The Secret to Getting JavaScript Get Function Arguments Fast

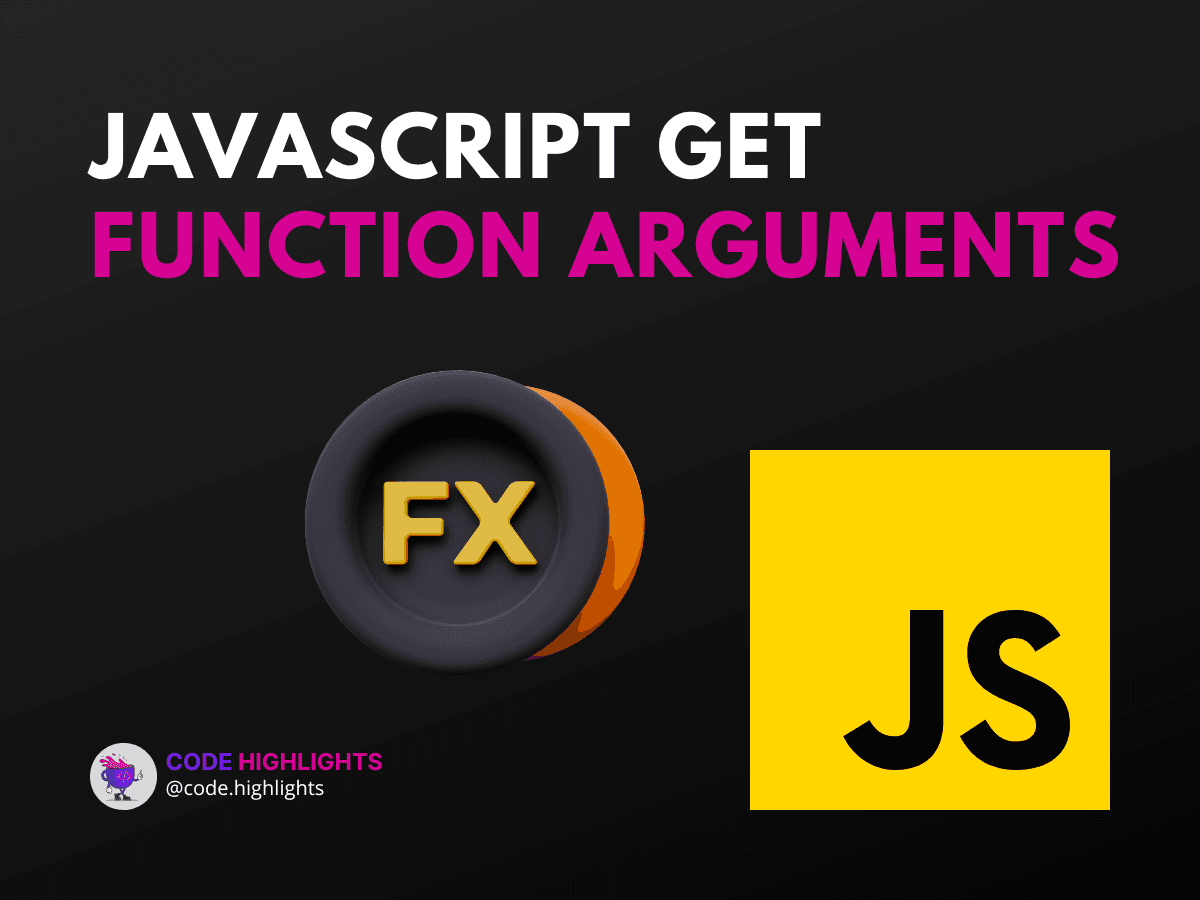
- Peeking Under the Hood: Accessing Function Arguments
- How to See the Arguments of a Function in JavaScript?
