7 Tips for JavaScript Length of String Mastery

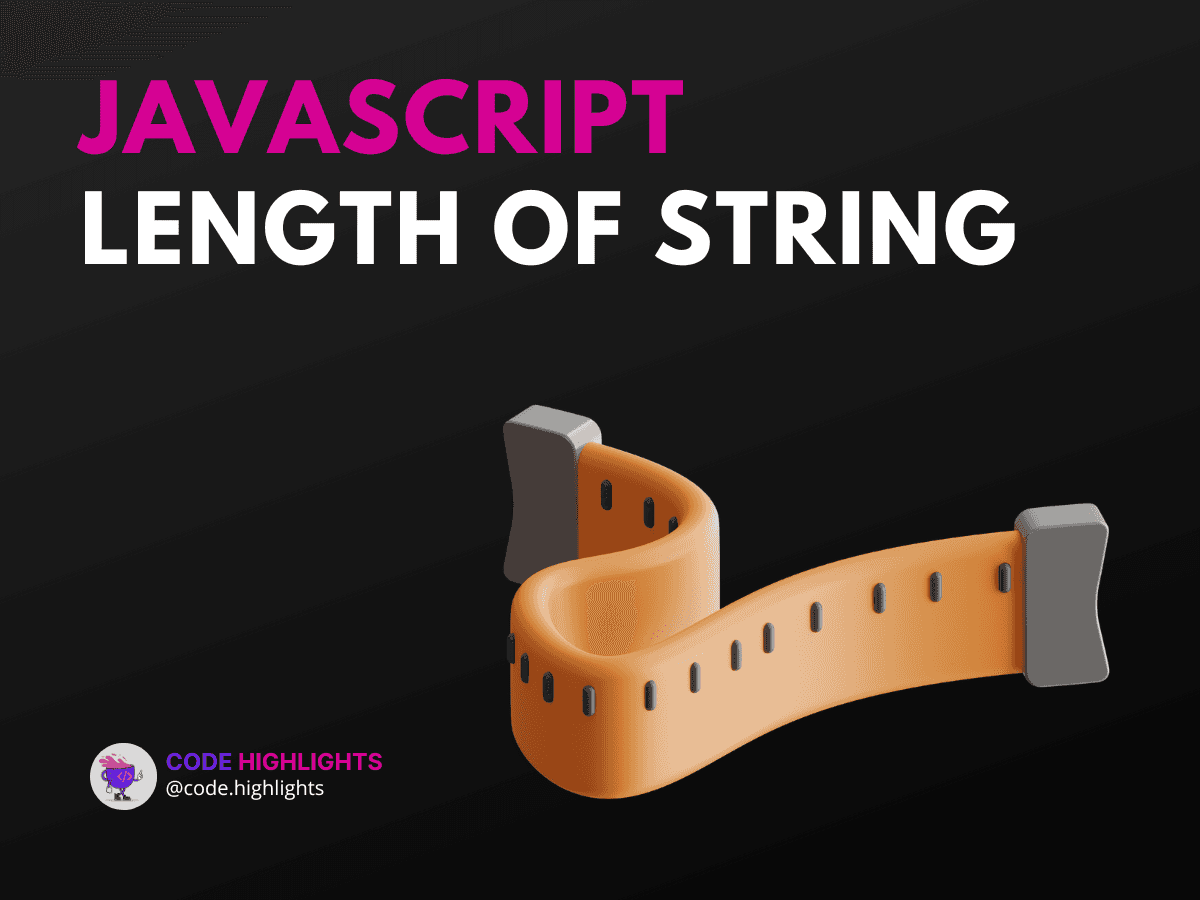
- Introduction to .length Property
- Syntax Breakdown
- Variations in Usage
- Practical Tips and Examples
- 1. Counting Characters in User Input
- 2. Trimming Strings Before Counting
- 3. Handling Multiline Strings
- 4. Combining .length with Other Functions
- 5. Finding Length of Array Elements
- 6. Validating Password Length
- 7. Optimizing Performance with .length
- Browser Compatibility
- Summary
Understanding how to find the length of a string in JavaScript is crucial for many coding tasks. Whether you're validating input, formatting text, or manipulating data, mastering the .length
property will simplify your work. Let's dive into some practical tips and examples to help you become proficient with the javascript length of string
.
