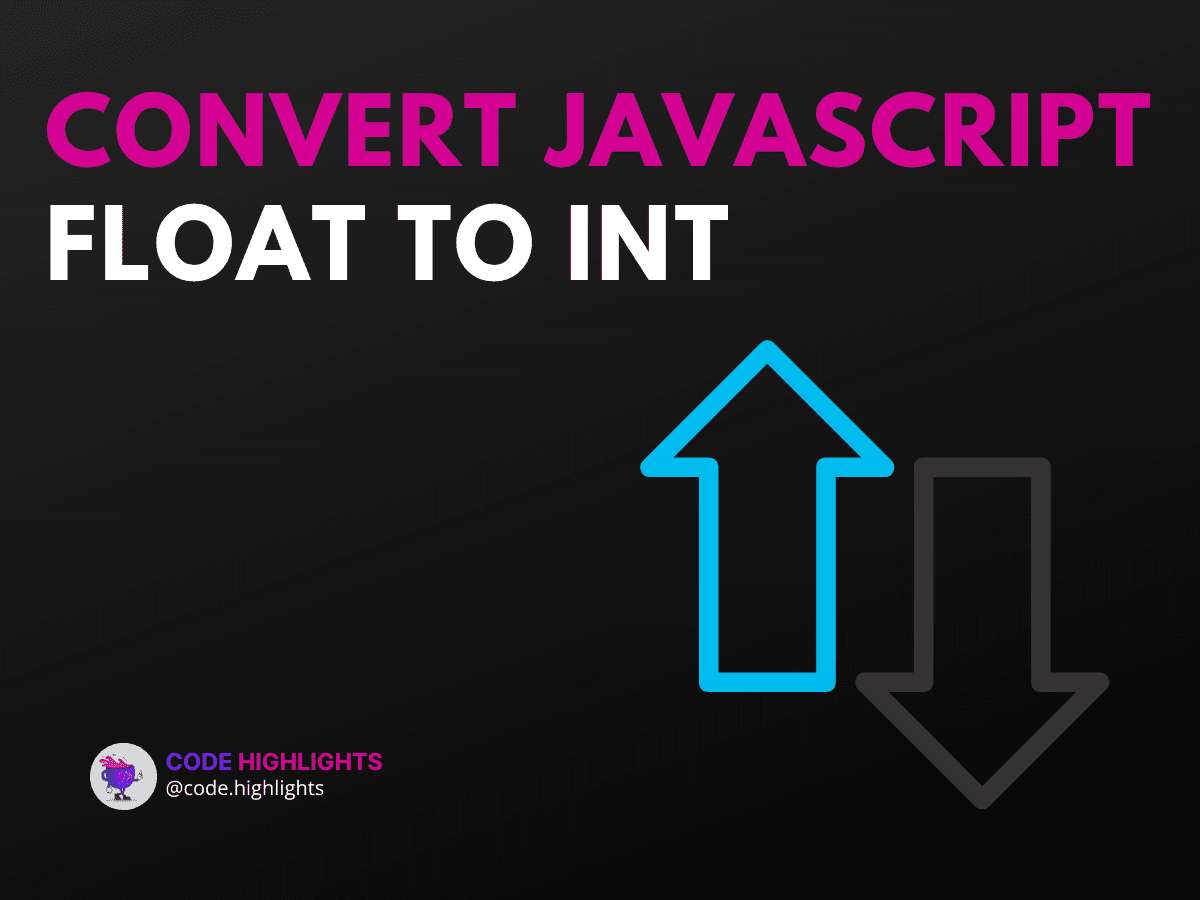
- Introduction Code Example
- Method 1: Using Math.floor()
- Syntax
- Example
- Method 2: Using Math.ceil()
- Syntax
- Example
- Method 3: Using Math.round()
- Syntax
- Example
- Method 4: Using parseInt()
- Syntax
- Example
- Method 5: Using Bitwise OR Operator (|)
- Syntax
- Example
- Compatibility
- Summary
Converting a float to an integer in JavaScript is a common task that many developers encounter. Whether you're dealing with user input, calculations, or data processing, understanding how to handle this conversion can simplify your code and improve its efficiency. Let's dive into five straightforward methods to achieve this.
