7 Proven Javascript Function Composition Techniques for 2024

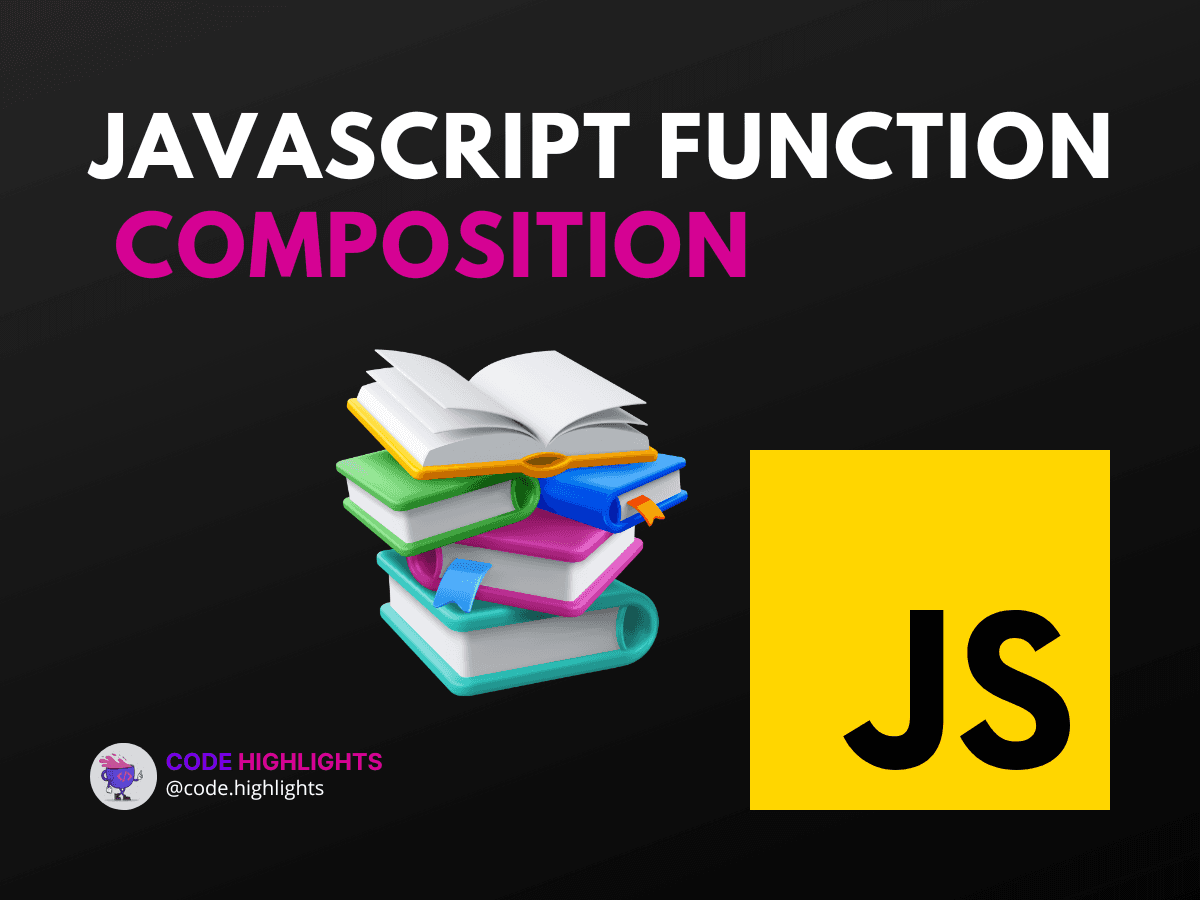
- Understanding Function Composition in JavaScript
- What is Composition Function in JS?
- What is a Composition in Functions?
- How Do You Know if a Function is Composite JS?
- What Are the Benefits of Function Composition?
- Techniques for Function Composition
- Let's Get Hands-On
- Conclusion
Welcome to the world of JavaScript, where functions are not just building blocks but also powerful tools for crafting efficient and maintainable code. In this tutorial, we'll delve into the concept of javascript function composition, a technique that can significantly enhance your coding skills and project quality.
Before we jump into the details, let's quickly touch base with an introductory code example that showcases the syntax we'll be exploring:
1const greet = name => `Hello, ${name}!`;
2const exclaim = statement => `${statement.toUpperCase()}!!!`;
3const welcome = compose(greet, exclaim);
4
5console.log(welcome('World')); // "HELLO, WORLD!!!"
