Avoid Boring Designs With JavaScript Make Button Techniques

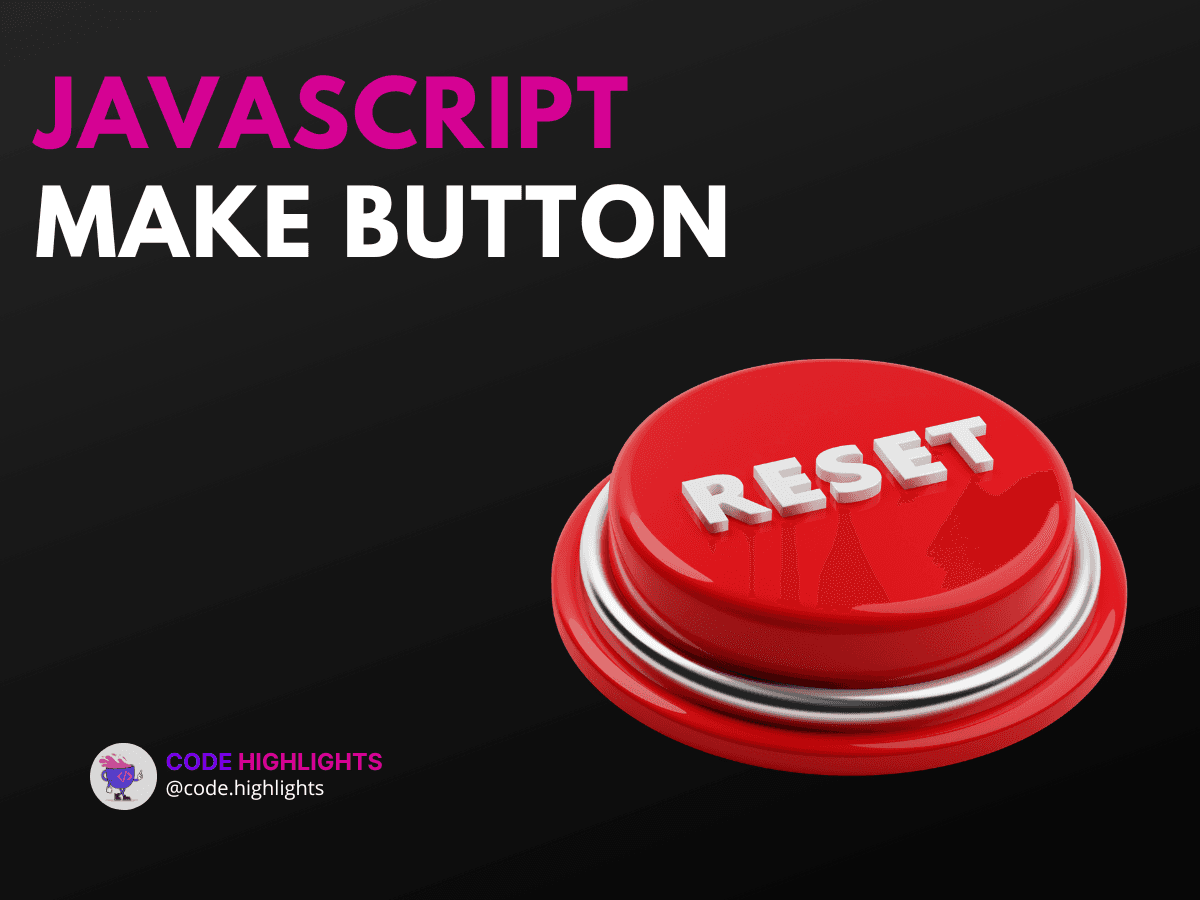
- Introduction to JavaScript Button Creation
- Understanding the Syntax
- Example: Creating a Styled Button
- Dynamic Button Creation
- How to Create a Button in JavaScript Dynamically
- How to Trigger a Button in JavaScript
- Compatibility with Major Web Browsers
- Summary
Creating buttons with JavaScript can add flair to your web projects. Buttons are essential for user interaction, making them a vital part of web design. In this tutorial, we will explore various techniques to create and manipulate buttons using JavaScript. This way, you can avoid dull designs and make your website more engaging!
