JavaScript hasOwnProperty() Method

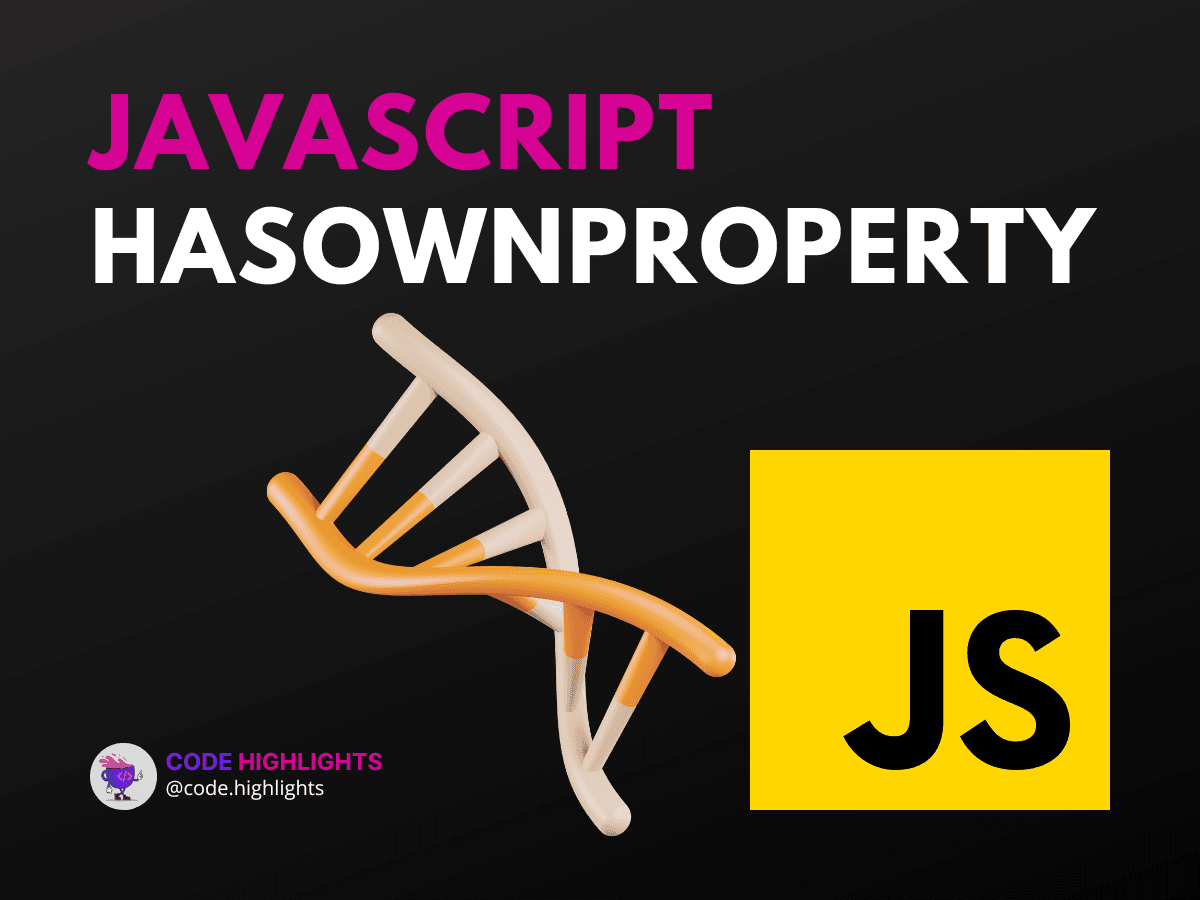
- Understanding hasOwnProperty()
- Checking Property Existence
- Object Keys vs. hasOwnProperty()
- Practical Examples
- Conclusion
JavaScript hasOwnProperty() Method provides a straightforward way to determine if an object has a specific property as its own. Before we dive deep into the syntax and use cases, let's take a quick peek at how hasOwnProperty()
is used in JavaScript:
1const car = {
2 make: 'Toyota',
3 model: 'Corolla'
4};
5
6console.log(car.hasOwnProperty('make')); // Output: true
In this tutorial, we'll explore the intricacies of the hasOwnProperty()
method, learn how to check if a property exists in an object, and understand the distinction between an object's key and hasOwnProperty()
. Whether you're a seasoned coder or just starting out, mastering this method will enhance your JavaScript toolkit.
