Master JavaScript Join: Top 10 Techniques for Developers

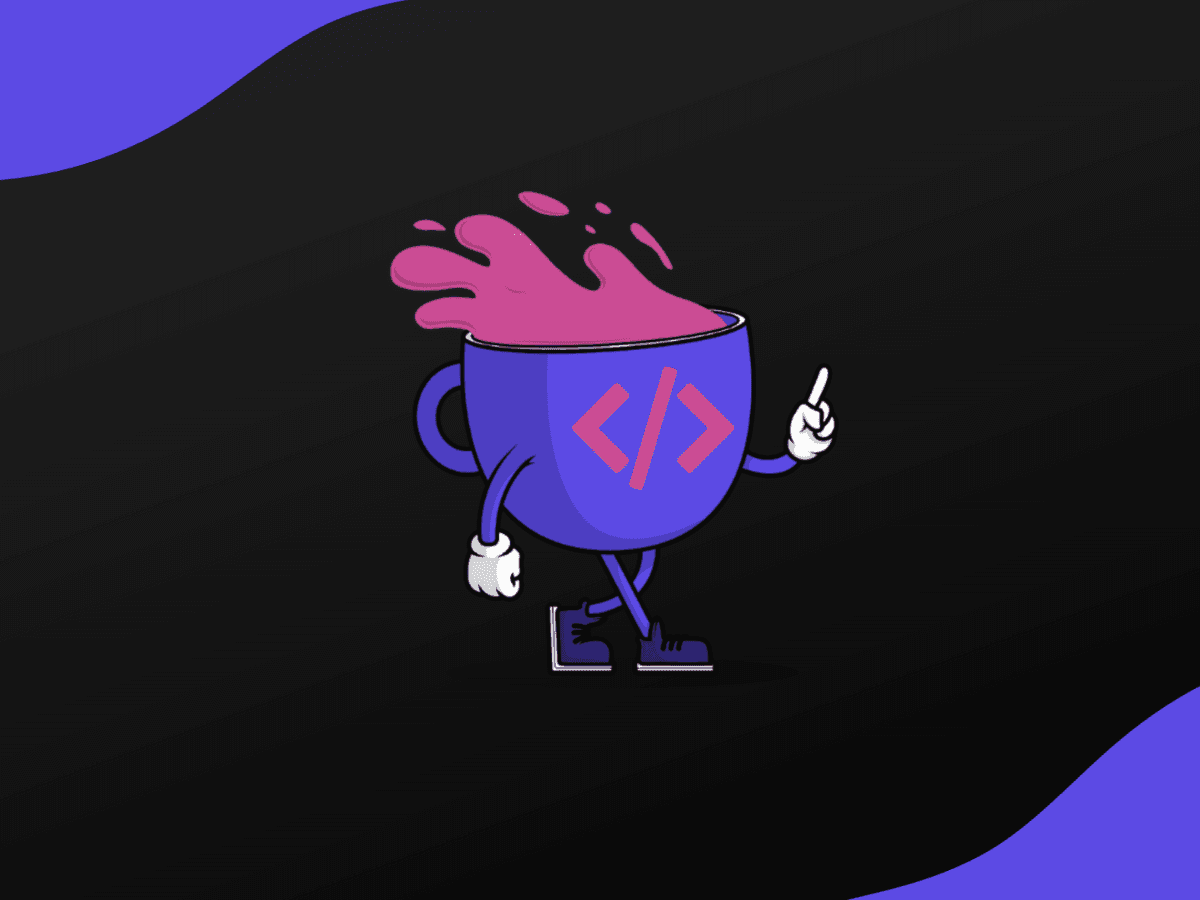
- Basic Usage
- Custom Separator
- Joining Numbers
- Nested Arrays
- Empty Arrays
- Undefined and Null
- Joining Array-Like Objects
- Performance
- Browser Compatibility
In JavaScript, arrays are a powerful tool for storing and manipulating data. One of the most useful methods provided by the Array object is join()
. This method allows you to combine all elements in an array into a single string.
1var arr = ["Master", "JavaScript", "Join"];
2var str = arr.join(); // returns "Master,JavaScript,Join"
Today, let's explore the top 10 techniques for using join()
effectively in your JavaScript development.
Basic Usage
At its simplest, join()
combines all elements in an array into a string, separated by commas.
Custom Separator
You can specify a different separator by passing it as an argument to join()
.
1var arr = ["apple", "banana", "cherry"];
2var str = arr.join(" - "); // returns "apple - banana - cherry"
Joining Numbers
join()
works with arrays of numbers too. It converts each number to a string before joining.
Nested Arrays
join()
turns nested arrays into strings too, but the results may surprise you.
1var arr = [["apple", "banana"], ["cherry", "date"]];
2var str = arr.join(); // returns "apple,banana,cherry,date"
Empty Arrays
If you call join()
on an empty array, it returns an empty string.
Undefined and Null
join()
treats undefined
and null
as empty strings.
Joining Array-Like Objects
With a little extra work, you can use join()
on array-like objects too.
1var obj = {0: "apple", 1: "banana", length: 2};
2var str = Array.prototype.join.call(obj, ", "); // returns "apple, banana"
Performance
When concatenating large arrays, join()
is significantly faster than using +
or +=
.
1var arr = new Array(1000000).fill("apple");
2console.time("join");
3var str = arr.join();
4console.timeEnd("join"); // Faster
Browser Compatibility
All modern browsers, and IE6 and up, support the join()
method. If you're new to JavaScript, check out our Learn JavaScript course for a comprehensive introduction.
For more in-depth information about JavaScript's join()
method, visit Mozilla Developer Network's documentation.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
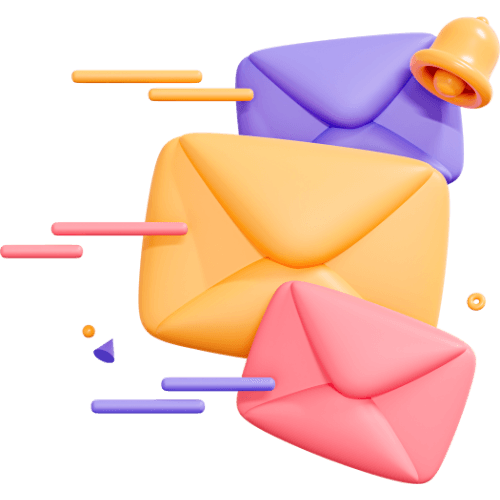
Related articles
9 Articles
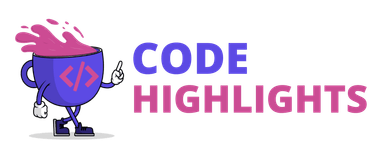
Copyright © Code Highlights 2025.