- Determining Argument Types
- Calling Functions with Arguments
- Retrieving Values from Functions
Ever wondered how to tap into the power of JavaScript functions and their arguments? Whether you're a budding coder or a seasoned developer, understanding how to work with function arguments is crucial for creating dynamic and flexible code. In this tutorial, we'll unravel the secrets behind JavaScript function arguments and how to handle them effectively.
Before we dive deep, let's set the stage with a simple example. Imagine a function that greets a user:
This snippet introduces us to the syntax of defining and calling a function with arguments. But there's so much more to explore!
Peeking Under the Hood: Accessing Function Arguments
In JavaScript, each function comes with a built-in object called arguments
. This special object holds all the arguments passed to the function. Let's see it in action:
1function showArguments() {
2 console.log(arguments);
3}
4showArguments('Learning', 'JavaScript', 'is', 'fun!');
When you run this code, you'll see an array-like object logged to the console, containing all the arguments you passed in. It's like having a backstage pass to your function's parameters!
How to See the Arguments of a Function in JavaScript?
To get a closer look at the function's arguments, you can iterate over the arguments
object using a loop. Here's how:
1function listArguments() {
2 for (let i = 0; i < arguments.length; i++) {
3 console.log(`Argument ${i}:`, arguments[i]);
4 }
5}
6listArguments('JavaScript', 'is', 'awesome!');
This will print out each argument with its corresponding index. Simple, right?
Determining Argument Types
But what if you need to know the type of each argument? JavaScript provides the typeof
operator for this exact purpose:
1function argumentTypes() {
2 for (let i = 0; i < arguments.length; i++) {
3 console.log(`Argument ${i} is of type:`, typeof arguments[i]);
4 }
5}
6argumentTypes('Hello', 42, true, null, undefined, {});
By running this code, you'll see the data type of each argument displayed in the console.
Calling Functions with Arguments
When calling a function, you simply list the arguments within the parentheses. For instance:
This example shows how to pass arguments when invoking a function and how to retrieve the result.
Retrieving Values from Functions
To get a value from a function, you use the return
statement. Here's a quick example:
1function multiply(x, y) {
2 return x * y;
3}
4let result = multiply(6, 7);
5console.log(result); // Outputs: 42
The multiply
function calculates the product of x
and y
, and then returns it. We store this returned value in the result
variable.
Ready to take your JavaScript skills to the next level? Dive into our JavaScript course, where you'll find a wealth of knowledge to enhance your coding journey.
For those who are just starting out, understanding the basics of HTML and CSS is also critical. Check out our HTML fundamentals course and Introduction to CSS to build a solid foundation. And if you're looking to understand the bigger picture, our Introduction to Web Development course is the perfect place to start.
For additional resources, consider these reputable external sources:
In conclusion, mastering JavaScript function arguments opens up a world of possibilities. By understanding how to access, determine the type of, and work with arguments, you're well on your way to writing more efficient and powerful JavaScript code. Keep practicing, and soon, handling function arguments will be second nature to you!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
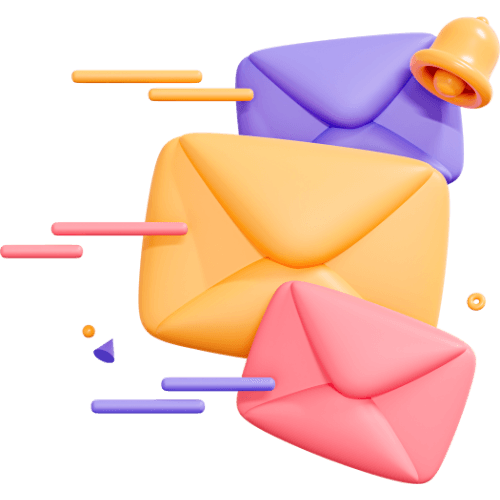
Related articles
9 Articles
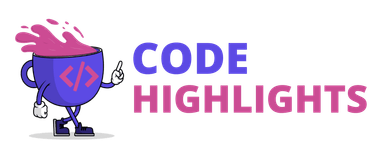
Copyright © Code Highlights 2024.