Avoid Confusion: JavaScript Import vs Require with These Simple Tips

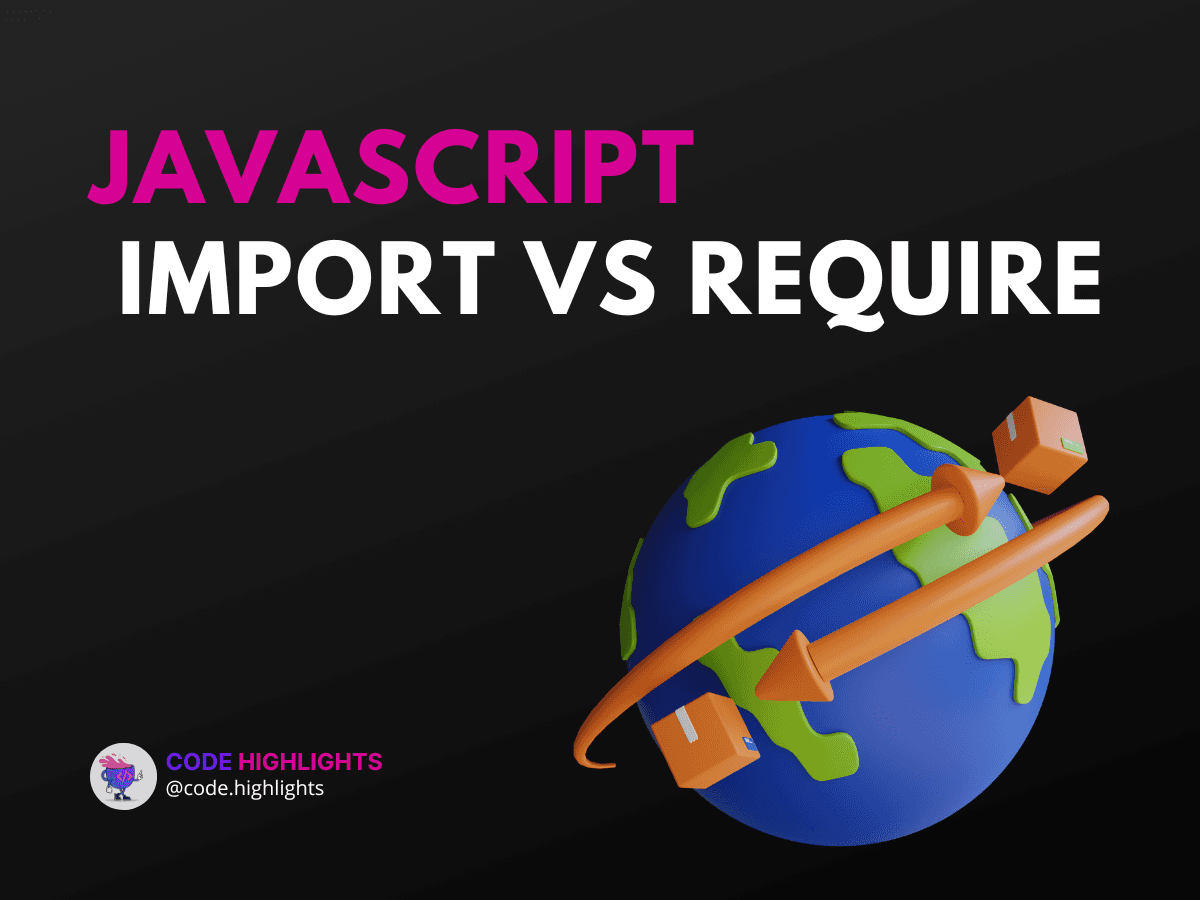
- Quick Code Example
- Syntax Overview
- require()
- import
- Key Differences
- Example 1: Basic Usage of require()
- Example 2: Basic Usage of import
- Example 3: Importing Multiple Exports
- When to Use import vs require()
- Compatibility with Major Browsers
- Summary
Understanding how to manage modules in JavaScript can be tricky, especially when you're faced with the choice between import
and require()
. Both are essential for including code from other files, but they work differently. In this tutorial, we will explore these two methods, their usage, and when to use each one. By the end, you'll have a solid grasp of the differences, helping you avoid confusion in your coding projects.
