Understanding JavaScript Let vs Var: Which to Use?

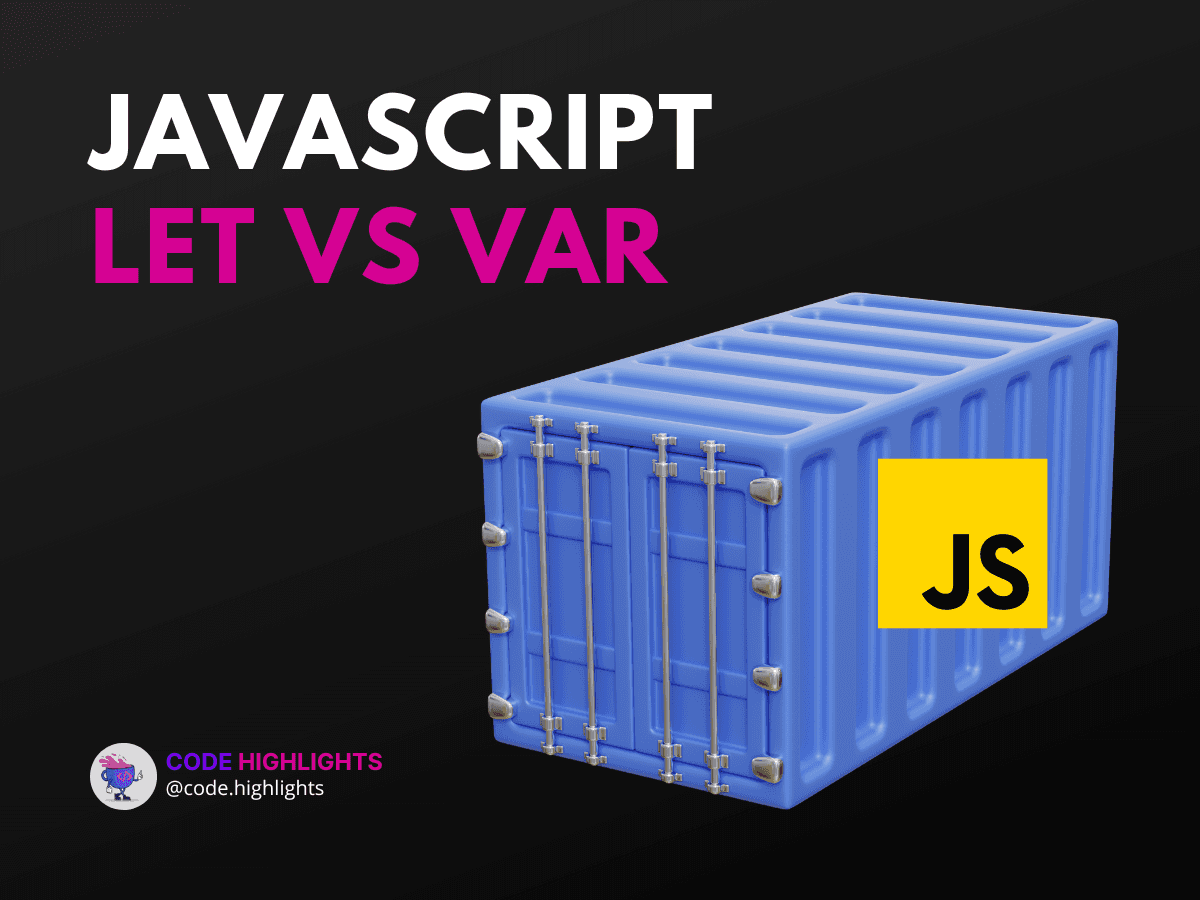
- Should I Use let or var in JavaScript?
- What is the Difference Between let, const, and var in JavaScript?
- Why is var Not Used in JavaScript Anymore?
- What Does let Do in JavaScript?
When diving into the world of JavaScript, one of the most common dilemmas faced by developers is choosing between let
and var
for variable declaration. This tutorial will shed light on this topic, helping you understand when and why to use each keyword.
