How to JS: Get Element by Class - Tips & Hacks

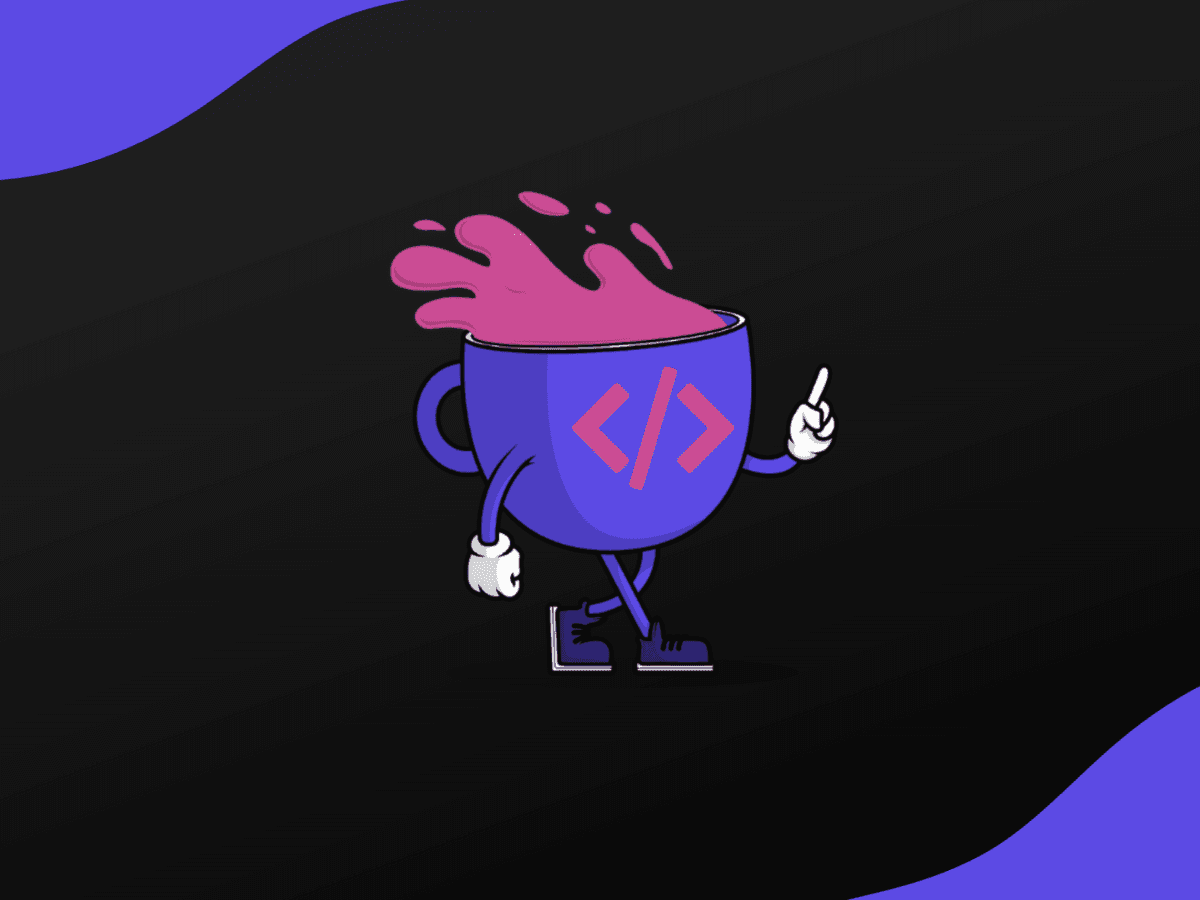
- getElementsByClassName()
- Finding Specific Element
- Checking if Element Exists
- Getting Child Elements
- Browser Compatibility
In JavaScript, a common task is to interact with HTML elements. Sometimes, you might want to find and manipulate elements based on their class name. In this tutorial, we will explore how to use JavaScript to get elements by class name.
getElementsByClassName()
The getElementsByClassName()
method allows you to select HTML elements based on their class name. It returns a live HTMLCollection of found elements.
This will return all elements with the class myClass
.
Finding Specific Element
To find a specific element with a certain class in JavaScript, you can access it like an array.
Checking if Element Exists
Before manipulating elements, it's good practice to check if the element exists. Here's how to check if an element exists by class in JavaScript:
Getting Child Elements
You can also use getElementsByClassName()
to get child elements using class in JavaScript. Simply call the method on the parent element.
Browser Compatibility
All modern browsers support getElementsByClassName()
. However, if you're working with IE8 or below, consider using jQuery's $('.myClass')
instead.
Check out our JavaScript course for more tutorials like this. If you're new to web development, our web development introduction course might be helpful.
For more details about getElementsByClassName()
, visit MDN web docs.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
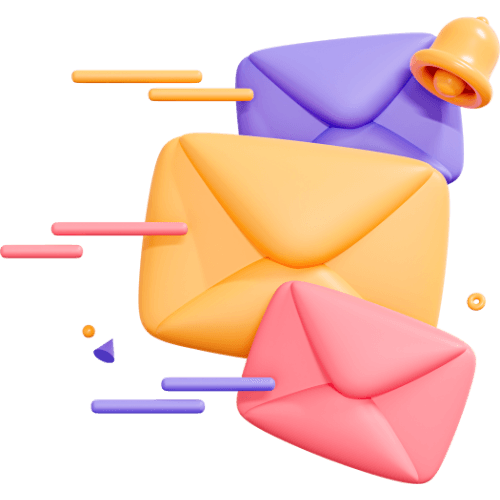
Related articles
9 Articles
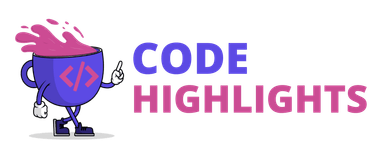
Copyright © Code Highlights 2025.