Quick Code Example
Before diving deeper, let’s look at a simple example of both methods:
1// Using require
2const myModule = require('./myModule');
3
4// Using import
5import myModule from './myModule.js';
Syntax Overview
require()
The require()
function is part of Node.js and is used to include modules. Its syntax is straightforward:
- Parameters: The string passed is the path to the module or package.
- Return Value: It returns the exported object from the required module.
import
The import
statement is part of the ES6 (ECMAScript 2015) syntax and is used in modern JavaScript environments.
- Parameters: Similar to
require()
, it takes a string that specifies the module path. - Return Value: It also returns the exported object from the module.
Key Differences
- Environment:
require()
is mainly used in Node.js, whileimport
is used in modern JavaScript (ES6). - Loading Behavior:
require()
loads modules synchronously, whileimport
works asynchronously.
Example 1: Basic Usage of require()
Here’s how you can use require()
in a Node.js environment:
1// myModule.js
2module.exports = function() {
3 return 'Hello from myModule!';
4};
5
6// main.js
7const greet = require('./myModule');
8console.log(greet()); // Output: Hello from myModule!
This example shows how to export a function from one file and import it into another using require()
.
Example 2: Basic Usage of import
Now, let’s see how to use import
:
1// myModule.js
2export default function() {
3 return 'Hello from myModule!';
4}
5
6// main.js
7import greet from './myModule.js';
8console.log(greet()); // Output: Hello from myModule!
In this case, we use export default
to allow our function to be imported easily.
Example 3: Importing Multiple Exports
You can also import multiple exports using the import
statement:
1// myModule.js
2export const greeting = 'Hello';
3export const farewell = 'Goodbye';
4
5// main.js
6import { greeting, farewell } from './myModule.js';
7console.log(greeting); // Output: Hello
8console.log(farewell); // Output: Goodbye
This demonstrates how to import specific named exports from a module.
When to Use import
vs require()
- Use
require()
when working in a Node.js environment, especially if you need synchronous loading. - Use
import
when working with modern JavaScript, particularly in front-end development or when using frameworks like React.
Compatibility with Major Browsers
The import
statement is widely supported in modern browsers, while require()
is primarily for Node.js. If you're targeting web applications, you should lean towards using import
. For more details on browser compatibility, check out MDN Web Docs.
Summary
In this tutorial, we covered the key differences between javascript import vs require
. We learned that require()
is used in Node.js for synchronous module loading, while import
is part of ES6 and is used for asynchronous loading in modern JavaScript. Knowing when to use each can help streamline your coding process.
For those looking to expand their knowledge, consider checking out our courses on HTML Fundamentals and Introduction to Web Development for a broader understanding of web technologies.
By mastering these concepts, you'll be better equipped to handle module management in your projects!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
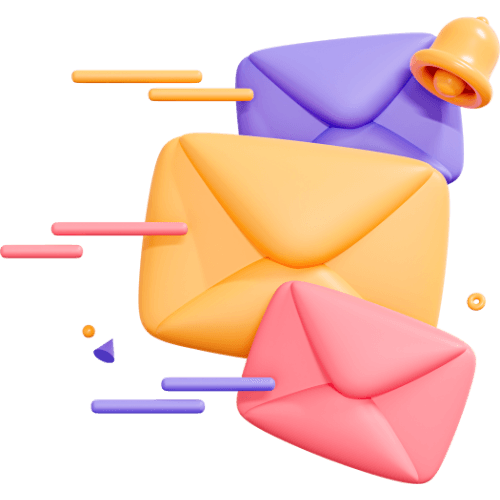
Related articles
9 Articles
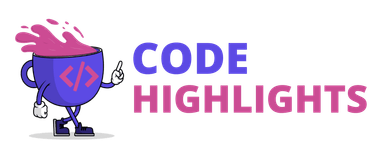
Copyright © Code Highlights 2024.