Let's start with a simple code snippet to get our feet wet:
Here we see var
and let
in action, but what sets them apart? As we unravel the mysteries of these keywords, you'll learn how to write more robust and maintainable JavaScript code.
Should I Use let
or var
in JavaScript?
To answer this, we must first delve into the history of JavaScript. Initially, var
was the only option for declaring variables. However, var
has its quirks, especially around scoping rules. Enter ES6 (ECMAScript 2015), which introduced let
and const
, providing developers with more control over variable scope.
let
allows you to declare variables that are limited in scope to the block, statement, or expression where they are used, compared to var
, which defines a variable globally or locally to an entire function regardless of block scope.
For a deeper understanding of JavaScript variables, consider enrolling in JavaScript courses or starting with the basics in an introduction to web development course.
What is the Difference Between let
, const
, and var
in JavaScript?
The primary differences lie in scope, hoisting, and reassignment. var
is function-scoped, while let
and const
are block-scoped. var
declarations are hoisted to the top of their scope and initialized with undefined
, meaning they can be referenced before they're declared. let
and const
, however, are not initialized until their definition is evaluated.
Additionally, var
allows redeclaration within its scope, whereas let
does not. const
is similar to let
but requires immediate assignment and cannot be reassigned later.
Why is var
Not Used in JavaScript Anymore?
While var
is still part of the language, it's often avoided in modern JavaScript due to its confusing scope behavior. let
provides a clearer, more predictable way to declare variables and has largely supplanted var
in contemporary codebases. For instance, let
is block-scoped, making it a better choice for loops and conditionals where you want the variable to exist only within a certain context.
For those who wish to learn more about the evolution of JavaScript and best practices, there are resources available like the HTML fundamentals course and the CSS introduction course that provide foundational knowledge beneficial for understanding modern JavaScript usage.
What Does let
Do in JavaScript?
let
allows you to declare a variable with block-level scope. Unlike var
, which can sometimes lead to unexpected results due to its function-level scope, let
makes it easier to manage variables in complex applications. Here's an example to illustrate:
1if (true) {
2 let blockScoped = "I'm only visible here!";
3 console.log(blockScoped); // Output: I'm only visible here!
4}
5console.log(blockScoped); // ReferenceError: blockScoped is not defined
In the above example, blockScoped
is not accessible outside the if
block, demonstrating the block-level scope of let
.
To solidify your understanding of let
, var
, and even const
, it's beneficial to read through reputable sources such as Mozilla Developer Network (MDN), W3Schools, and ECMA International for the official ECMAScript documentation.
By now, you should have a clearer picture of the javascript let vs var
debate. Remember, let
is generally preferred for its block-level scoping, reducing the chance of bugs related to variable scope. It's a modern feature of JavaScript that aligns well with the principle of least privilege, granting variable access only where necessary.
Keep practicing, keep learning, and soon the nuances of JavaScript variable declaration will become second nature to you. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
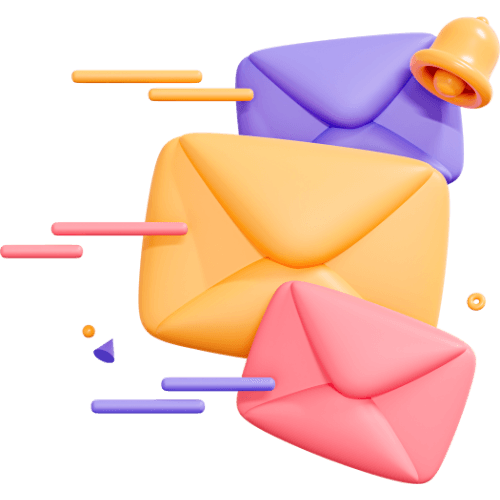
Related articles
9 Articles
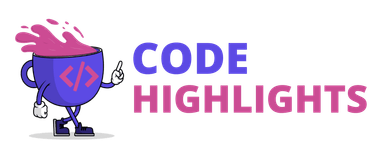
Copyright © Code Highlights 2025.