3 Quick Tips to Enhance JavaScript Modal Show in Minutes

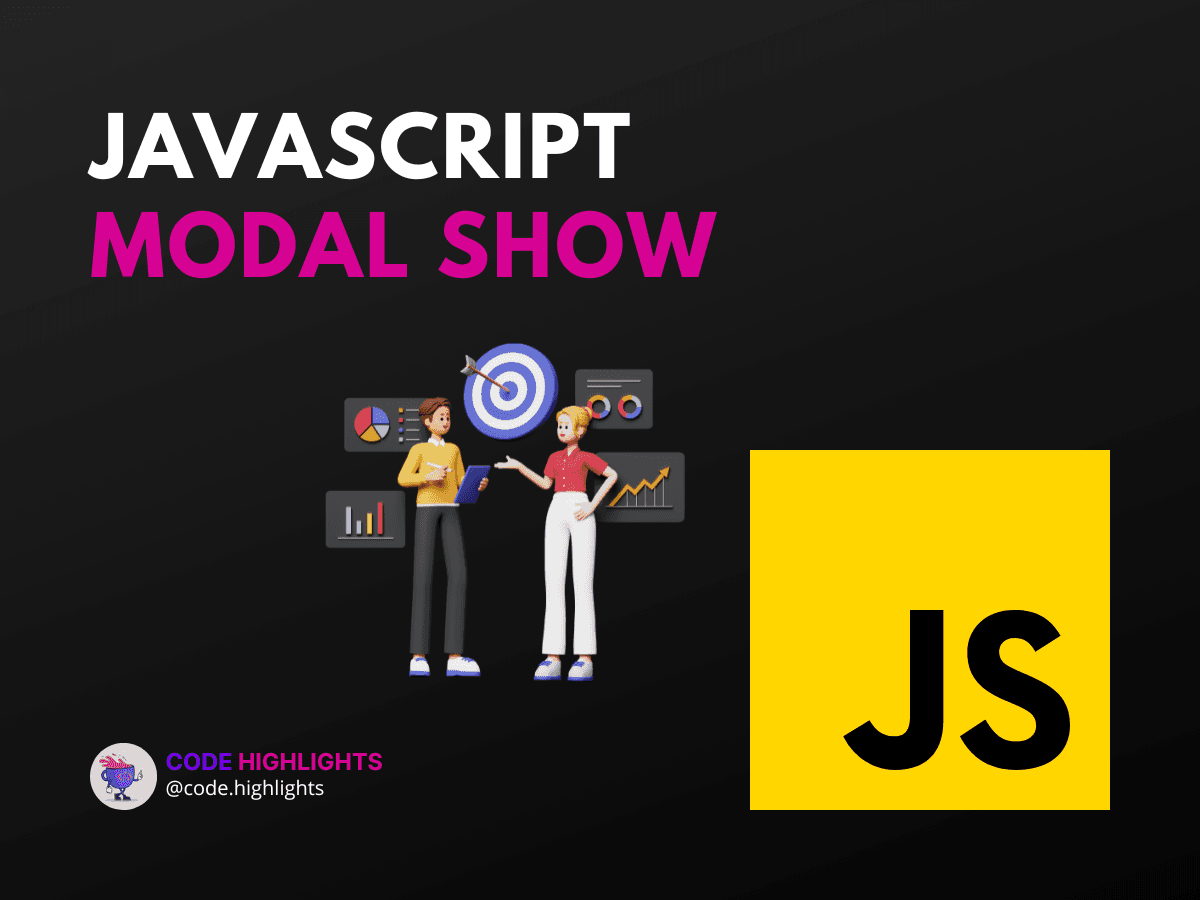
- Tip 1: Animate Your Modal Entrance
- Tip 2: Use JavaScript to Dynamically Display Data
- Tip 3: Enhance Accessibility with ARIA Attributes
When building interactive websites, you may often need to display additional information without navigating away from the current page. This is where modals come into play. A modal is a dialog box/popup window that is displayed on top of the current page. In this tutorial, we'll explore some quick tips to enhance your javascript modal show
technique, ensuring you can present information in a sleek, user-friendly manner.