Introduction to JavaScript Button Creation
To kick things off, let's look at a simple example of how to create a button using JavaScript. Here’s a quick code snippet:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>JavaScript Button Example</title>
6</head>
7<body>
8 <script>
9 const button = document.createElement('button');
10 button.innerText = 'Click Me!';
11 document.body.appendChild(button);
12 </script>
13</body>
14</html>
This code creates a button that says "Click Me!" and adds it to the webpage.
Understanding the Syntax
The document.createElement()
method is crucial for creating elements in JavaScript. Here’s a breakdown of the syntax:
- Parameters:
- The name of the element you want to create (e.g.,
'button'
).
- The name of the element you want to create (e.g.,
- Return Value:
- Returns the newly created element.
You can also set attributes and styles for your button using properties like innerText
, style
, and setAttribute()
.
Example: Creating a Styled Button
Here’s how you can create a button with some style:
1const styledButton = document.createElement('button');
2styledButton.innerText = 'Styled Button';
3styledButton.style.backgroundColor = 'blue';
4styledButton.style.color = 'white';
5document.body.appendChild(styledButton);
This example shows how to change the background color and text color of the button.
Dynamic Button Creation
How to Create a Button in JavaScript Dynamically
You can create buttons dynamically based on user actions. For instance, let’s create a button each time another button is clicked:
1const createButton = () => {
2 const newButton = document.createElement('button');
3 newButton.innerText = 'New Button';
4 document.body.appendChild(newButton);
5};
6
7const triggerButton = document.createElement('button');
8triggerButton.innerText = 'Add a Button';
9triggerButton.onclick = createButton;
10document.body.appendChild(triggerButton);
In this example, clicking the "Add a Button" will generate a new button on the page.
How to Trigger a Button in JavaScript
You can also trigger actions when a button is clicked. Here’s an example of how to display an alert when a button is pressed:
1const alertButton = document.createElement('button');
2alertButton.innerText = 'Show Alert';
3alertButton.onclick = () => alert('Button Clicked!');
4document.body.appendChild(alertButton);
This button will show an alert message when clicked.
Compatibility with Major Web Browsers
The methods described above are widely supported across all major browsers, including Chrome, Firefox, Safari, and Edge. This ensures that your buttons will work seamlessly for most users.
Summary
In this tutorial, we explored various techniques for creating buttons with JavaScript. From basic button creation to dynamic generation and event triggering, these skills can significantly enhance your web design. Remember, engaging designs keep users interested!
If you’re eager to learn more about web development, check out our courses on HTML Fundamentals and CSS Introduction. You can also dive deeper into JavaScript to further improve your skills.
By mastering these JavaScript button techniques, you can take your web projects from boring to exciting!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
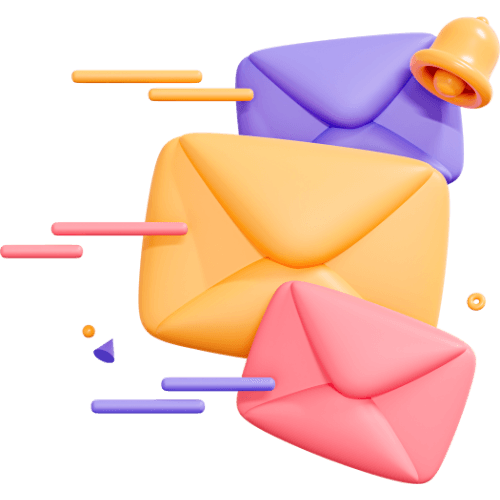
Related articles
9 Articles
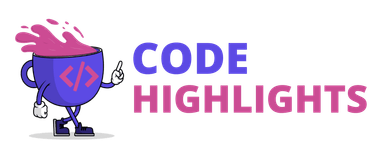
Copyright © Code Highlights 2025.