How to Get the Current Timezone in JavaScript

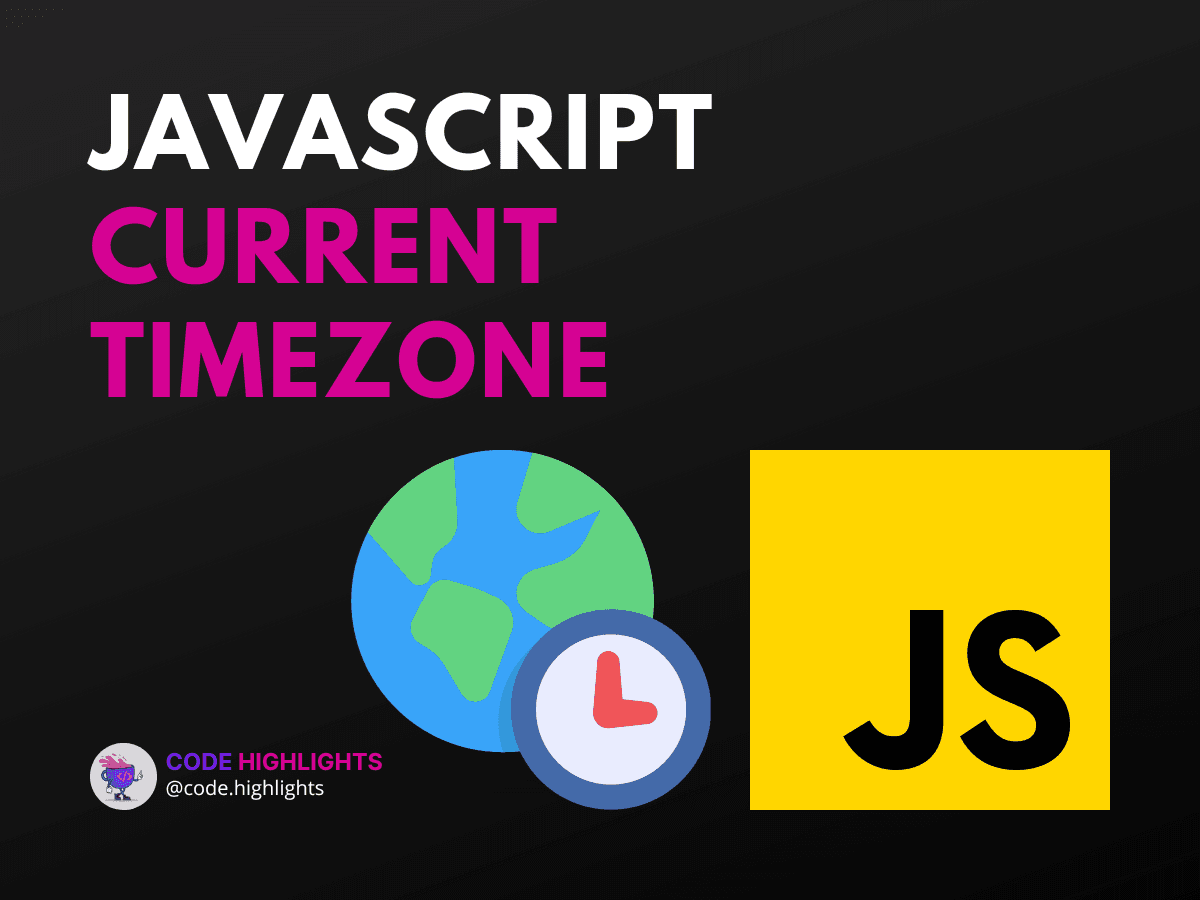
- Understanding Time Zones in JavaScript
- The Date Object
- Getting the Local Time Zone
- Fetching Current GMT and UTC Time
- Working with Timezone Intl
- Real-World Examples
- Scheduling Events Across Time Zones
- Browser Compatibility
- Wrapping Up
Managing time zones is a common challenge in web development. Whether you're scheduling events or logging timestamps, understanding how to handle time zones can greatly enhance your JavaScript applications. In this tutorial, we'll dive into how you can effectively get the current timezone using JavaScript.
