JavaScript Multiline Strings: How Can You Use Them Effectively?

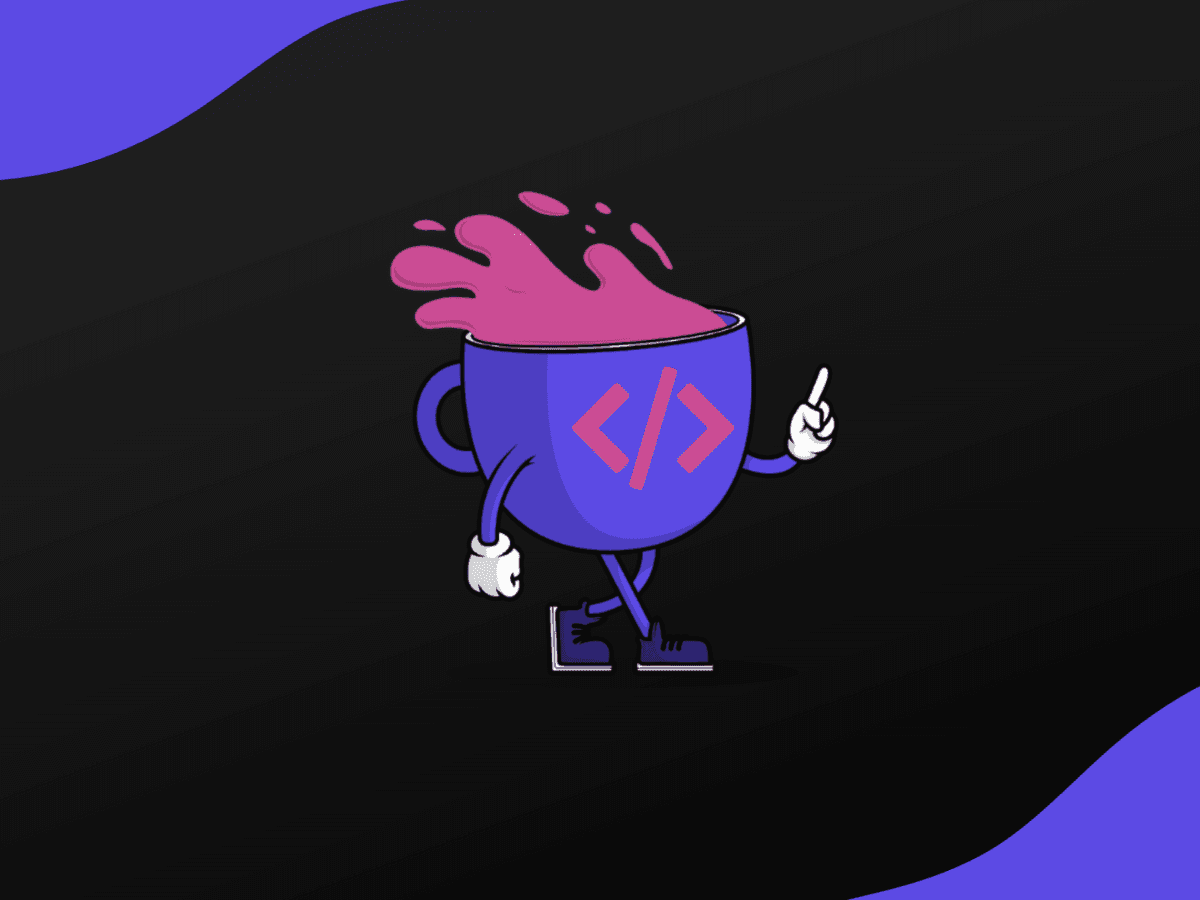
- Template Literals
- Escape Sequences
- Browser Compatibility
In JavaScript, strings are typically defined within single ('
) or double ("
) quotes. However, these methods don't allow for easy creation of multiline strings. This tutorial will show you how to create multiline strings using backticks (```) in JavaScript.
1var singleLine = 'This is a single line string';
2console.log(singleLine);
3
4var multiLine = `This is a
5multiline
6string`;
7console.log(multiLine);
The output of the above code will be:
1This is a single line string
2This is a
3multiline
4string
Template Literals
Backticks are used in JavaScript to define template literals, a type of string that can span multiple lines and include embedded expressions.
1var name = 'John';
2var greeting = `Hello, ${name}!
3Welcome to our website.`;
4console.log(greeting);
This will output:
1Hello, John!
2Welcome to our website.
Escape Sequences
Alternatively, you can create multiline strings in JavaScript using escape sequences. The newline character (\n
) is used to insert a new line.
This will output:
1This is a
2multiline
3string
Browser Compatibility
All modern browsers support template literals and escape sequences for creating multiline strings in JavaScript.
For a more in-depth understanding of JavaScript strings, check out our Learn JavaScript
course.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
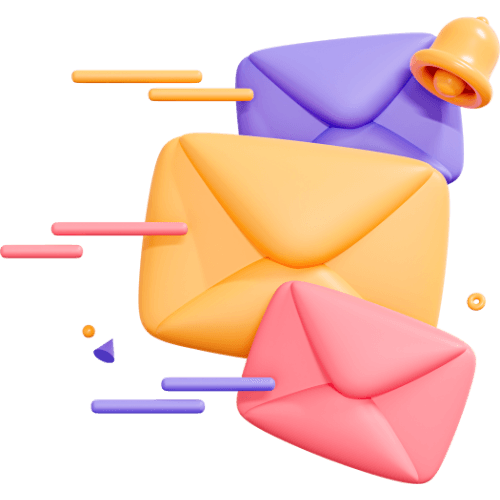
Related articles
9 Articles
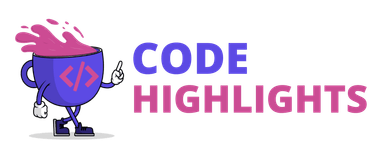
Copyright © Code Highlights 2024.