JavaScript Merge 2 Objects: 10 Common Mistakes to Avoid

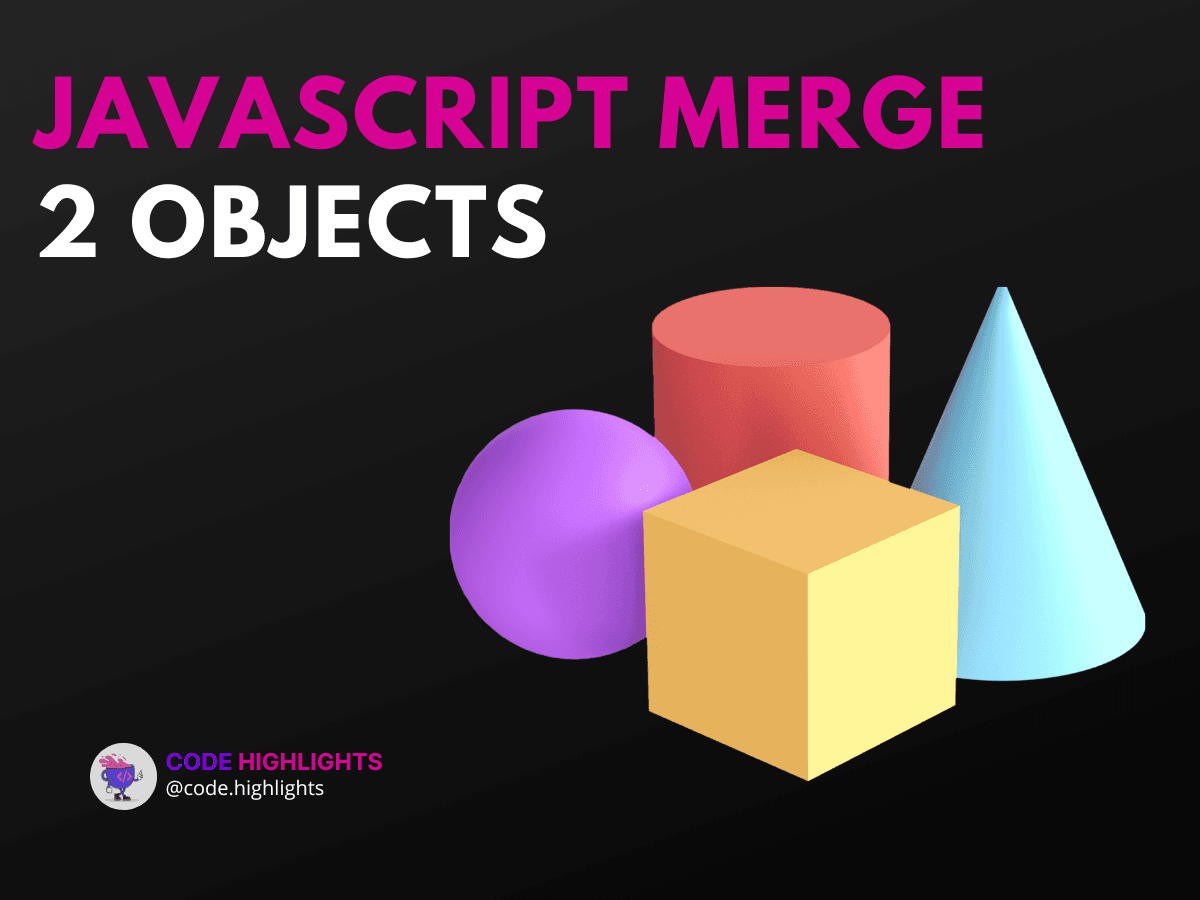
- Quick Example to Get Started
- Understanding the Syntax
- Parameters
- Return Value
- Common Mistakes to Avoid
- 1. Ignoring Property Overwrites
- 2. Forgetting Nested Objects
- 3. Using Non-Object Types
- 4. Not Checking for Undefined Properties
- 5. Merging Arrays Incorrectly
- 6. Forgetting to Clone Objects
- 7. Not Handling Prototype Properties
- 8. Overlooking Performance Implications
- 9. Skipping Browser Compatibility Checks
- 10. Failing to Document Merged Objects
- Conclusion
Merging objects in JavaScript is a common task that can help you combine data from different sources into a single object. This process can be useful for many applications, such as when you want to update user profiles or consolidate configuration settings. However, it's easy to make mistakes along the way. In this tutorial, we will explore the javascript merge 2 objects
technique and highlight ten common pitfalls to avoid.