Introduction Code Example
1let floatNumber = 4.56;
2let intNumber = Math.floor(floatNumber);
3console.log(intNumber); // Output: 4
Method 1: Using Math.floor()
The Math.floor()
function rounds a number down to the nearest integer.
Syntax
- Parameters:
x
(the number to be rounded down) - Return Value: The largest integer less than or equal to
x
Example
1let floatNumber = 7.89;
2let intNumber = Math.floor(floatNumber);
3console.log(intNumber); // Output: 7
Method 2: Using Math.ceil()
The Math.ceil()
function rounds a number up to the nearest integer.
Syntax
- Parameters:
x
(the number to be rounded up) - Return Value: The smallest integer greater than or equal to
x
Example
1let floatNumber = 7.89;
2let intNumber = Math.ceil(floatNumber);
3console.log(intNumber); // Output: 8
Method 3: Using Math.round()
The Math.round()
function rounds a number to the nearest integer.
Syntax
- Parameters:
x
(the number to be rounded) - Return Value: The value of
x
rounded to the nearest integer
Example
1let floatNumber = 7.49;
2let intNumber = Math.round(floatNumber);
3console.log(intNumber); // Output: 7
Method 4: Using parseInt()
The parseInt()
function parses a string argument and returns an integer of the specified radix (base).
Syntax
- Parameters:
string
(the value to parse)radix
(an integer between 2 and 36 that represents the base of the numeral system)
Example
1let floatNumber = "7.89";
2let intNumber = parseInt(floatNumber);
3console.log(intNumber); // Output: 7
Method 5: Using Bitwise OR Operator (|
)
The bitwise OR operator can also convert a float to an integer by truncating the decimal part.
Syntax
- Parameters:
x
(the number to be converted) - Return Value: The integer part of
x
Example
Compatibility
All these methods are compatible with major web browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer. This ensures that your code will run smoothly across different environments.
Summary
In this tutorial, we explored five simple methods to convert a JavaScript float to an int:
- Math.floor(): Rounds down to the nearest integer.
- Math.ceil(): Rounds up to the nearest integer.
- Math.round(): Rounds to the nearest integer.
- parseInt(): Parses a string and returns an integer.
- Bitwise OR (
|
): Truncates the decimal part.
Understanding these methods helps in handling numerical data more effectively. For further learning, consider exploring our JavaScript course or our Introduction to Web Development course.
For more detailed information on JavaScript number conversions, you can refer to this MDN Web Docs article.
By mastering these techniques, you'll be better equipped to manage numerical data and enhance your coding skills. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
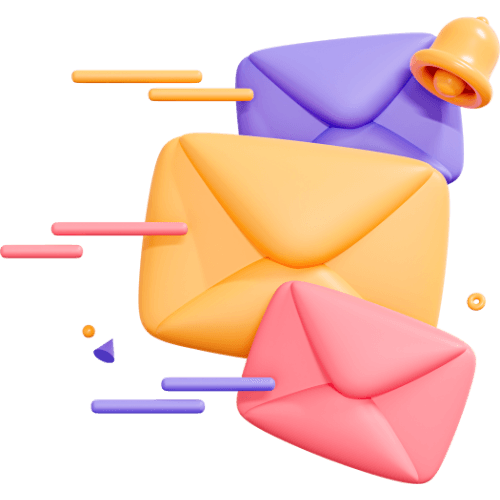
Related articles
9 Articles
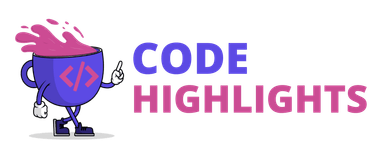
Copyright © Code Highlights 2024.