Here's a sneak peek at what a basic modal might look like in JavaScript:
1function showModal() {
2 var modal = document.getElementById('myModal');
3 modal.style.display = "block";
4}
This simple snippet activates a modal by changing its display style. But let's not stop here; we'll dive into how to make this experience even better.
Tip 1: Animate Your Modal Entrance
To capture your user's attention smoothly, consider adding animations to your modal's appearance. CSS transitions can do wonders here. If you're starting with HTML fundamentals, you'll find that combining it with CSS and JavaScript can produce impressive results.
1.modal {
2 display: none;
3 transition: opacity 0.5s ease;
4}
5.modal.show {
6 display: block;
7 opacity: 1;
8}
Now, modify your JavaScript function to add the show
class:
1function showModal() {
2 var modal = document.getElementById('myModal');
3 modal.classList.add('show');
4}
With the transition property, your modal now fades in gently instead of popping up abruptly.
Tip 2: Use JavaScript to Dynamically Display Data
Often, you'll need to display dynamic data within your modals. How do you go about this? It's simple with JavaScript. Let's say you have user information that you want to show in a modal popup. Here's how you could do it:
1function showModalWithData(userId) {
2 var modal = document.getElementById('myModal');
3 var userData = getUserData(userId); // Assume this function fetches user data
4 var modalContent = modal.querySelector('.modal-content');
5 modalContent.innerHTML = `<h2>User Profile</h2><p>Name: ${userData.name}</p>`;
6 modal.classList.add('show');
7}
This snippet fetches the user data and dynamically inserts it into the modal's content area when you call showModalWithData
.
Tip 3: Enhance Accessibility with ARIA Attributes
Accessibility is key in web development. When showing a dialog in JavaScript, using ARIA (Accessible Rich Internet Applications) attributes can make your modals more accessible to users with disabilities. Dive into an introduction to web development to understand the importance of building inclusive websites.
1function showModal() {
2 var modal = document.getElementById('myModal');
3 modal.setAttribute('aria-hidden', 'false');
4 modal.classList.add('show');
5}
6
7function hideModal() {
8 var modal = document.getElementById('myModal');
9 modal.setAttribute('aria-hidden', 'true');
10 modal.classList.remove('show');
11}
Setting aria-hidden
to false
when the modal is shown and true
when it's hidden helps screen readers understand the state of the modal.
By implementing these tips, your javascript modal show
functionality will not only look better but also be more dynamic and accessible. Remember, the key to mastering JavaScript is practice and continuous learning. Explore courses like Learn JavaScript or Learn CSS to deepen your understanding.
For further reading, check out reputable sources like Mozilla Developer Network (MDN) or W3Schools for comprehensive guides and best practices. They offer a wealth of information that can help you refine your skills in creating modals and much more.
In conclusion, enhancing your modals with animation, dynamic data, and accessibility features can greatly improve the user experience on your website. Keep experimenting with different styles, animations, and techniques to find what works best for your projects. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
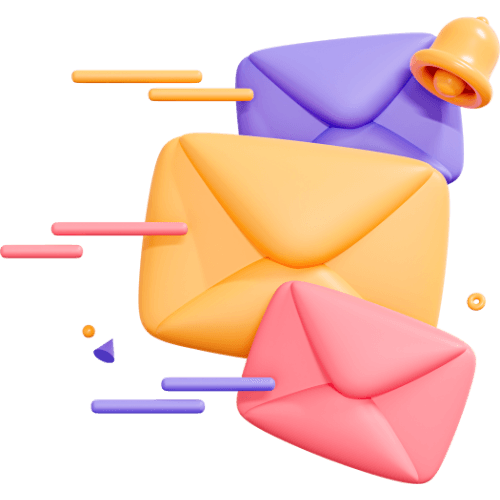
Related articles
9 Articles
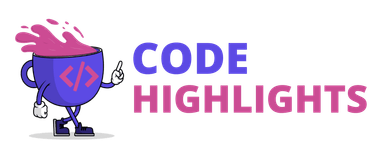
Copyright © Code Highlights 2024.