Before we delve into the details, let's look at a basic code example that demonstrates how to obtain the local time zone in JavaScript:
1let currentTimeZone = Intl.DateTimeFormat().resolvedOptions().timeZone;
2console.log(currentTimeZone);
This line of code utilizes the Internationalization API available in JavaScript, which we will explore further in this tutorial.
Understanding Time Zones in JavaScript
Time zones are crucial for ensuring that users around the world receive data relevant to their locale. JavaScript offers several methods to work with time zones and dates, which we'll cover here.
The Date
Object
The Date
object in JavaScript is your starting point for all date-time related operations. It provides various methods to get and set date-time values.
Getting the Local Time Zone
To answer the question, "How to get the current time zone in JavaScript?" we use the Internationalization API:
1let localTimeZone = Intl.DateTimeFormat().resolvedOptions().timeZone;
2console.log(localTimeZone); // Output might be \"America/New_York\"
The Intl.DateTimeFormat
object is part of the ECMAScript Internationalization API, which provides language sensitive date and time formatting. The resolvedOptions()
method returns an object with properties reflecting the locale and formatting options computed during the initialization of the object.
Fetching Current GMT and UTC Time
GMT (Greenwich Mean Time) and UTC (Coordinated Universal Time) are often used interchangeably, as they both represent the time at the Prime Meridian (0° longitude). Here's how to get the current GMT/UTC time in JavaScript:
This snippet will print the current date and time in UTC.
Working with Timezone Intl
The Intl object we mentioned earlier provides several constructors to work with internationalization. To get timezone Intl in JavaScript, you would still use the DateTimeFormat
as shown previously. However, you can also format dates according to different time zones like so:
1let formatter = new Intl.DateTimeFormat("en-US", { timeZone: "Europe/London" });
2console.log(formatter.format(new Date()));
This code will output the current date and time in London.
Real-World Examples
Scheduling Events Across Time Zones
When scheduling events for users across different time zones, you can convert the event time to the user's local time zone using the Intl.DateTimeFormat
:
1let eventTimeUTC = new Date("2023-04-14T12:00:00Z");
2let userTimeZone = Intl.DateTimeFormat().resolvedOptions().timeZone;
3let eventTimeLocal = new Intl.DateTimeFormat("en-US", {
4 timeZone: userTimeZone,
5 hour: "2-digit",
6 minute: "2-digit",
7 second: "2-digit",
8}).format(eventTimeUTC);
9
10console.log(`Event time in your time zone: ${eventTimeLocal}`);
Browser Compatibility
The methods described are widely supported in major web browsers, including Chrome, Firefox, Safari, and Edge. Always ensure you check the compatibility for specific features, especially if you're targeting older browser versions.
Wrapping Up
In this tutorial, we've explored how to use JavaScript to get the current timezone, along with GMT and UTC times. We've seen how the powerful Intl API can be utilized for these purposes and discussed some practical applications.
Whether you're a beginner looking to learn JavaScript, or you're interested in HTML fundamentals, understanding how to manage time zones is an invaluable skill.
For further reading on date and time handling in JavaScript, consider these reputable sources:
- MDN Web Docs on Date
- ECMAScript Internationalization API Specification
- You Don't Know JS: Date & Time
Remember, practice makes perfect. Try out these examples and experiment with different time zones to master JavaScript's date-time capabilities.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
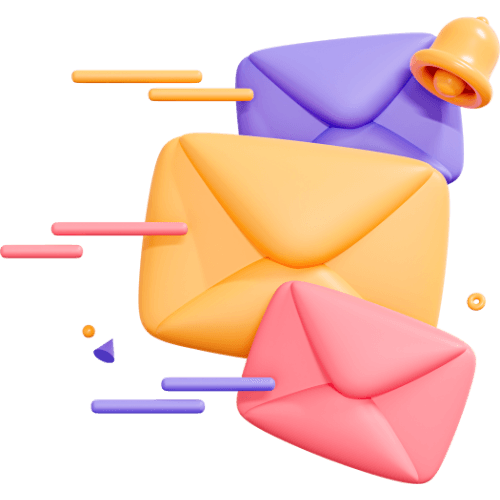
Related articles
9 Articles
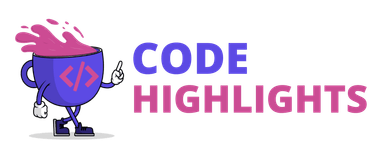
Copyright © Code Highlights 2025.