Understanding hasOwnProperty()
The hasOwnProperty()
method is a part of JavaScript's Object prototype. It's used to check whether an object contains a property as a direct, non-inherited feature. You might wonder, "What does hasOwnProperty()
do in JavaScript that a simple property check can't?" Well, it ensures that the property belongs specifically to the object in question, not inherited from its prototype chain.
1const gadget = {
2 price: 199
3};
4
5console.log(gadget.hasOwnProperty('price')); // true
6console.log(gadget.hasOwnProperty('toString')); // false
Here, gadget
has its own price
property, but toString
is inherited from the Object prototype, hence hasOwnProperty()
returns false
.
Checking Property Existence
So, how to check if a property exists in JavaScript? While you can use the in
operator, hasOwnProperty()
gives you more precision by ignoring the prototype's properties.
1if (gadget.hasOwnProperty('price')) {
2 console.log('Price is specified.');
3} else {
4 console.log('Price is not specified.');
5}
This check is particularly useful when iterating over object keys using a for...in
loop, allowing you to focus only on the object's own properties.
Object Keys vs. hasOwnProperty()
Understanding the difference between object keys and hasOwnProperty()
is crucial in JavaScript. Object keys can be retrieved using Object.keys(obj)
, which lists all enumerable property names. But it doesn't tell you if those properties are inherited or not. That's where hasOwnProperty()
comes in, helping you discern if those keys are indeed the object's own.
1const device = {
2 brand: 'OnePlus',
3 screen: 'AMOLED'
4};
5
6const keys = Object.keys(device);
7keys.forEach(key => {
8 if (device.hasOwnProperty(key)) {
9 console.log(`${key} is an own property.`);
10 }
11});
Practical Examples
Let's apply our knowledge with some practical examples. Consider checking if an object has its own properties in JavaScript:
1const user = {
2 id: 42,
3 name: 'Alice'
4};
5
6console.log(user.hasOwnProperty('id')); // true
7console.log(user.hasOwnProperty('name')); // true
8console.log(user.hasOwnProperty('hasOwnProperty')); // false
Notice how hasOwnProperty
itself is not an own property since it's inherited from the Object prototype.
Conclusion
The javascript hasOwnProperty
method is an essential part of working with objects. It provides a reliable way to verify property ownership within an object, distinguishing between own properties and those inherited through the prototype chain. As you continue to build and manipulate objects, keep this method in mind for cleaner, more efficient code.
Looking to expand your web development skills further? Check out these courses:
For more information on JavaScript and its myriad of methods, don't hesitate to delve into external resources such as MDN Web Docs or W3Schools. These reputable sources offer in-depth explanations and examples that can further solidify your understanding of the JavaScript language. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
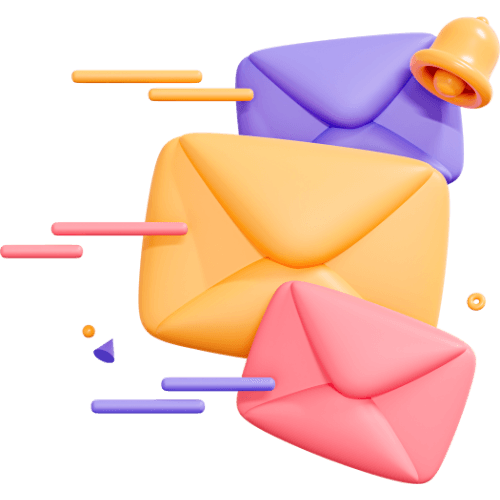
Related articles
9 Articles
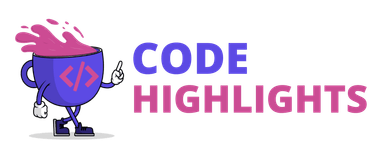
Copyright © Code Highlights 2025.