In the above snippet, compose
is a function that takes greet
and exclaim
as arguments and creates a new function welcome
. This is a sneak peek into the power of function composition.
Now, let's dive into the world of function composition and discover how it can transform the way you write JavaScript.
Understanding Function Composition in JavaScript
At its core, function composition involves combining two or more functions to create a new function. It's like creating a pipeline where the output of one function becomes the input of the next. This concept is not unique to JavaScript; it's a fundamental principle in functional programming.
What is Composition Function in JS?
In JavaScript, a composition function is a higher-order function that combines multiple functions into a single function. The resulting function allows data to flow through the composed functions in sequence, providing a clear and concise way to perform multiple operations.
What is a Composition in Functions?
Composition in functions refers to the process of applying one function to the result of another, chaining them together in a sequence. It's akin to nesting functions, but with a more structured and readable approach.
How Do You Know if a Function is Composite JS?
To determine if a function is composite in JavaScript, look for the following characteristics:
- It takes at least one other function as an argument.
- It returns a new function that, when called, executes the composed functions in order.
What Are the Benefits of Function Composition?
Function composition offers several benefits:
- Code Reusability: Compose small, reusable functions instead of duplicating code.
- Readability: Create a clear flow of data processing, making the code easier to follow.
- Debugging: Isolate problems within smaller, independent functions.
- Maintenance: Update parts of the code without affecting the whole.
Techniques for Function Composition
-
The Pipe Function: Similar to
compose
, but the functions are applied from left to right. It's useful when the order of operations is critical. -
Composing with Reduce: Use the
reduce
method to apply functions to an accumulator, which holds the current value after each function application. -
Point-Free Style: Write functions where the arguments are not explicitly stated, making the code cleaner and focused on the transformation.
-
Currying: Transform a function with multiple arguments into a sequence of functions each taking a single argument.
-
Partial Application: Fix a few arguments of a function, generating a new function with a smaller arity.
-
Using Compose Libraries: Leverage existing libraries like Ramda or lodash/fp that offer compose utilities, ensuring robustness and efficiency.
-
Async Composition: Combine asynchronous functions using promises or async/await to handle operations that involve waiting for results.
Let's Get Hands-On
To solidify your understanding of javascript function composition, let's create a simple function that combines several operations to process a string:
1// Define our simple functions
2const scream = str => str.toUpperCase();
3const exclaim = str => `${str}!`;
4const repeat = str => `${str} `.repeat(3);
5
6// Compose them
7const makeExcited = compose(scream, exclaim, repeat);
8
9// Use the composed function
10console.log(makeExcited('hello')); // "HELLO! HELLO! HELLO! "
In this example, makeExcited
is our composite function that turns a string into an excited, repeated shout.
Conclusion
By mastering javascript function composition, you unlock a new level of coding proficiency. As you practice these techniques, consider enrolling in courses to further enhance your skills (JavaScript Course, HTML Fundamentals, Introduction to CSS, Web Development Introduction). Also, don't forget to consult reputable sources to deepen your understanding (MDN Web Docs, JavaScript Info, Functional Light JS).
Embrace the power of function composition and watch as your JavaScript projects become more elegant and your workflow more efficient. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
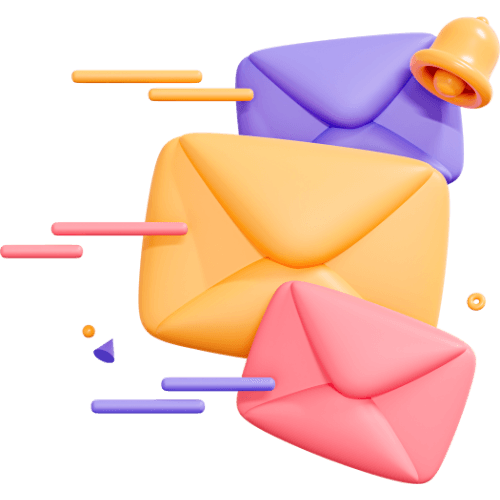
Related articles
9 Articles
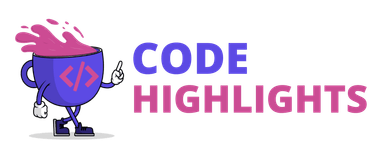
Copyright © Code Highlights 2025.