How to Fix 'JavaScript Foreach Is Not a Function' Quickly

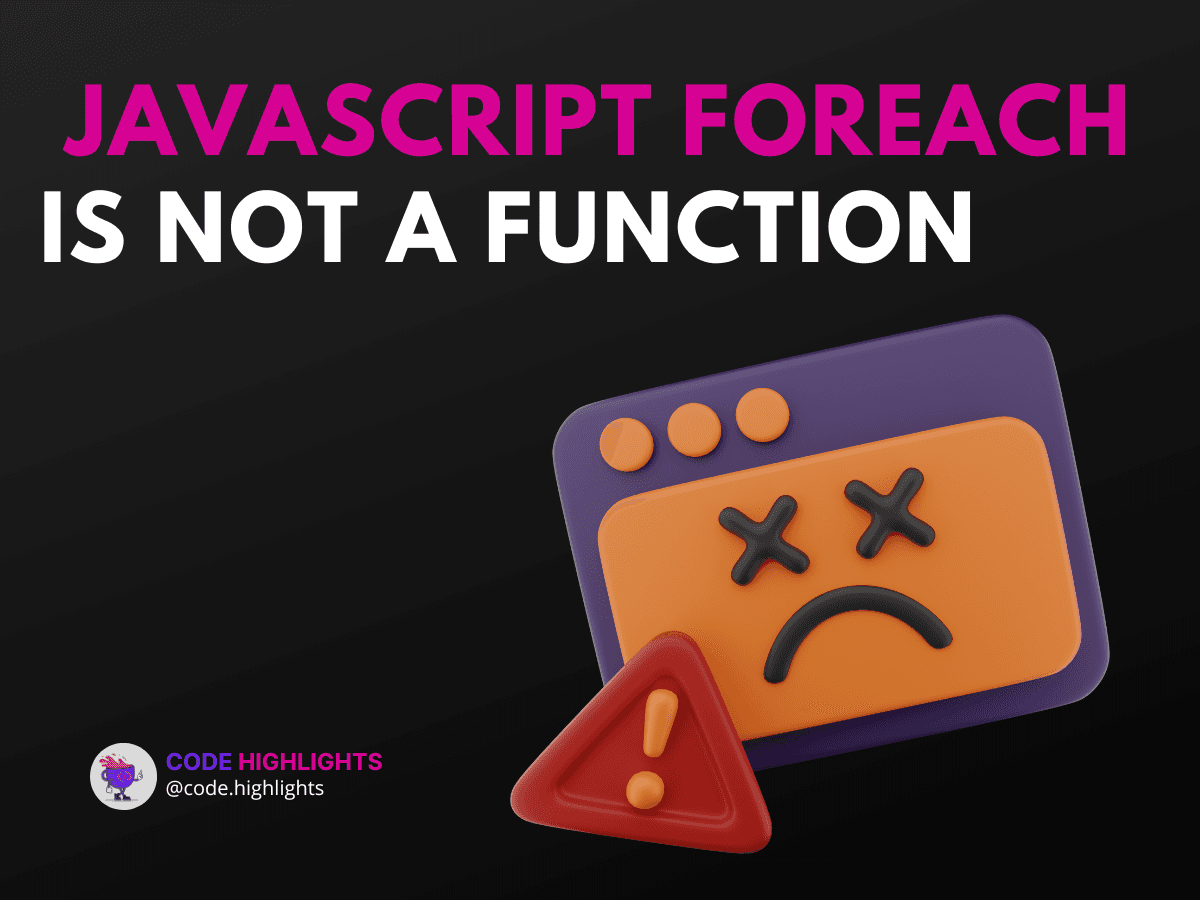
- Quick Code Example
- Understanding the Syntax
- Example 1: Using forEach with an Array
- Correct Usage
- Example 2: Fixing the Error with Array Check
- Adding a Check
- Example 3: Working with Objects
- Handling Objects
- Example 4: Using forEach with Other Functions
- Combining with Map
- Browser Compatibility
- Summary
If you've encountered the error message "javascript foreach is not a function," you're not alone. This issue typically arises when trying to use the forEach
method on a variable that isn't an array or doesn't support this function. In this tutorial, we will explore what causes this error and how to fix it effectively.
Quick Code Example
Here's a quick example to illustrate the error:
In this case, trying to call forEach
on null
will trigger the error. Let’s dive deeper into understanding the syntax and solutions.
Understanding the Syntax
The forEach
method is used with arrays in JavaScript. Its syntax looks like this:
- callback: A function that executes on each element.
- currentValue: The current element being processed.
- index (optional): The index of the current element.
- array (optional): The array
forEach
was called upon. - thisArg (optional): Value to use as
this
when executing the callback.
The return value of forEach
is undefined
.
Example 1: Using forEach with an Array
Correct Usage
In this example, forEach
iterates over the fruits
array and logs each fruit to the console. This is the correct way to use forEach
.
Example 2: Fixing the Error with Array Check
Adding a Check
To avoid the error, ensure the variable is an array. Here’s how:
1let items = null;
2
3if (Array.isArray(items)) {
4 items.forEach(item => console.log(item));
5} else {
6 console.log("items is not an array");
7}
This code checks if items
is an array before calling forEach
. If it isn’t, it logs a friendly message instead.
Example 3: Working with Objects
Handling Objects
The forEach
method does not work on objects directly. You can convert an object’s values to an array first:
This example uses Object.values()
to get an array of the object's values and then applies forEach
.
Example 4: Using forEach with Other Functions
Combining with Map
You can also combine forEach
with other array methods, like map
:
Here, map
doubles each number, and forEach
prints the results.
Browser Compatibility
The forEach
method is widely supported across all major browsers, including Chrome, Firefox, Safari, and Edge. However, if you need to support older browsers, consider checking compatibility tables on MDN Web Docs.
Summary
In this tutorial, we covered the common error "javascript foreach is not a function." We learned about the proper syntax for forEach
, how to check if a variable is an array, and how to handle objects and combine methods. Remember, always check the type of your variable before using forEach
to avoid errors.
For more information on JavaScript, you can explore our courses on JavaScript, HTML Fundamentals, and CSS Introduction. If you're starting from scratch, check out our course on Introduction to Web Development. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
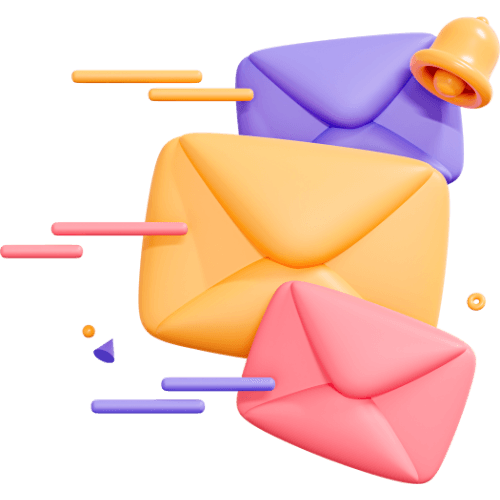
Related articles
9 Articles
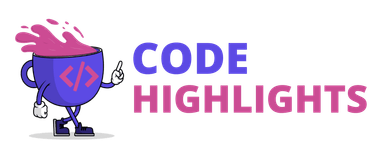
Copyright © Code Highlights 2025.