7 Pro Tips for Mastering JavaScript Floats

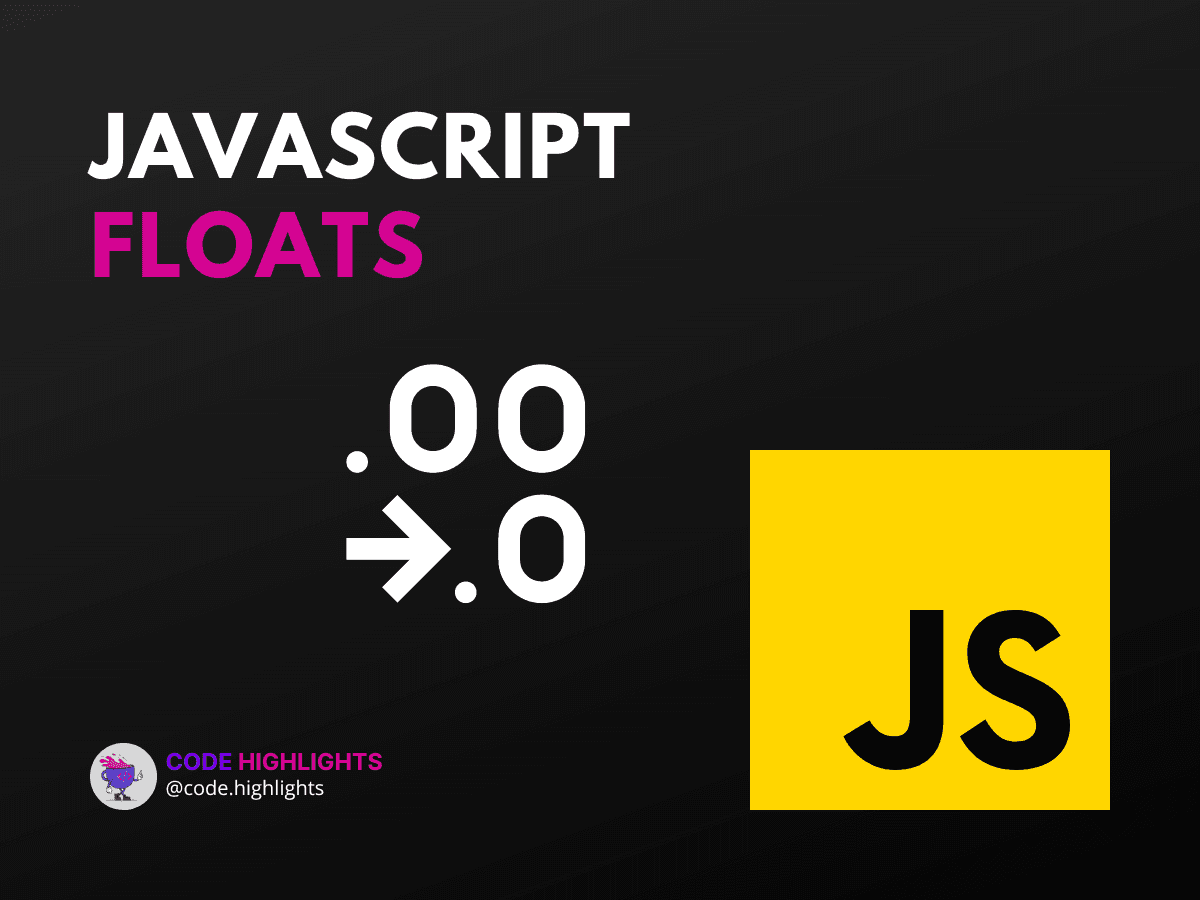
- 1. Allowing Float Numbers in JavaScript
- 2. Understanding JavaScript Number Types
- 3. How JavaScript Stores Floats
- 4. Converting to Floats in JavaScript
- 5. Handling Precision with toFixed()
- 6. Avoiding Common Pitfalls
- 7. Libraries Can Help
JavaScript, the backbone of web development, is a language full of nuances, especially when it comes to handling different data types. One area that often perplexes new developers is dealing with numbers—more specifically, javascript floats
. In this tutorial, you'll learn essential tips to master floating-point numbers in JavaScript, ensuring your calculations are accurate and efficient.
