5 Tips to Fix JavaScript Heap Out of Memory Issues

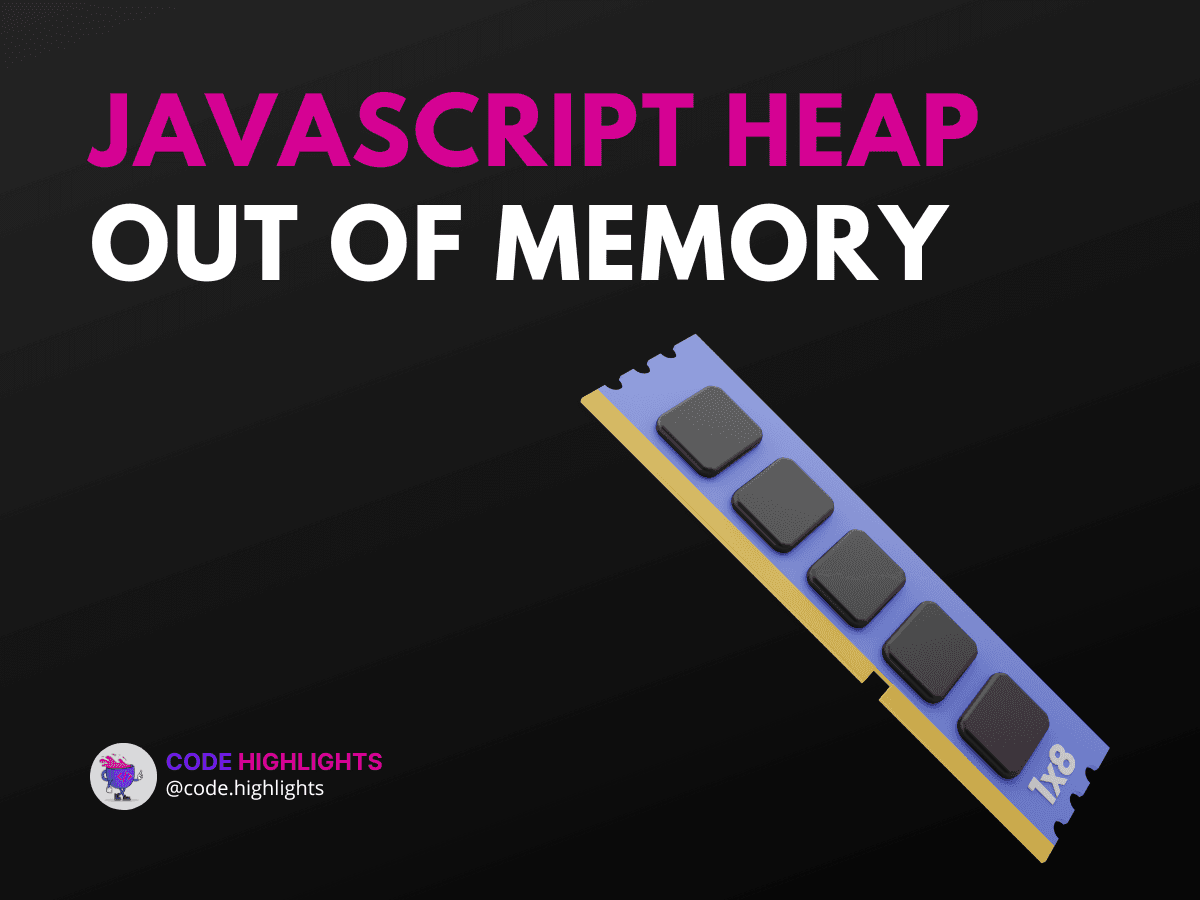
- Understanding JavaScript Heap Out of Memory
- Syntax and Parameters
- Tip 1: Increase Node.js Heap Size
- Tip 2: Optimize Your Code
- Tip 3: Use Streaming for Large Data
- Tip 4: Monitor Memory Usage
- Tip 5: Garbage Collection
- Compatibility with Major Browsers
- Summary
JavaScript is a powerful language used widely in web development. However, running complex applications can sometimes lead to a "heap out of memory" error. This tutorial will explain what causes this issue and provide practical tips to fix it.