Quick Example to Get Started
Before diving into the details, let’s look at a simple example of merging two objects using the spread operator.
1const obj1 = { name: "Alice", age: 25 };
2const obj2 = { city: "Wonderland", age: 30 };
3
4const mergedObj = { ...obj1, ...obj2 };
5console.log(mergedObj); // Output: { name: 'Alice', age: 30, city: 'Wonderland' }
In this example, the age
property from obj2
overrides the one in obj1
.
Understanding the Syntax
When merging objects, you can use several methods, including the spread operator, Object.assign()
, and more. Here’s a breakdown of the syntax:
- Spread Operator:
{ ...object1, ...object2 }
- Object.assign():
Object.assign(target, source1, source2)
Parameters
- target: The object to which properties will be copied.
- source: The objects from which properties are taken.
Return Value
The returned value is the target object with the merged properties.
Common Mistakes to Avoid
1. Ignoring Property Overwrites
When merging, properties in the second object will overwrite those in the first. Be aware of this behavior!
1const a = { x: 1 };
2const b = { x: 2 };
3
4const c = { ...a, ...b };
5console.log(c); // Output: { x: 2 }
2. Forgetting Nested Objects
Merging does not deeply merge nested objects. For example:
1const objA = { info: { name: "Bob" } };
2const objB = { info: { age: 30 } };
3
4const merged = { ...objA, ...objB };
5console.log(merged); // Output: { info: { age: 30 } }
3. Using Non-Object Types
Trying to merge non-object types can lead to errors. Always ensure you're working with objects.
1const objC = null;
2const objD = { y: 3 };
3
4// This will throw an error
5const result = { ...objC, ...objD };
4. Not Checking for Undefined Properties
Undefined properties can lead to unexpected results. Always check if properties exist before merging.
5. Merging Arrays Incorrectly
Merging arrays directly can lead to unexpected concatenation. Instead, use functions like concat()
.
1const array1 = [1, 2];
2const array2 = [3, 4];
3
4const mergedArray = [...array1, ...array2]; // Output: [1, 2, 3, 4]
6. Forgetting to Clone Objects
If you modify the merged object, it can affect the original objects. Always create a clone if needed.
7. Not Handling Prototype Properties
Using Object.assign()
can copy properties from the prototype chain. Be cautious with inherited properties.
8. Overlooking Performance Implications
Merging large objects can impact performance. Consider using libraries like Lodash for complex merges.
9. Skipping Browser Compatibility Checks
Ensure that the methods you use are compatible with the browsers you want to support. The spread operator is not supported in Internet Explorer.
10. Failing to Document Merged Objects
Always document your merged objects clearly to avoid confusion later on.
Conclusion
Merging objects in JavaScript is a powerful tool, but it comes with its challenges. By avoiding these common mistakes, you can ensure smoother coding experiences. Remember, whether you're updating user profiles or consolidating data, knowing how to correctly javascript merge 2 objects
is crucial.
For more in-depth learning, consider checking out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. If you're interested in a broader context, our Introduction to Web Development course might be helpful.
For further reading, you can explore resources from MDN Web Docs, JavaScript.info, and W3Schools.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
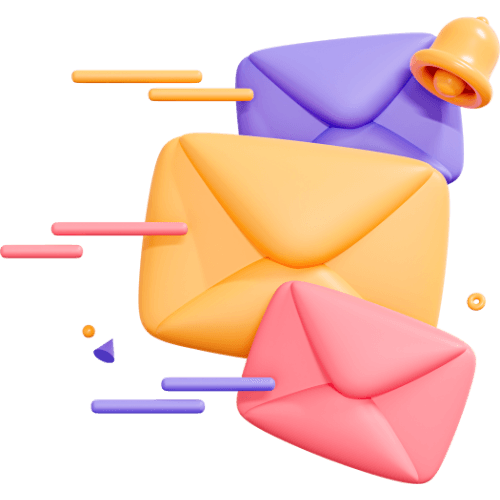
Related articles
9 Articles
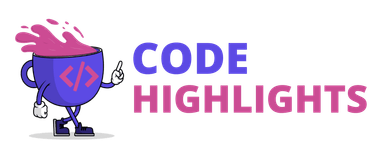
Copyright © Code Highlights 2024.