Merge JavaScript Arrays: Top 3 Efficient Methods

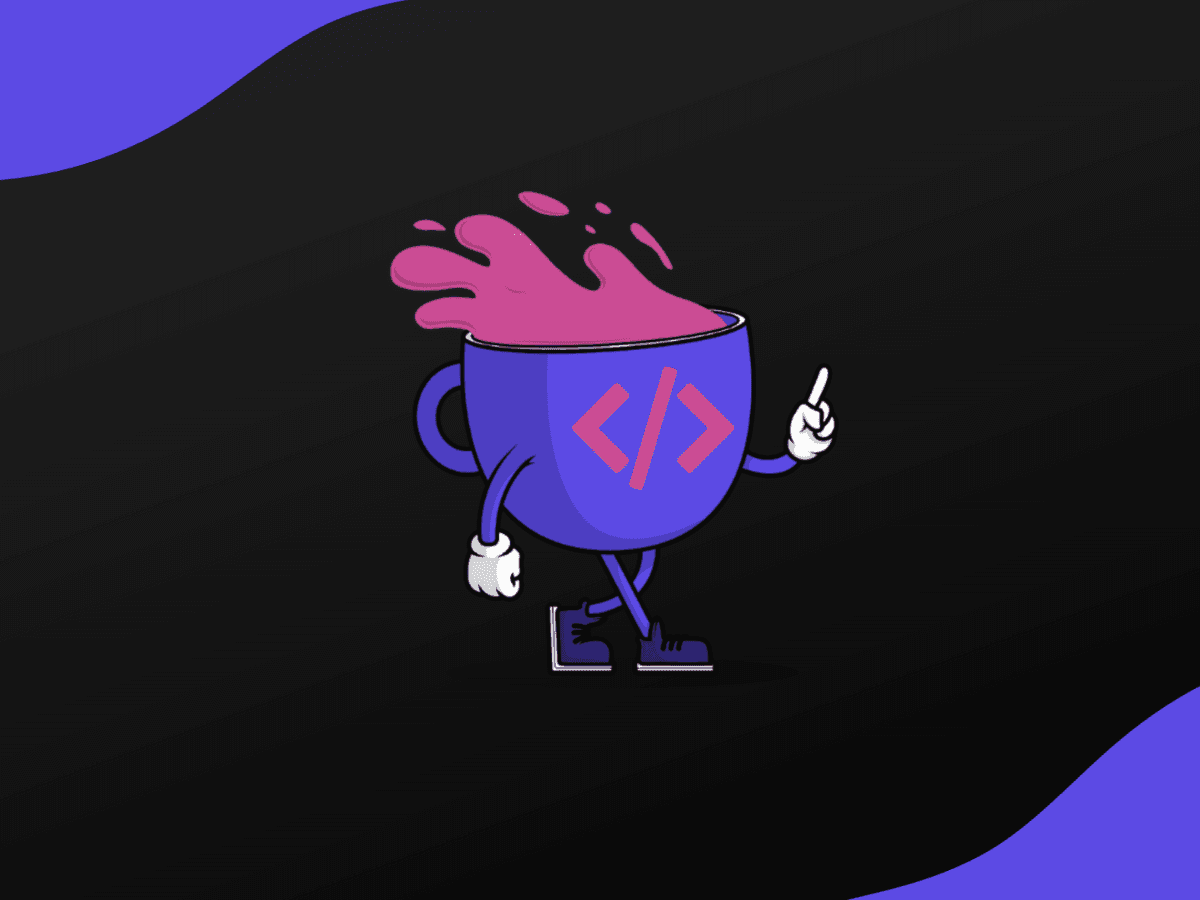
- concat()
- Object.assign()
- Spread Operator
- Browser Compatibility
In JavaScript, there's a common task that involves merging arrays and objects. This tutorial will explore various ways to achieve this with vanilla JavaScript, focusing on the merge array of objects
concept.
concat()
The concat()
method is used to merge two or more arrays. This method does not change the existing arrays but returns a new array.
1var arr1 = ["Cecil", "David"];
2var arr2 = ["Barbara", "Daniel"];
3var arr3 = arr1.concat(arr2);
4console.log(arr3); // ["Cecil", "David", "Barbara", "Daniel"]
This method can also be used to merge arrays of objects.
1var obj1 = [{ name: "Cecil", age: 25 }];
2var obj2 = [{ name: "David", age: 30 }];
3var obj3 = obj1.concat(obj2);
4console.log(obj3); // [{ name: "Cecil", age: 25 }, { name: "David", age: 30 }]
Object.assign()
The Object.assign()
method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It returns the target object.
1var obj1 = { name: "Cecil", age: 25 };
2var obj2 = { name: "David", age: 30 };
3var obj3 = Object.assign(obj1, obj2);
4console.log(obj3); // { name: "David", age: 30 }
Note that Object.assign()
does not deep clone. If the source value is a reference to an object, it only copies the reference value. For a deep clone, you need to use other methods, like the Spread operator or Lodash's _.cloneDeep()
method.
Spread Operator
The spread operator allows an iterable to expand in places where zero or more arguments or elements are expected. In the case of objects, it copies enumerable own properties from a provided object onto a new object.
1var obj1 = { name: "Cecil", age: 25 };
2var obj2 = { ...obj1, name: "David" };
3console.log(obj2); // { name: "David", age: 25 }
Browser Compatibility
All three methods work in all modern browsers, and IE6 and up. If you found this post useful, you might also like my Learn JavaScript, HTML Fundamentals Course, Learn CSS Introduction, and Introduction to Web Development courses, which feature a ton of useful methods like these.
For more on JavaScript arrays, check out MDN's guide on Array, and for more on Objects, see MDN's guide on Object.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
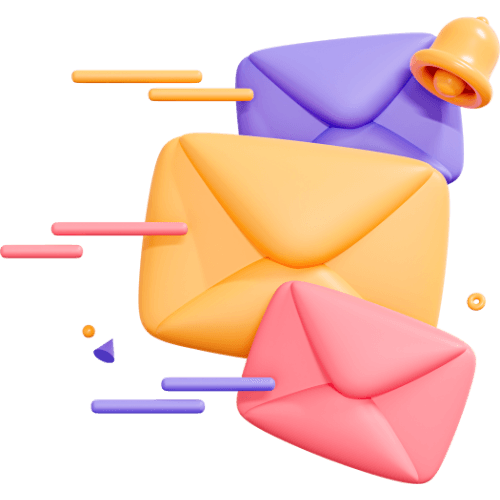
Related articles
9 Articles
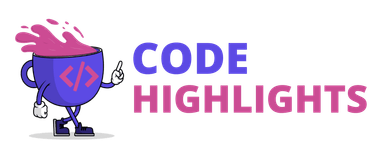
Copyright © Code Highlights 2025.