Introduction to .length
Property
The .length
property in JavaScript is used to determine the number of characters in a string. Here’s a quick example:
Syntax Breakdown
- Property:
.length
- Parameters: None
- Return Value: Integer representing the number of characters in the string
Variations in Usage
The .length
property can be applied to any string variable or string literal. Here are a few variations:
Practical Tips and Examples
1. Counting Characters in User Input
When handling user input, you might need to count the number of characters entered. This can be useful for form validation.
1let userInput = prompt("Enter your name:");
2if (userInput.length < 3) {
3 alert("Name must be at least 3 characters long.");
4}
2. Trimming Strings Before Counting
Sometimes, you might want to ignore leading and trailing whitespace when counting characters.
1let rawInput = " Hello! ";
2let trimmedInput = rawInput.trim();
3console.log(trimmedInput.length); // Output: 6
3. Handling Multiline Strings
Multiline strings can also be handled using the .length
property.
4. Combining .length
with Other Functions
You can combine .length
with other string methods for more complex operations.
1let sentence = "The quick brown fox jumps over the lazy dog.";
2let words = sentence.split(" ");
3console.log(words.length); // Output: 9
5. Finding Length of Array Elements
The .length
property can also be used with array elements.
1let fruits = ["apple", "banana", "cherry"];
2fruits.forEach(fruit => {
3 console.log(`${fruit}: ${fruit.length} characters`);
4});
6. Validating Password Length
Ensuring a password meets length requirements is a common task.
1let password = "securePassword123";
2if (password.length >= 8) {
3 console.log("Password is valid.");
4} else {
5 console.log("Password is too short.");
6}
7. Optimizing Performance with .length
Using the .length
property is efficient and doesn't require additional computation.
Browser Compatibility
The .length
property is compatible with all major web browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer. Therefore, you can confidently use it in your web applications without worrying about compatibility issues.
Summary
In this tutorial, we covered the importance of the .length
property in JavaScript for finding the length of a string. We explored various practical examples, from counting characters in user input to validating password length. By mastering these tips, you'll be well-equipped to handle string length operations efficiently in your JavaScript projects.
For more in-depth learning, consider exploring our JavaScript courses and related topics on HTML fundamentals, CSS introduction, and web development basics.
For further reading, check out these reputable sources:
- MDN Web Docs on String Length
- W3Schools JavaScript String Length
- GeeksforGeeks JavaScript String Length
By integrating these tips into your coding practice, you'll gain confidence and efficiency in handling string lengths in JavaScript. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
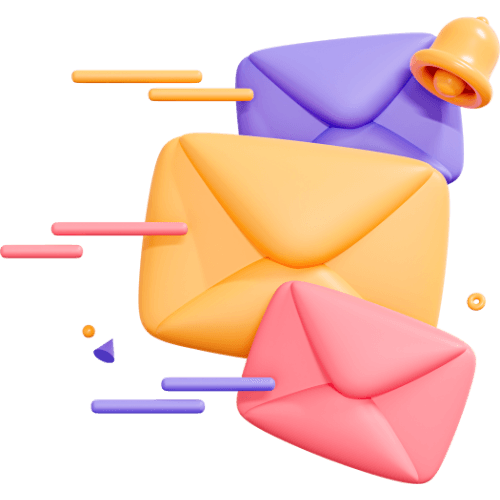
Related articles
9 Articles
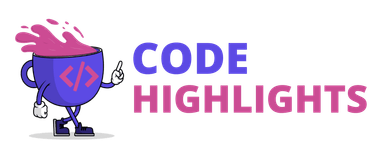
Copyright © Code Highlights 2025.