Let's dive right into an example that demonstrates how JavaScript handles decimal numbers:
As you can see, the syntax for representing floats is straightforward. Now, let's explore some pro tips to help you work with these numbers like a seasoned developer.
1. Allowing Float Numbers in JavaScript
When working with decimals, JavaScript doesn't differentiate between ints
and floats
as some other languages do. Instead, all numbers are treated as floating-point numbers under the IEEE 754 standard. To allow float numbers, simply include a decimal point:
This flexibility means you don't need to declare a number type explicitly, but it also requires attention to precision and rounding.
2. Understanding JavaScript Number Types
In JavaScript, there aren't separate ints
and floats
; every number is a double-precision 64-bit binary format IEEE 754 value (a "double"). This means that both integers and non-integer numbers are stored in the same way, which can lead to precision issues with very large or very small numbers.
3. How JavaScript Stores Floats
JavaScript stores numbers as 64-bit floating-point format, which allocates 1 bit for the sign, 11 bits for the exponent, and 52 bits for the mantissa. This representation allows for a vast range of values but also introduces some quirks, such as the famous 0.1 + 0.2 !== 0.3
scenario due to binary rounding errors.
4. Converting to Floats in JavaScript
To ensure a value is treated as a float, you can use the parseFloat()
function:
1let stringValue = "24.987";
2let floatValue = parseFloat(stringValue);
3console.log(floatValue); // Output: 24.987
This function parses a string and returns a floating-point number. It's particularly useful when you receive numeric values as strings, such as from user input or APIs.
5. Handling Precision with toFixed()
Dealing with floating-point arithmetic can result in long decimal numbers. To limit the number of decimal places, use the toFixed()
method:
This method converts a number into a string, rounding to the specified number of decimal places.
6. Avoiding Common Pitfalls
Beware of the imprecision that can arise from floating-point arithmetic. For instance, comparing floats for equality might not always yield the expected results. Instead, define a tolerance level for comparison:
1function areFloatsEqual(float1, float2, tolerance = 0.001) {
2 return Math.abs(float1 - float2) < tolerance;
3}
7. Libraries Can Help
For complex mathematical operations, consider using libraries like math.js
or decimal.js
, which provide more robust handling of floats and precise calculations.
By understanding and applying these tips, you'll be better equipped to manage javascript floats
effectively. If you're looking to expand your JavaScript knowledge, check out this comprehensive course on JavaScript. For beginners, a solid foundation in HTML and CSS is crucial, and you can find excellent resources in our introduction to web development course.
For further reading on JavaScript numbers and precision, reputable sources like MDN Web Docs, ECMA-262, and IEEE Standard for Floating-Point Arithmetic (IEEE 754) offer in-depth information.
By integrating these pro tips into your coding practice, you'll navigate the world of javascript floats
with greater confidence and precision.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
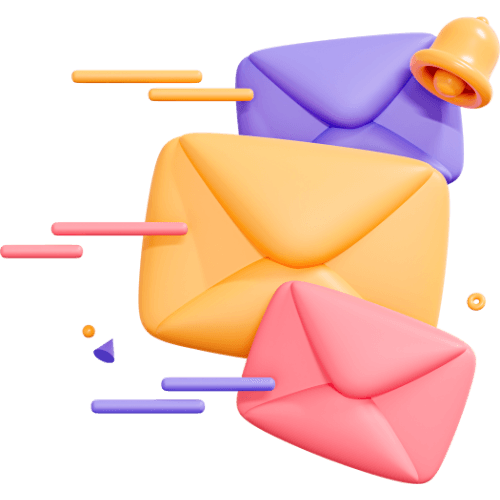
Related articles
125 Articles
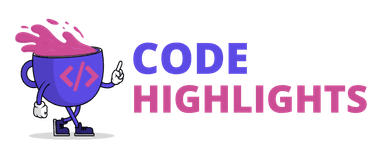
Copyright © Code Highlights 2024.