Understanding JavaScript Heap Out of Memory
When JavaScript runs, it uses a portion of the computer's memory called the heap to store objects. If your application tries to use more memory than is available, you'll encounter a heap out of memory error. Here's a simple example that might trigger this error:
Syntax and Parameters
The above code creates an infinite loop that continuously adds large arrays to arr
, eventually exhausting the available heap memory. To understand this better, let's break down the components:
- Array: Creates a new array.
- fill(): Fills the array with a specified value.
- push(): Adds new items to the end of an array.
Tip 1: Increase Node.js Heap Size
One way to handle memory issues is by increasing the heap size. You can do this by using the --max-old-space-size
flag when running your Node.js application:
1node --max-old-space-size=4096 your_script.js
This command increases the heap size to 4GB. Adjust the value based on your application's needs.
Tip 2: Optimize Your Code
Efficient coding practices can help you avoid memory issues. For instance, avoid creating large arrays or objects unless absolutely necessary. Here's a better approach to handling data:
By limiting the size of the arrays, you reduce the risk of running out of memory.
Tip 3: Use Streaming for Large Data
When dealing with large files or data streams, use streaming to process data in chunks rather than loading everything into memory at once. Here's an example using Node.js streams:
1const fs = require('fs');
2const readStream = fs.createReadStream('largefile.txt');
3readStream.on('data', (chunk) => {
4 console.log(chunk);
5});
Streaming helps manage memory more efficiently by processing data piece by piece.
Tip 4: Monitor Memory Usage
Regularly monitor your application's memory usage to identify potential issues early. You can use tools like Chrome DevTools or Node.js built-in modules:
This code snippet logs the current heap statistics, helping you track memory consumption.
Tip 5: Garbage Collection
JavaScript has automatic garbage collection, but you can manually trigger it if needed. Use the --expose-gc
flag and call global.gc()
in your code:
1node --expose-gc your_script.js
1if (global.gc) {
2 global.gc();
3} else {
4 console.log('Garbage collection unavailable. Use --expose-gc');
5}
Manual garbage collection can help free up memory, especially in long-running applications.
Compatibility with Major Browsers
JavaScript heap memory management is consistent across major browsers like Chrome, Firefox, and Edge. However, if you're running server-side code with Node.js, the heap size can be adjusted as shown earlier.
Summary
In this tutorial, we covered five essential tips to address JavaScript heap out of memory issues:
- Increase Node.js heap size.
- Optimize your code.
- Use streaming for large data.
- Monitor memory usage.
- Utilize garbage collection.
These strategies will help you manage memory effectively and keep your applications running smoothly. For more in-depth learning, consider exploring our JavaScript course or Introduction to Web Development.
For additional resources, check out these best practices for memory management and Node.js performance tips from reputable sources.
By following these tips, you can prevent heap out of memory errors and ensure your JavaScript applications perform optimally.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
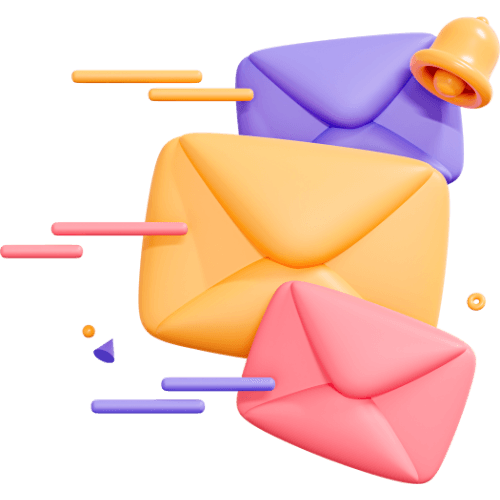
Related articles
9 Articles
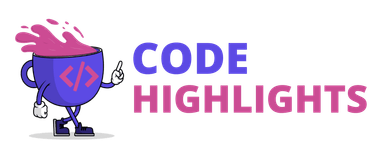
Copyright © Code Highlights 2025.